Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial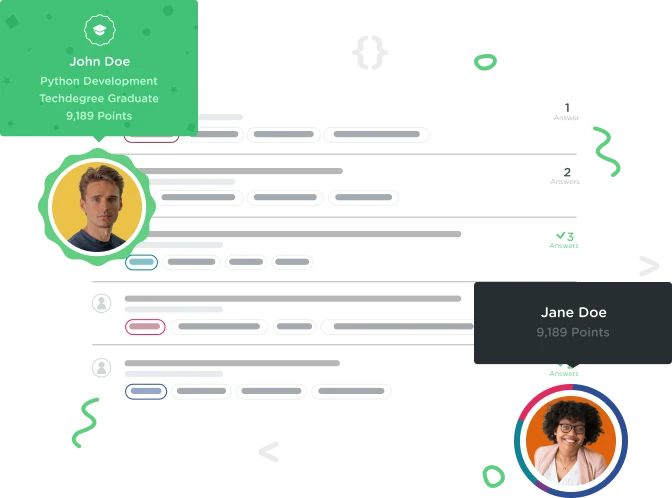
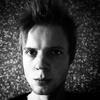
Audrius Lebednykas
1,570 PointsNeed to make a simple question answer form in Swift. Don't know where to start.
Hello there, I need to make a very simple Swift app, which gives a person 5-7 questions , and he has to answer them. I've almost completed the Swift basics course, but no such topic as user input was covered. Where do I start? Design is not at all important at this point, although it has to be single page app!
7 Answers
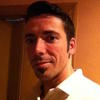
Daniel Sattler
4,867 Points- Set up your View in the Storyboard
- Add all labels and Textfields needed.
- Add IBOutlets for those Textfields
- Add one Button and the IBAction for this button
- within the function of the IBAction take the String from the Textfield and save it permanent.
- Then set the Textfields Text to this permanent values, if they exist.
In total it would look something like this, for example:
IBOutlets for the Textfields
@IBOutlet var firstNameTextField: UITextField!
@IBOutlet var surnameTextField: UITextField!
@IBOutlet var ageTextField: UITextField!
@IBOutlet var emailTextField: UITextField!
// and so on
Then the Save Button that stores everything permanently
@IBAction func submitButtonPressed() {
NSUserDefaults.standardUserDefaults().setObject(firstNameTextField.text, forKey: "firstName")
NSUserDefaults.standardUserDefaults().setObject(surnameTextField.text, forKey: "surname")
NSUserDefaults.standardUserDefaults().setObject(ageTextField.text, forKey: "age")
NSUserDefaults.standardUserDefaults().setObject(emailTextField.text, forKey: "email")
NSUserDefaults.standardUserDefaults().synchronize()
}
then in the viewDidLoad Method check if there is something stored and if not, set some placeholder texts
func viewDidLoad() {
super.viewDidLoad()
if NSUserDefaults.standardUserDefaults().objectForKey("firstName") != nil {
firstNameTextField.text = NSUserDefaults.standardUserDefaults().objectForKey("firstName") as String
} else {
firstNameTextField.placeholder = "First name"
}
if NSUserDefaults.standardUserDefaults().objectForKey("firstName") != nil {
surnameTextField.text = NSUserDefaults.standardUserDefaults().objectForKey("surname") as String
} else {
surnameTextField.placeholder = "Surname"
}
if NSUserDefaults.standardUserDefaults().objectForKey("age") != nil {
ageTextField.text = NSUserDefaults.standardUserDefaults().objectForKey("age") as String
} else {
ageTextField.placeholder = "Age"
}
if NSUserDefaults.standardUserDefaults().objectForKey("email") != nil {
emailTextField.text = NSUserDefaults.standardUserDefaults().objectForKey("email") as String
} else {
emailTextField.placeholder = "E-Mail"
}
}
Next time you load the App, everything will show up in the text fields as you saved it before.
That´s just a quick example of how it COULD be done. Of course as always, there are several ways to get what you want.
Just have a look around ;-)
I hope this helps
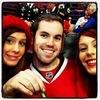
Patrick Cooney
12,216 PointsThe easiest way to store such basic information would be to use a plist. Take a look at the NSFileManager documentation for more on how to use it. The plist will get stored on the user's phone and you can pull the information from it later.
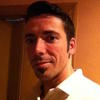
Daniel Sattler
4,867 Pointsoh... and you could of course set NSUserDefaults to stringForKey instead of Object ;-)
My mistake
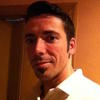
Daniel Sattler
4,867 PointsWhat are you going to do with those answers? Just save them in the App or do you want to send them somewhere? Do you want text fields as answers or multiple choice?
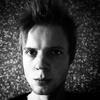
Audrius Lebednykas
1,570 PointsIn the future I am going to send them, but now, I want to save them in the app. Questions are simple like what's you name and etc. I want to use label as a question and text field as an answer, but how do I store those answers in the app?
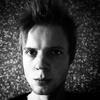
Audrius Lebednykas
1,570 PointsThank you very much!
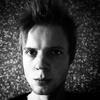
Audrius Lebednykas
1,570 PointsThanks again, this is exactly what I needed :)