Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial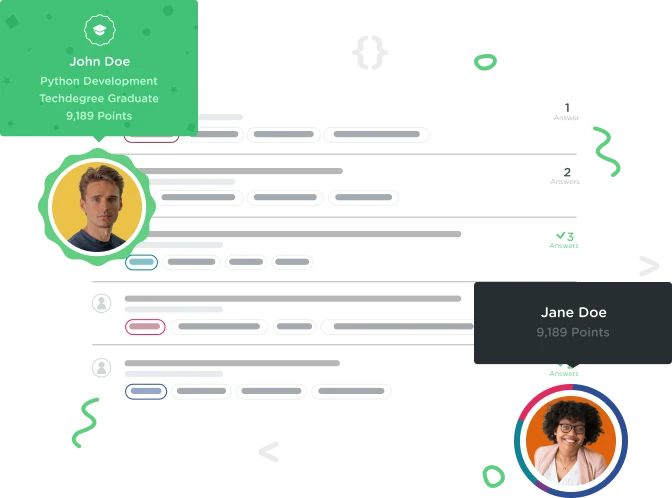
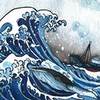
Like Water
7,873 PointsNeeding to Click Twice to Add Friends to List?
Hello all,
I'm currently working on the Self-Destructing App and everything seems to be working perfectly. However, there is one nuance in my app that's really bugging me.
Supposedly, when you click on a friend to either add or remove them from your friends list, after clicking their name, you should immediately be able to see a check mark appear or disappear from that particular row.
However, on my implementation of this app, you have to click the name once to select it, and then click the name again to either remove or add them.
Consequently, clicking on a particular row and then clicking away to another name also seems to do the trick.
Below is the code in my EditFriendsController.m file:
#import "EditFriendsViewController.h"
@interface EditFriendsViewController ()
@end
@implementation EditFriendsViewController
- (void)viewDidLoad
{
[super viewDidLoad];
PFQuery *query = [PFUser query];
[query orderByAscending:@"username"];
[query findObjectsInBackgroundWithBlock:^(NSArray *objects, NSError *error) {
if (error) {
NSLog(@"Error %@ %@", error, [error userInfo]);
} else {
self.allUsers = objects;
[self.tableView reloadData];
}
}];
self.currentUser = [PFUser currentUser];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
static NSString *cellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:cellIdentifier forIndexPath:indexPath];
PFUser *user = [self.allUsers objectAtIndex:indexPath.row];
cell.textLabel.text = user.username;
if ([self isFriend:user]) {
cell.accessoryType = UITableViewCellAccessoryCheckmark;
} else {
cell.accessoryType = UITableViewCellAccessoryNone;
}
return cell;
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
// Return the number of sections.
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
#warning Incomplete method implementation.
// Return the number of rows in the section.
return [self.allUsers count];
}
- (void)tableView:(UITableView *)tableView didDeselectRowAtIndexPath:(NSIndexPath *)indexPath {
[self.tableView deselectRowAtIndexPath:indexPath animated:NO];
UITableViewCell *cell = [tableView cellForRowAtIndexPath:indexPath];
PFUser *user = [self.allUsers objectAtIndex:indexPath.row];
PFRelation *friendsRelation = [self.currentUser relationForKey:@"friendsRelation"];
if ([self isFriend:user]) {
cell.accessoryType = UITableViewCellAccessoryNone;
for (PFUser *friend in self.friends) {
if ([friend.objectId isEqualToString:user.objectId]) {
[self.friends removeObject:friend];
break;
}
}
[friendsRelation removeObject:user];
} else {
cell.accessoryType = UITableViewCellAccessoryCheckmark;
[self.friends addObject:user];
[friendsRelation addObject:user];
}
[self.currentUser saveInBackgroundWithBlock:^(BOOL succeeded, NSError *error) {
if (error) {
NSLog(@"Error %@ %@", error, [error userInfo]);
}
}];
}
#pragma mark - Helper Methods
- (BOOL)isFriend:(PFUser *)user {
for (PFUser *friend in self.friends) {
if ([friend.objectId isEqualToString:user.objectId]) {
return YES;
}
}
return NO;
}
@end
Any ideas? Is my if statement for adding and removing friends missing a step, or is it the fact that I'm using Xcode 6, while the video seems to be using Xcode 4 an issue?
Thanks in advance.
5 Answers

Ezequiel Amaya
3,094 PointsHi,
I found several differences with the original code :
(1) in tableView:(UITableView *)tableView didDeselectRowAtIndexPath: ...
replace relationForKey (this creates a compilation error) for relationforKey
(2) in (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath: ...
replace static NSString *cellIdentifier = @"Cell"; for static NSString *CellIdentifier = @"Cell"; replace dequeueReusableCellWithIdentifier:cellIdentifier for dequeueReusableCellWithIdentifier:CellIdentifier
!!!!!!!!
it took me several hours and I almost replaced line by line the original code with your code until I found this ...
you wrote .... didDeselectRowAtIndexPath (and this code compiles with no errors and apparently works!)
and the correct one is .... didSelectRowAtIndexPath
Please try this correction and let us know if this solves your problem.

Ezequiel Amaya
3,094 PointsGlad to help! Because of the name, the method belonged to the EditFriendsViewController class. There was no error from the UITableViewController class since didSelectRowAtIndexPath is an optional method.

M T
11,934 PointsThank you for this. I had a similar problem that needed the same solution. Since we are now using a different version of Xcode than the instructor, our EditFriendsViewController.m populates with different (fewer) default methods. In hand-typing them to match the instructor's information, it's possible to mistake
didSelectRowAtIndexPath
for
didDeselectRowAtIndexPath
when auto-populating code.
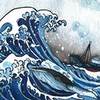
Like Water
7,873 PointsDude!
First, props for the several hours worth of work! I bow to your tedious excellence!!!!!!
When reading your response, I IMMEDIATELY focused on the "Deselect" error that I didn't seem to notice.
I just changed that to "Select" and it worked like a charm!
Going to fix some of the other errors that while didn't affect compilation, may affect me in some other way down the road.
Again... I tip my hat!!!
+++++++++++++++++ several minutes later.....
Hey Ezequiel,
I distinctly remember the "relationforKey" letter "f" being typed as lowercased in the video, so I went back to find out exactly why I typed an uppercase "F". It turns out because I'm using the Xcode6 beta3, the message I received after typing "relationforKey" was the following:
'relationforKey:' is deprecated: Use - relationForKey: instead.
Regarding the capitalization of "cell", I changed the @"Cell" strings to capital because the cell identifier was typed as "Cell" in the instruction video. However, I decided to leave the "cell" variable as such, since the video instructed this way.
Thanks a million!! Again!! You really made my week... I will never forget it, bro...
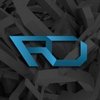
Raul Armendariz
6,970 PointsIt was also bugging me lol, made the same mistake as everyone else. To my brain aparently both methods look the same.