Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial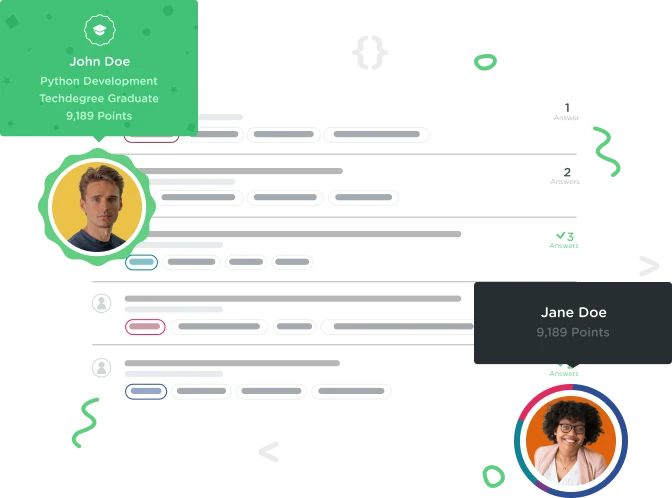
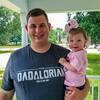
Eric Wilson
9,380 PointsNewtonsoft.Json.JsonReaderException Input string '0.64' is not a valid integer.
I keep getting a Newtonsoft.Json.JsonReaderException that says "Input string '0.64' is not a valid integer. I can't figure out how to fix the issue.
Here is my Program.cs file:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
using Newtonsoft.Json;
using System.Net;
using System.Net.Http.Headers;
using System.Net.Http;
namespace SoccerStats
{
class Program
{
static void Main(string[] args)
{
string currentDirectory = Directory.GetCurrentDirectory();
DirectoryInfo directory = new DirectoryInfo(currentDirectory);
var fileName = Path.Combine(directory.FullName, "SoccerGameResults.csv");
var fileContents = ReadSoccerResults(fileName);
fileName = Path.Combine(directory.FullName, "players.json");
var players = DeserializePlayers(fileName);
var topTenPlayers = GetTopTenPlayers(players);
foreach (var player in topTenPlayers)
{
var playerName = $"{player.FirstName} {player.SecondName}";
Console.WriteLine(playerName);
List<NewsResult> newsResults = GetNewsForPlayer(string.Format("{0} {1}", player.FirstName, player.SecondName));
SentimentResponse sentimentResponse = GetSentimentResponse(newsResults);
foreach (var sentiment in sentimentResponse.Docs)
{
foreach (var result in newsResults)
{
if (result.Headline == sentiment.Id)
{
double score;
if (double.TryParse(sentiment.Sentiment, out score))
{
result.SentimentScore = score;
}
break;
}
}
}
foreach (var result in newsResults)
{
Console.WriteLine(string.Format("Sentiment Score: {0} Date: {1:f}, Headline: {2}, Summary: {3} \r\n", result.SentimentScore, result.DatePublished, result.Headline, result.Summary));
Console.ReadKey();
}
}
fileName = Path.Combine(directory.FullName, "topten.json");
SerializePlayersToFile(topTenPlayers, fileName);
}
public static string ReadFile(string fileName)
{
using (var reader = new StreamReader(fileName))
{
return reader.ReadToEnd();
}
}
public static List<GameResult> ReadSoccerResults(string fileName)
{
var soccerResults = new List<GameResult>();
using (var reader = new StreamReader(fileName))
{
string line = "";
reader.ReadLine();
while ((line = reader.ReadLine()) != null)
{
var gameResult = new GameResult();
string[] values = line.Split(',');
DateTime gameDate;
if (DateTime.TryParse(values[0], out gameDate))
{
gameResult.GameDate = gameDate;
}
gameResult.TeamName = values[1];
HomeOrAway homeOrAway;
if (Enum.TryParse(values[2], out homeOrAway))
{
gameResult.HomeOrAway = homeOrAway;
}
int parseInt;
if (int.TryParse(values[3], out parseInt))
{
gameResult.Goals = parseInt;
}
if (int.TryParse(values[4], out parseInt))
{
gameResult.GoalAttempts = parseInt;
}
if (int.TryParse(values[5], out parseInt))
{
gameResult.ShotsOnGoal = parseInt;
}
if (int.TryParse(values[6], out parseInt))
{
gameResult.ShotsOnGoal = parseInt;
}
double possessionPercent;
if (double.TryParse(values[7], out possessionPercent))
{
gameResult.PosessionPercent = possessionPercent;
}
soccerResults.Add(gameResult);
}
}
return soccerResults;
}
public static List<Player> DeserializePlayers(string fileName)
{
var players = new List<Player>();
var serializer = new JsonSerializer();
using (var reader = new StreamReader(fileName))
using (var jsonReader = new JsonTextReader(reader))
{
players = serializer.Deserialize<List<Player>>(jsonReader);
}
return players;
}
public static List<Player> GetTopTenPlayers(List<Player> players)
{
var topTenPlayers = new List<Player>();
players.Sort(new PlayerComparer());
int counter = 0;
foreach (var player in players)
{
topTenPlayers.Add(player);
counter++;
if (counter == 10)
break;
}
return topTenPlayers;
}
public static void SerializePlayersToFile(List<Player> players, string fileName)
{
var serializer = new JsonSerializer();
using (var writer = new StreamWriter(fileName))
using (var jsonWriter = new JsonTextWriter(writer))
{
serializer.Serialize(jsonWriter, players);
}
}
public static string GetGoogleHomePage()
{
using (var webClient = new WebClient())
{
byte[] googleHome = webClient.DownloadData("https://www.google.com");
using (var stream = new MemoryStream(googleHome))
using (var reader = new StreamReader(stream))
{
return reader.ReadToEnd();
}
}
}
public static List<NewsResult> GetNewsForPlayer(string playerName)
{
var results = new List<NewsResult>();
using var webClient = new WebClient();
webClient.Headers.Add("Ocp-Apim-Subscription-Key", "7ea759046e5343218afe9da0599ac724");
byte[] searchResults = webClient.DownloadData(string.Format("https://api.bing.microsoft.com/v7.0/news/search?q={0}&mkt-en-us", playerName));
var serializer = new JsonSerializer();
using (var stream = new MemoryStream(searchResults))
using (var reader = new StreamReader(stream))
using (var jsonReader = new JsonTextReader(reader))
{
results = serializer.Deserialize<NewsSearch>(jsonReader).NewsResults;
}
return results;
}
public static SentimentResponse GetSentimentResponse(List<NewsResult> newsResults)
{
var sentimentResponse = new SentimentResponse();
var sentimentRequest = new SentimentRequest();
sentimentRequest.Documents = new List<Document>();
foreach (var result in newsResults)
{
sentimentRequest.Documents.Add(new Document { Id = result.Headline, Text = result.Summary });
}
using var webClient = new WebClient();
webClient.Headers.Add("Ocp-Apim-Subscription-Key", "b7b73b68d795435f8d155a8b641df466");
webClient.Headers.Add(HttpRequestHeader.ContentType, "application/json");
string requestJson = JsonConvert.SerializeObject(sentimentRequest);
byte[] requestBytes = Encoding.UTF8.GetBytes(requestJson);
byte[] response = webClient.UploadData("https://soccerstatsnlp.cognitiveservices.azure.com/text/analytics/v3.1/sentiment", requestBytes);
string sentiments = Encoding.UTF8.GetString(response);
sentimentResponse = JsonConvert.DeserializeObject<SentimentResponse>(sentiments);
return sentimentResponse;
}
}
}
Here is my SentimentResponse class:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
namespace SoccerStats
{
// Root myDeserializedClass = JsonConvert.DeserializeObject<Root>(myJsonResponse);
public class ConfidenceScores
{
[JsonProperty("negative")]
public int Negative { get; set; }
[JsonProperty("neutral")]
public int Neutral { get; set; }
[JsonProperty("positive")]
public int Positive { get; set; }
}
public class Relation
{
[JsonProperty("ref")]
public string Ref { get; set; }
[JsonProperty("relationType")]
public string RelationType { get; set; }
}
public class Target
{
[JsonProperty("confidenceScores")]
public ConfidenceScores ConfidenceScores { get; set; }
[JsonProperty("length")]
public int Length { get; set; }
[JsonProperty("offset")]
public int Offset { get; set; }
[JsonProperty("relations")]
public List<Relation> Relations { get; set; }
[JsonProperty("sentiment")]
public string Sentiment { get; set; }
[JsonProperty("text")]
public string Text { get; set; }
}
public class Assessment
{
[JsonProperty("confidenceScores")]
public ConfidenceScores ConfidenceScores { get; set; }
[JsonProperty("isNegated")]
public bool IsNegated { get; set; }
[JsonProperty("length")]
public int Length { get; set; }
[JsonProperty("offset")]
public int Offset { get; set; }
[JsonProperty("sentiment")]
public string Sentiment { get; set; }
[JsonProperty("text")]
public string Text { get; set; }
}
public class Sentence
{
[JsonProperty("targets")]
public List<Target> Targets { get; set; }
[JsonProperty("confidenceScores")]
public ConfidenceScores ConfidenceScores { get; set; }
[JsonProperty("length")]
public int Length { get; set; }
[JsonProperty("offset")]
public int Offset { get; set; }
[JsonProperty("assessments")]
public List<Assessment> Assessments { get; set; }
[JsonProperty("sentiment")]
public string Sentiment { get; set; }
[JsonProperty("text")]
public string Text { get; set; }
}
public class Doc
{
[JsonProperty("confidenceScores")]
public ConfidenceScores ConfidenceScores { get; set; }
[JsonProperty("id")]
public string Id { get; set; }
[JsonProperty("sentences")]
public List<Sentence> Sentences { get; set; }
[JsonProperty("sentiment")]
public string Sentiment { get; set; }
[JsonProperty("warnings")]
public List<object> Warnings { get; set; }
}
public class SentimentResponse
{
[JsonProperty("documents")]
public List<Doc> Docs { get; set; }
[JsonProperty("errors")]
public List<object> Errors { get; set; }
[JsonProperty("modelVersion")]
public string ModelVersion { get; set; }
}
}
This video is tough because the Text Analytics API has changed since it was filmed. According to the Cognitive Services docs, it should be returning the following json response:
{
"documents": [
{
"confidenceScores": {
"negative": 0,
"neutral": 0,
"positive": 1
},
"id": "1",
"sentences": [
{
"targets": [
{
"confidenceScores": {
"negative": 0,
"positive": 1
},
"length": 10,
"offset": 6,
"relations": [
{
"ref": "#/documents/0/sentences/0/assessments/0",
"relationType": "assessment"
}
],
"sentiment": "positive",
"text": "atmosphere"
}
],
"confidenceScores": {
"negative": 0,
"neutral": 0,
"positive": 1
},
"length": 17,
"offset": 0,
"assessments": [
{
"confidenceScores": {
"negative": 0,
"positive": 1
},
"isNegated": false,
"length": 5,
"offset": 0,
"sentiment": "positive",
"text": "great"
}
],
"sentiment": "positive",
"text": "Great atmosphere."
},
{
"targets": [
{
"confidenceScores": {
"negative": 0.01,
"positive": 0.99
},
"length": 11,
"offset": 37,
"relations": [
{
"ref": "#/documents/0/sentences/1/assessments/0",
"relationType": "assessment"
}
],
"sentiment": "positive",
"text": "restaurants"
},
{
"confidenceScores": {
"negative": 0.01,
"positive": 0.99
},
"length": 6,
"offset": 50,
"relations": [
{
"ref": "#/documents/0/sentences/1/assessments/0",
"relationType": "assessment"
}
],
"sentiment": "positive",
"text": "hotels"
}
],
"confidenceScores": {
"negative": 0.01,
"neutral": 0.86,
"positive": 0.13
},
"length": 52,
"offset": 18,
"assessments": [
{
"confidenceScores": {
"negative": 0.01,
"positive": 0.99
},
"isNegated": false,
"length": 15,
"offset": 18,
"sentiment": "positive",
"text": "Close to plenty"
}
],
"sentiment": "neutral",
"text": "Close to plenty of restaurants, hotels, and transit!"
}
],
"sentiment": "positive",
"warnings": []
}
],
"errors": [],
"modelVersion": "2020-04-01"
}
1 Answer
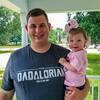
Eric Wilson
9,380 PointsTook this to Stack Overflow and found what was throwing the exception. The positive
, neutral
, and negative
members of my SentimentResponse class should be type double
not type int
.
Here is the fix:
public class ConfidenceScores
{
[JsonProperty("negative")]
public double Negative { get; set; }
[JsonProperty("neutral")]
public double Neutral { get; set; }
[JsonProperty("positive")]
public double Positive { get; set; }
}
However, now my Sentiment score is 0 for every news result. Working on fixing that bug now...