Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial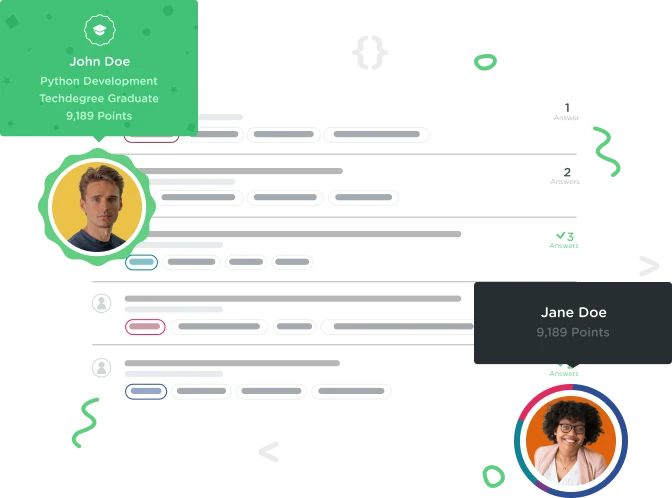

Anup Sheth
18,090 PointsNo Body Property on Request?
Hello,
What's the reason there is no body property when the first middleware function gets called? In this case, we're using Postman to send a POST request, setting Content Type in the HTTP Request header to 'application/json'. When sending a POST request, my understanding is that any information sent to the server is sent via the message body.
So when the first jsonCheck function is called, why does request.body evaluate to false? And where is the information we're sending to our API if not in the request body?
Thanks for your help!
'use strict';
var express = require('express');
var app = express();
var jsonParser = require("body-parser").json;
var jsonCheck = function(request, response, next) {
if (request.body) {
console.log("The sky is", request.body.color);
} else {
console.log("There is no body property on the request");
}
next();
}
app.use(jsonCheck);
app.use(jsonParser());
app.use(jsonCheck);
var port = process.env.PORT || 3000;
app.listen(port, function() {
console.log('Express server is listening on port', port);
});
3 Answers

Donnie Reese
17,211 PointsFrom my understanding, you need some sort of middleware function to read the stream of input from ajax request. I did some lesson on Treehouse AGES ago about ajax and streams and having to read it in chunks and then piecing the chunks together and parsing it. Well, I believe it was on Treehouse.
Basically, though, it isn't some basic object because the ajax request could be getting 1 character or 5 mb image file, and ajax is generally asynchronous and the web sends packets. So, yea, there is that. Hopefully someone smarter can explain it better than that. If I'm not talking complete nonsense, I think it's because ajax could accept any type of content and doesn't determine what it is, because it could be anything. So it just has a stream that needs to be caught and read and it doesn't prescribe how you should do that because there are a million reasons to do it any specific way.

Donnie Reese
17,211 PointsFrom what I understood of your question, you are wondering why req.body returns false before using the jsonParser middleware?
I logged out the req Object to the console when the if statement returned false.
} else {
console.log("There is no body property on the request.");
console.log(req);
}
There did not seem to be a body property. It looks like the jsonParser actually creates the property and, since there is no body property, it would return false.
I hope that helped.

Anup Sheth
18,090 PointsThanks for your comment, Donnie!
It definitely looks like the jsonParser creates the property. I guess I'm just confused as to why it creates the property. My understanding is that when a POST request is sent to a server, a message body is sent after the request header. So I guess I just don't understand why/how the jsonParser would create a property that should already exist before the request header/body hits the middleware.
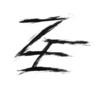
Zachary Eckstein
9,901 PointsThe first call to 'app.use(jsonCheck);' happens before the 'app.use(jsonParser());' can create a readable JSON object. So the code asks, "Do you have a readable JSON file for me?". The first call responds no, then the parser creates a readable JSON object on the body property, then the next call can respond yes and find the body property you are asking for.
Even though the POST request contains a JSON object, it cant be read by express until it is parsed. Pay careful attention to the order your middleware runs in and happy coding!
Anup Sheth
18,090 PointsAnup Sheth
18,090 PointsYou're right! I took at a look at the documentation for body-parser (https://www.npmjs.com/package/body-parser), the middleware we're using to parse incoming request bodies. It says right on the first line: "Parse incoming request bodies in a middleware before your handlers, available under the req.body property."
And this Stack Overflow Q/A (http://stackoverflow.com/questions/4295782/how-do-you-extract-post-data-in-node-js ) also does a great job of explaining how body-parser works.