Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial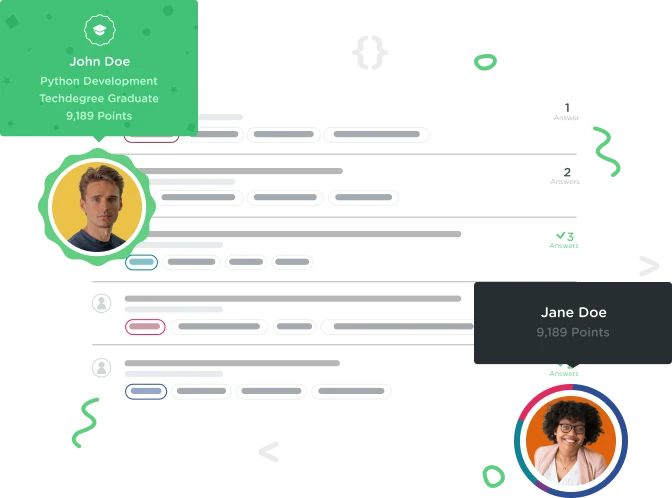

Jared Welch
5,616 PointsNeeding Help
is this not the right way to write my code?
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
if (3 == 0) && (5 == 0) {
print("FizzBuzz")
} else if (3 == 0) {
print("Fizz")
} else if (5 == 0) {
print("Buzz")
}
// End code
return "\(n)"
}
2 Answers
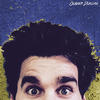
Oliver Duncan
16,642 PointsThere are a few things wrong with your code. Let's take a look:
func fizzBuzz(n: Int) -> String {
// This if statement will never be true, because 3 will never equal 0, and neither will 5
if (3 == 0) && (5 == 0) {
print("FizzBuzz")
// 3 will never equal 0
} else if (3 == 0) {
print("Fizz")
// 5 will also never equal 0
} else if (5 == 0) {
print("Buzz")
}
return "\(n)"
}
You need to use the modulo (or remainder) operator, %, to test whether the integer you pass into the function is divisble by 3 and/or 5, and return the corresponding string. The correct implementation would therefore be
func fizzBuzz(n: Int) -> String {
// Check if the remainder of (n / 3) and (n / 5) is equal to 0 and therefore divisible by both 3 and 5
if (n % 3 == 0) && (n % 5 == 0) {
return "FizzBuzz")
// If n is not divisible by both 3 and 5, check if it's divisible by 3
} else if (n % 3 == 0) {
return "Fizz"
// If n isn't divisible by 3, check if it's divisible by 5
} else if (n % 5 == 0) {
return "Buzz"
}
// If n isn't divisible by 3 or 5, we just return the number as a string
return "\(n)"
}

Jared Welch
5,616 PointsAlright i see it now thank you very much!