Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial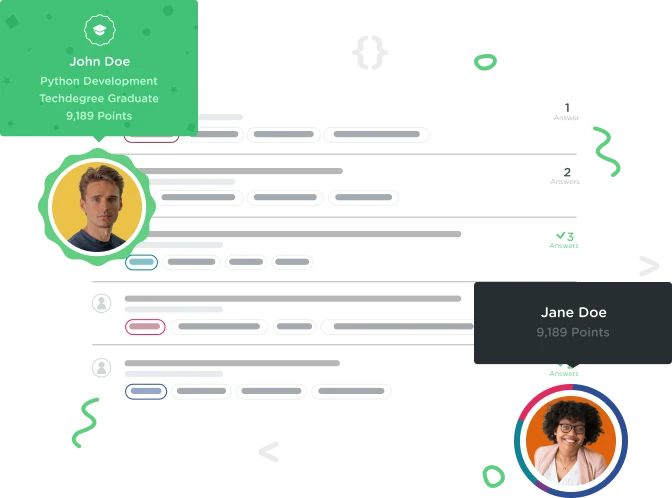

tomd
16,701 PointsNo 'Access-Control-Allow-Origin' when using fetch
I don't really understand whats happening. But I keep getting this error and the images won't load. Here is all my JS code
const select = document.getElementById('breeds');
const card = document.querySelector('.card');
const form = document.querySelector('form');
// ------------------------------------------
// FETCH FUNCTIONS
// ------------------------------------------
function fetchData(url){
return fetch(url)
.then(res => res.json());
}
fetchData('https://dog.ceo/api/breeds/list')
.then( data => generateOptions(data.message));
fetchData('https://dog.ceo/api/breeds/image/random')
.then(data => generateImage(data.message));
// ------------------------------------------
// HELPER FUNCTIONS
// ------------------------------------------
function generateOptions(data){
const options = data.map( item => `
<option value="${item}">${item}</option>
`).join('');
select.innerHTML = options;
}
function generateImage(data){
const html = `
<img src="${data}" alt>
<p>Click to view images of ${select.value}s</p>
`;
card.innerHTML = html;
}
function fetchBreedImage(){
const breed = select.value;
const img = card.querySelector('img');
const p = card.querySelector('p');
fetchData(`https://dog.ceo/api/breed/${breed}/images/random)`)
.then(data => {
img.src = data.message;
img.alt = breed;
p.textContent = `Click to view more ${breed}s`;
})
}
// ------------------------------------------
// EVENT LISTENERS
// ------------------------------------------
select.addEventListener('change', fetchBreedImage);
card.addEventListener('click', fetchBreedImage);
// ------------------------------------------
// POST DATA
// ------------------------------------------
2 Answers
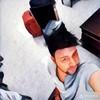
Ari Misha
19,323 PointsHello there! By default, Fetch API is restricted to the same origin policy. Browsers restrict scripts to access the URL 'coz of the safety issues and the same could be done by the Hackers to crawl or access any endpoint for the sensitive data. In order to use fetch for with CORS(Cross-Origin Policy) , you're gonna have to send custom headers with your fetch. It should look something like this:
fetch(someUrl, { mode: 'cors' }).then()
In the above syntax, the additional object is called as options object where you can provide additional Headers or options about the fetch API should work. You can also use { mode: 'no-cors' }
for opaque responses.
I hope it helped!
~ Ari

tomd
16,701 PointsI found the problem, there is a parentheses in my template literal.