Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial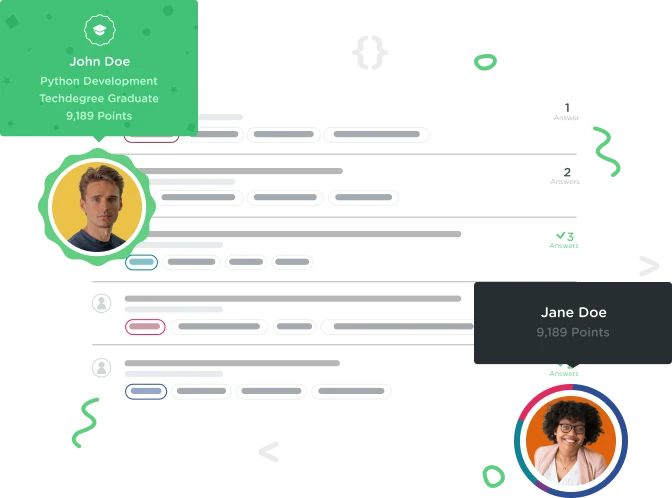

Piotr Manczak
Front End Web Development Techdegree Graduate 29,104 PointsNo access to an array.
I can't acces an array (employeeArrays). I can log it and can see it as a whole, it is there but for some reason I can't access any of its items. I tried different things and it does not work. console.log(employeeArrays[0]) returns undefined. I can't move forward without that array. and when I console.log(employeeArrays.length) it returns 0. ??? https://github.com/PiotrManczak39/techdegree-project-8.git (edited)
const mainContent = document.getElementById('main-content');
const employeeArrays = [];
let index = 0;
fetch('https://randomuser.me/api/?results=12')
.then(response => response.json())
.then(data => {
generateEmployees(data.results);
// console.log(data.results);
})
.catch(error => console.log('Looks like there was a problem.', error))
function generateEmployees(data) {
data.map(result => {
const html = `
<section class = 'card' index = ${index}>
<img class = 'image' src='${result.picture.large}' alt = 'profile-image'>
<h3 class = 'employee-info'>${result.name.first} ${result.name.last}</h3>
<p class = 'employee-info'>${result.email}</p>
<p class = 'employee-info'>${result.location.city}</p>
</section>
`
index += 1;
employeeArrays.push(result);
mainContent.innerHTML += html;
});
}
console.log(employeeArrays[0]);
<!DOCTYPE html>
<html lang="en">
<head>
<!-- Link for mobile devices -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Google fonts -->
<link href="https://fonts.googleapis.com/css?family=Varela+Round&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/normalize.css">
<link rel="stylesheet" href="css/styles.css" >
<title>The Employee Directory</title>
</head>
<body>
<div class="wrapper">
<h3>AWESOME STARTUP EMPLOYEE DIRECTORY</h3>
<div id="main-content">
</div>
</div> <!-- End of Wrapper -->
<div class="modal">
</div>
<script src="js/jquery.js"></script>
<!-- <script src="js/app.js"></script> -->
<script src="js/appbis.js"></script>
</body>
</html>
3 Answers
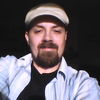
Robert Manolis
Treehouse Guest TeacherHey Piotr Manczak, yes, setTimeout can be used as a sort of workaround in this situation, but it's not the preferred way to handle asynchronous code. Using setTimeout in this way is generally considered to be an unreliable and sort of hacky way to go about it because there's no way to be sure that your API request will resolve before the timer is up. There's no way to accurately predict latency, so it is possible that your array will still be empty even with a four second delay. It's recommended that you use JS Promises or async await to handle this asynchronous code.
Here's the course that walks through those techniques. Hope it helps. :)
https://teamtreehouse.com/library/asynchronous-programming-with-javascript

Torben Korb
Front End Web Development Techdegree Graduate 91,432 PointsHello Piotr,
there is no data in your array available at the time you're logging because it will be assigned in the function call of an asynchronous fetch request (Fetch is always asynchronous). Once you call the fetch method there will be a call to your callback when the response is ready (which can happen at any time). But your other code below will fire first before this happens. This is the reason why employeesArray[0] is undefined when logging.
To move forward your code has to happen inside the callback. Try to log your data inside your generateEmployees function and you'll see results. This is because this function is called when the data is ready.
By the way even if your request would be really fast or you would have many line of codes below the log statement it still would execute all the global code and put the callback of your asynchronous request in a queue. This is defined by something called the event loop and is a JavaScript engine implementation detail.
Hope this helps you. Happy coding!

Piotr Manczak
Front End Web Development Techdegree Graduate 29,104 PointsThanks for your help. Now when you said it I can see more clearly. So let's say I do this:
window.setTimeout(() => {
console.log(employeeArrays[0]);
}, 4000);
that should work assuming that fetch() would take much less than 8sek.

Piotr Manczak
Front End Web Development Techdegree Graduate 29,104 PointsYup. I've just checked that. It is in fact working. Thanks again Torben Corb.
Piotr Manczak
Front End Web Development Techdegree Graduate 29,104 PointsPiotr Manczak
Front End Web Development Techdegree Graduate 29,104 PointsThanks for your advice. I've done that course, but now I can see a practical use of it. I will have to go all over it again, this time with better understanding!