Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial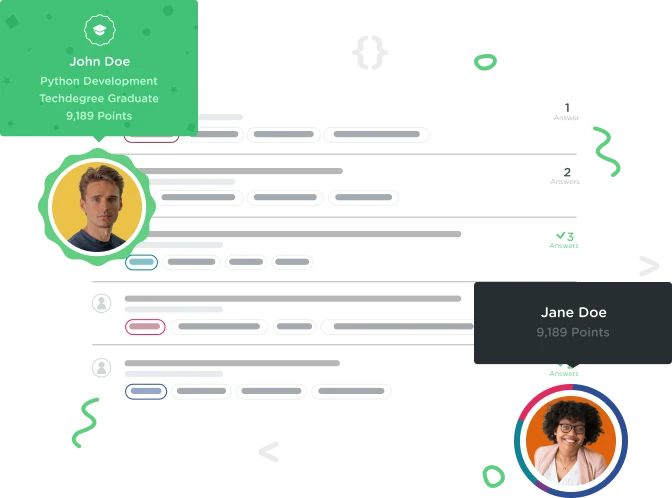
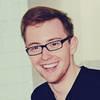
Peter Kolawa
36,765 PointsNext code challenge task is f***ed up!!!
Any idea if it's necessary to check if dir with slug already exists? If yes, then what for if function was meant to create slug??? Isn't it the bad practice?
Any idea how slugify()
should look like to pass the test?
7 Answers

Stephen Cole
Courses Plus Student 15,809 PointsThis was my answer. I really don't like using negation. However, sometimes it is the only way. I prefer to think positively and, sometimes, I have a hard time visualizing negative speech.
When I test to see if there is an invalid first character, I replace it with: stub_
Part of the challenge is to make sure valid characters are used. So, I borrowed code from the builder.py exercise.
I wasn't sure how to handle the optional positional argument of "path." So, I took a guess and gave it a default value of ''.
Personally, I don't enjoy challenges like these. I think the requirements were too vague. I understand that doing things like this help with the learning process. However, there's a point that it becomes an exercise in frustration.
import os
import re
import unicodedata
def slugify(my_string, my_path=''):
my_string = unicodedata.normalize('NFKC', my_string)
if not re.match(r'^[\w\d_]', my_string): # Is the first character valid?
my_string = 'stub_' + my_string
my_string = re.sub(r'\s+', '_', my_string) # Remove spaces.
if my_path:
my_slug = os.path.join(my_path, my_string) # If there's a path, add the stub.
if os.path.exists(my_slug):
print("File exists. Try again.")
return
else:
return my_slug
else:
return my_string
return

Thomas Smith
23,240 PointsI have no idea what it wants us to do. The challenge says a slug should be unique for it's path
What path? Is the function taking a filename or directory name with or without the path attached? Are we supposed to make up our own path to put the files in?
Here's the code I used, which doesn't work because I have no idea what the challenge is asking.
import re
import os
def slugify(string):
if re.match(r'[\w\d_]', string):
string = re.sub(r'\s+', '_', string)
if os.path.exists(string):
pass
else:
return string
else:
pass
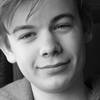
Martin Nørskov Jensen
17,612 PointsI had problems with this code challenge myself but i have finally found my mistake.
The function needs to take two arguments a string which is a directory or file and a path like this
def slugify(string, path):
Futhermore you need to do the following things:
- Use path + string checking if the file exists
- If it does exists use a while loop to change the name until the file name does not exist anymore e.g. filename.txt -> 0filename.txt -> 1filename.txt
- And lastly check if the first character of the file name is a number, letter or underscore
- If not add underscore, letter or number to start
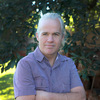
Christopher Shaw
Python Web Development Techdegree Graduate 58,248 PointsThe instructions say you should provide a path: "Your function should accept two arguments, a string to make into an acceptable file or directory name, and a path."

Thomas Smith
23,240 PointsThanks!
This worked
import re
import os
def slugify(string, path):
if not re.match(r'[\w\d_]', string):
string += '_'
string = re.sub(r'\s+', '_', string)
slug = os.path.join(path, string)
while os.path.exists(slug):
slug += '_'
return slug
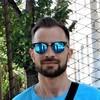
Oszkár Fehér
Treehouse Project Reviewerimport os
import re
import unicodedata
def slugify(string_, path):
string = unicodedata.normalize('NFKC', string_)
string = re.sub(r'[^\w\s]', '', string).strip().lower()
string = re.sub(r'[_\-\s]+', '_', string)
nr = 1
for file in os.scandir(path):
if string in file.name:
string = '({})'.format(nr) + string
nr += 1
return string
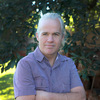
Christopher Shaw
Python Web Development Techdegree Graduate 58,248 PointsWithout overthinking it.
import os
def slugify(name, path):
name = name.strip().lower()
while name.find(' ') > 1:
name = name.replace(' ', ' ')
name = name.replace(' ', '_')
if os.path.exists(os.path.join(path, name)):
return
return name

Ken Rasmussen
3,534 Pointsimport re
import os
def slugify(string, path):
string = re.sub(r'[^\w_]', '', string)
checkpath = os.path.join(path, string)
if not os.path.exists(checkpath):
return checkpath

Lynn Collins
10,080 PointsOkay, I hate to say this, but trying this challenge has been a pure process of 'slugification' for me. Anyway, your tips were wonderful....ty for helping me finally pass it!
pegamoose
16,046 Pointspegamoose
16,046 PointsI was pretty confused about the vague instruction of this challenge. I was able to complete it after finding other answers to your question.