Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial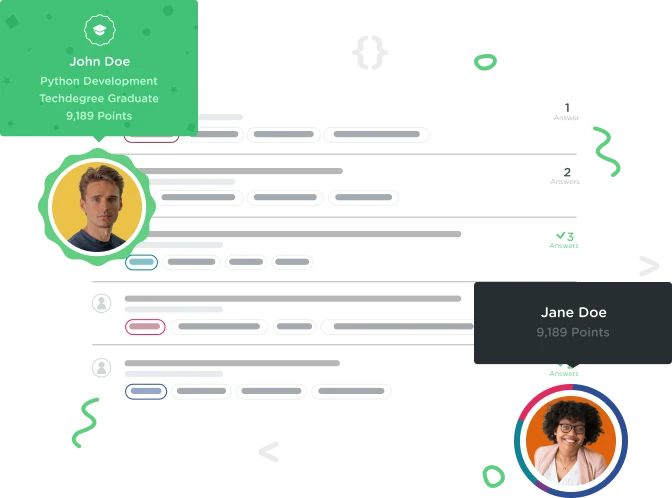

Simon Strömberg
1,423 PointsNext, we need the flavor selected in the dropdown to be submitted to this process.php file. We need to be able to access
What have im done wrong or what am im missing?
<?php
$_POST["flavor"];
?>
<!DOCTYPE html>
<html>
<head>
<title>Ye Olde Ice Cream Shoppe</title>
</head>
<body>
<p>Your order has been created. What flavor of ice cream would you like to add to it?</p>
<form method="$post" action="process.php">
<label for="$_POST">Flavor</label>
<select id="$_POST;">
<option value='$_POST["flavor"];'>— Select —</option>
<option value="Vanilla">Vanilla</option>
<option value="Chocolate">Chocolate</option>
<option value="Strawberry">Strawberry</option>
<option value="Cookie Dough">Cookie Dough</option>
</select>
<input type="submit" value="Update Order">
</form>
</body>
</html>
5 Answers

Christian Andersson
8,712 PointsI see a few errors here Simon,
<?php
$_POST["flavor"];
?>
The above code doesn't actually do anything. You are using the $_POST variable, but you aren't doing anything with it. This is not "wrong", but you should remove it as it does nothing at all.
<form method="$post" action="process.php">
You almost got it right here. The error here is method=$post
. You are telling the browser that the method which the form will be sent is $post
, but $post
to the browser this looks as a variable because you used the dollar-sign. Ultimately the browser doesn't know what to do because you have never used a variable called $post. What you want here is method=POST
, which the browser will understand.
<label for="$_POST">Flavor</label>
<select id="$_POST;">
<option value='$_POST["flavor"];'>— Select —</option>
<option value="Vanilla">Vanilla</option>
<option value="Chocolate">Chocolate</option>
<option value="Strawberry">Strawberry</option>
<option value="Cookie Dough">Cookie Dough</option>
</select>
What you are trying to do here is incorrect for 2 reasons:
(1) The challange wants us to be able to access the selected flavor (Vanilla, Chocolate, etc) by using the variable "$_POST["flavor"]. To do that we need to specify what is "flavor". To do that, we need to give the select
tag the name
attribute. So your solution is:
<select id="flavor" name="flavor">
Now when you click that Submit button, the browser will scan through all tags within the form
and look for any tags that have a name attribute. If they do, they get saved inside the $_POST variable and sent to the next page. Those that don't have a name
attribute will be ignored.
(2) Another error here is the way you tried to use the $_POST variable. You don't need to use it here at all, but when you do, remember that php variables (such as $_POST) or other php code MUST BE inside a <?php ?> tag. Otherwise your browser won't be able to tell what is HTML and what is PHP. So if you were to use php inside HTML it should look like this:
<a href="<?php echo $_POST['variable_name']; ?>">www.google.com</a>
Again, this has nothing to do with the code challange you are having problem with. Just a reminder of how to correctly use php inside html for when you do.
Good luck!

Paul Bentham
24,090 PointsHi SImon,
This should work for you, I've commented to try and help you understand.
Christian Andersson deserves full credit for a very clear description above.
<form action="process.php" method="post"> /* post needs to be lower case, not sure if this is necessary but task won't pass without it being lowercase */
<label for="flavor">Flavor</label> /* No need for the $_POST here */
<select id="flavor;" name="flavor;">
<option value=''>— Select —</option> /* Value is intentionally empty for this as this is a helper instruction */
<option value="Vanilla">Vanilla</option>
<option value="Chocolate">Chocolate</option>
<option value="Strawberry">Strawberry</option>
<option value="Cookie Dough">Cookie Dough</option>
</select>
<input type="submit" value="Update Order">
</form>```

Simon Strömberg
1,423 PointsThis is the "question":
Next, we need the flavor selected in the dropdown to be submitted to this process.php file. We need to be able to access it in the “flavor” element of the $_POST array, like this: $_POST["flavor"]. What two changes do we need to make to the HTML? (Hint. We need to add two new attributes. The first needs to be added to one existing HTML element, the second needs to be added to a different existing HTML element.)
Your code doesnt get approved in step 2.
Regards

Paul Bentham
24,090 PointsSorry Simon, you also need to remove the semi colons after flavor in the select element ID & Name.
<select id="flavor;" name="flavor;">
Should be:
<select id="flavor" name="flavor">

Paul Bentham
24,090 PointsCheck out the answer on this question, see if it helps you out with what you need.
https://teamtreehouse.com/forum/html-forms-php
You don't need all of the $_POST's that you currently have in your html.

Tinashe Bvukumbwe
14,089 PointsInside the section tag add a name attribute and set its value to flavor that is <section name="flavor"></section>

Simon Strömberg
1,423 Points<!DOCTYPE html> <html> <head> <title>Ye Olde Ice Cream Shoppe</title> </head> <body>
<p>Your order has been created. What flavor of ice cream would you like to add to it?</p>
<form method="POST" action="process.php">
<label for="$_POST">Flavor</label>
<select id="flavor;" name="flavor;">
<option value='<?php echo $_POST["flavor"];?>'>— Select —</option>
<option value="Vanilla">Vanilla</option>
<option value="Chocolate">Chocolate</option>
<option value="Strawberry">Strawberry</option>
<option value="Cookie Dough">Cookie Dough</option>
</select>
<input type="submit" value="Update Order">
</form>
</body> </html>

Christian Andersson
8,712 PointsSimon, it looks like you're trying to add actual php code here which is not necessary. Re-read what I wrote above carefully.
You have a problem on each of these 3 lines:
<label for="$_POST">Flavor</label>
<select id="flavor;" name="flavor;">
<option value='<?php echo $_POST["flavor"];?>'>— Select —</option>
Your id
for the select
is almost right. Remove the ;
. Same thing goes for the name
. The ;
are used at the end of each command in php. This row is HTML, not php.
<label for="$_POST">Flavor</label>
This is a label that shows the text "Flavor". The for
attribute tells the browser what the label is "attached to". In this case it's a label for your select
tag, so use it's id here.
<option value='<?php echo $_POST["flavor"];?>'>— Select —</option>
Again, read my last post. You are using php here and you shouldn't do that.
Simon Strömberg
1,423 PointsSimon Strömberg
1,423 PointsGreat, but that didnt work, how about a complete code with issue fixed`?
Christian Andersson
8,712 PointsChristian Andersson
8,712 PointsCan you paste the code that you tried after my suggestions above?