Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial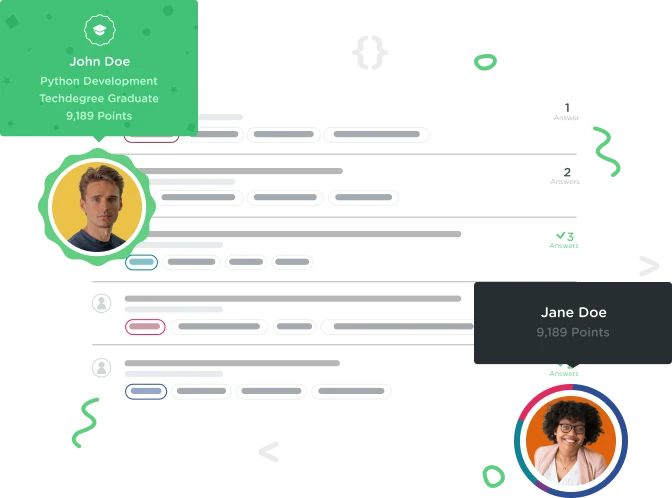

Tony Brackins
28,766 Pointsnew Treet[0];
in this level we are creating a Serialization and De-Serialization. On the Deserialization we create an array like so:
public static Treet[] load() {
Treet[] treets = new Treet[0];
} }
My question is, wouldn't we want to create our Array with more than 0 places? I was expecting to create one with 5+ or setup some kind of code where this can be increased.
1 Answer

Gavin Ralston
28,770 PointsWell, we need a treets array, so you start out by declaring a variable (which, importantly, is NOT YET an array) and assigning a tiny array to it:
Treet[] treets = new Treet[0];
Once you get to actually opening the file handler and reading the input, you're going to completely replace the array anyway (remember, array sizes can't be changed once they're set)
So there's no point in putting any additional space in the array to hold more treets, since you (presumably) don't know how many there will be anyway, and under-filling it can be just as bad as over-filling it.
You could just initialize by having it not point to any array at all:
Treet[] treets = null;
...and that would work just fine, because pointing to nothing is as good as pointing to an array, as far as Java is concerned. It just wants some kind of value to be stored in the variable. (Treet[] treets)
As long as it has some kind of appropriate value to start, it'll work just fine until you're ready to replace the initial value (null, or an array with a single Treet) which happens immediately afterward.
My guess is it's just a best practice to actually create the array (= new Treet[0]) rather than run the risk of a null pointer exception to only save yourself a few keystrokes, and maybe serves as a better visual cue.
Tony Brackins
28,766 PointsTony Brackins
28,766 PointsGot it. Thx!