Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial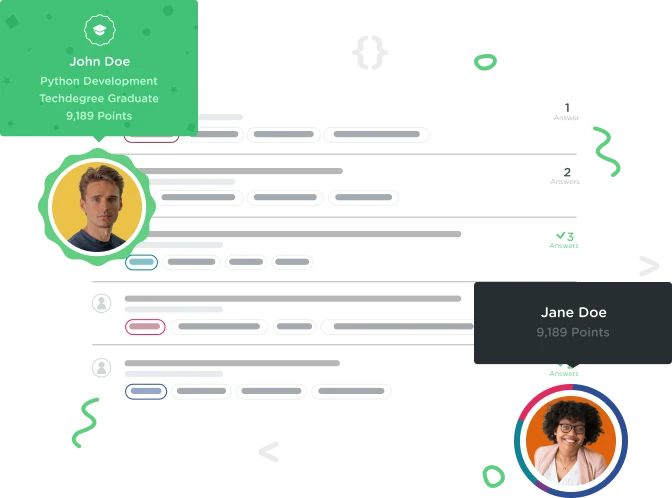
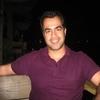
Renan A
4,425 Points`nil`
What is the purpose of the nil
?
1 Answer

Martin Wildfeuer
Courses Plus Student 11,071 PointsIn Swift, nil
means the absence of a value. Consequently, nil
can only be assigned to optional values in Swift. Optionals are a key concept of Swift, I recommend reading Apple Docs: Swift - The Basics
One example would be an integer: we want to know whether a value has been assigned to it and act accordingly. Back in the days you would initialize this variable with 0 or -1 and check against these values to see if the variable has already been changed or not. But what if 0 or -1 were valid values as well? Nil is a much better approach, as a nil value definitely means the absence of a value, so we don't have to worry about initializing optionalInt with some arbitrary number.
// Error, nil cannot be assigned to a non-optional value
let optionalInt: Int = nil
// Works, as we declare optionalInt as optional with ?
let optionalInt: Int? = nil
// As optionalInt is optional, we have to unwrap this value
// "optionalInt is nil"
if let unwrappedInt = optionalInt {
print("optionalInt is not nil")
} else {
print("optionalInt is nil")
}
// Now we set the value to an actual integer
optionalInt = 1
// "optionalInt is not nil"
if let unwrappedInt = optionalInt {
print("optionalInt is not nil")
// Now we can use unwrappedInt here, it is now
// unwrapped and therefore not optional anymore
} else {
print("optionalInt is nil")
}
This is just a very basic example, there lies great potential in how and when to use nil, so make yourself familiar with the optionals concept :)
Hope that helps :)