Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial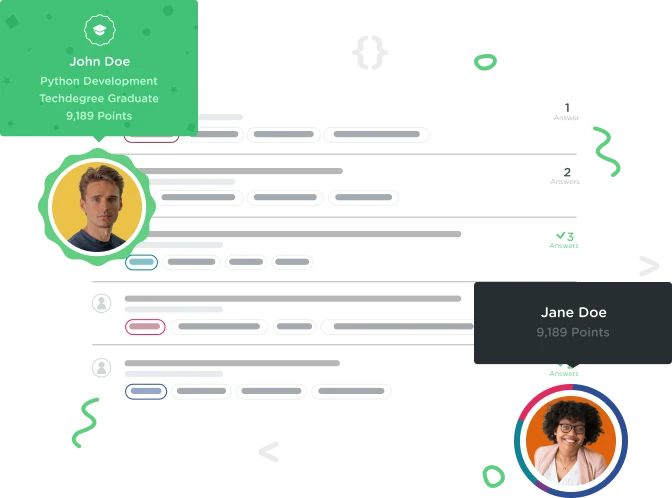
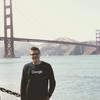
Ben Ahlander
7,528 Points$newPet remove function not working
We created $newPet. The last span element contains an x. if the user clicks that, the section should be removed. This is not working. Not sure why. The console is not showing any errors.
Thanks!
$('.loc').hover(
function(){
$(this).html("<strong>Location:</strong> Your house?!");
},
function() {
$(this).html("<strong>Location:</strong> Treehouse Adoption Center");
});
// Adds a pet to the page with user input on button click
$('#add-pet').on('click', function() {
// Grab info from the form
var $name = $('#pet-name').val();
var $species = $('#pet-species').val();
var $notes = $('#pet-notes').val();
//Assemble the HTML of our new element with the above variables
var $newPet = $(
'<section class="six columns"><div class="card"><p><strong>Name:</strong> ' + $name +
'</p><p><strong>Species:</strong> ' + $species +
'</p><p><strong>Notes:</strong> ' + $notes +
'</p><span class="close">×</span></div></section>'
);
// Attach the new element to the page
$('#posted-pets').append($newPet);
});
// Puppy images fade in
$('img').css('display', 'none').fadeIn(1600);
$('.card').on('click', function() {
$(this).toggleClass('selected');
});
// Make the 'x' in the corner remove the section it's contained within
$('.close').on('click', function(){
$(this).parent().remove();
});
2 Answers

Kelly Musgrove
3,504 PointsHi Ben, The event handler code you've added needs to be inside of the anonymous function to add a pet $('#add-pet'). Basically, adding the pet and being able to remove the pet are all part of the same function -- otherwise it won't work. Rather than adding the $('.close') code to the bottom, add it before the closing brackets of the $('#add-pet') event handler. Here's the code. I've added some comments and extra space to make the main event handler/function more obvious. Just look for the comments with !!! :
$('.loc').hover(
function(){
$(this).html("<strong>Location:</strong> Your house?!");
},
function() {
$(this).html("<strong>Location:</strong> Treehouse Adoption Center");
});
// Adds a pet to the page with user input on button click
// !!! This is the opening bracket of the $('#add-pet') event handler
$('#add-pet').on('click', function() {
// Grab info from the form
var $name = $('#pet-name');
var $species = $('#pet-species');
var $notes = $('#pet-notes');
//Assemble the HTML of our new element with the above variables
var $newPet = $(
'<section class="six columns"><div class="card"><p><strong>Name:</strong> ' + $name.val() +
'</p><p><strong>Species:</strong> ' + $species.val() +
'</p><p><strong>Notes:</strong> ' + $notes.val() +
'</p><span class="close">×</span></div></section>'
);
// Attach the new element to the page
$('#posted-pets').append($newPet);
// !!! PUT YOUR CODE HERE Make the 'x' in the corner remove the section it's contained within
$('.close').on('click', function() {
$(this).parent().remove();
});
// Reset form fields
$name.val("");
$species.val("Dog");
$notes.val("");
}); //!!! This is the closing bracket for the $('#add-pet') event handler
// Puppy images fade in
$('img').css('display', 'none').fadeIn(1600);
$('.card').on('click', function() {
$(this).toggleClass('selected');
});
Hope that helps!

Hugo Paigneau
4,253 PointsYou just forgot to identify specially the parents : this code works for me
$('.close').on('click', function() {
$(this).parent('.card').parent('section').remove();
});