Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial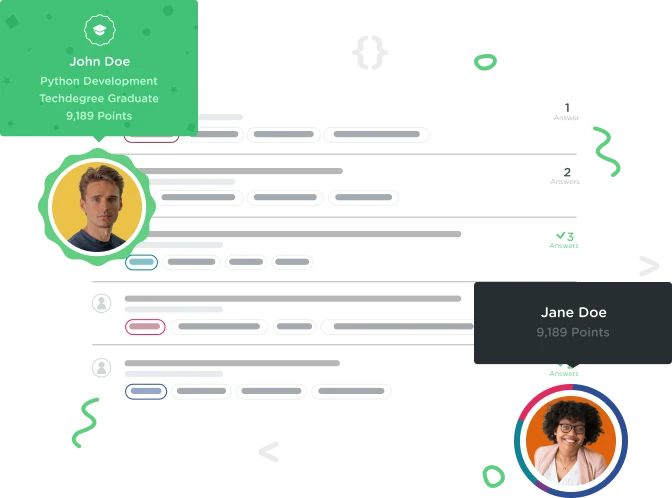
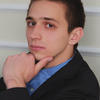
Iskander Ismagilov
13,298 PointsNo difference between variables
Why does shopping_list work if there are two different variables: item and new_item. In add_to_list(item) and in while True loop: new_item = Input(">") and then add_to_list(new_item). I thought those variables should be the same for the programm working.
def add_to_list(item):
shopping_list.append(item)
print("Added! List has {} items.".format(len(shopping_list)))
def show_list():
print("Here is your list:")
for item in shopping_list:
print(item)
show_help()
while True:
new_item = input(">")
if new_item == 'DONE':
show_list()
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list()
continue
add_to_list(new_item)
continue
5 Answers
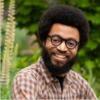
Nicolas Hampton
44,638 PointsIskander,
Just to expand on what's already been said (because what's been said is pretty good), the question you've posed is pretty important in almost all programming languages. When the function is defined, it creates it's own private world to work in. This is what's referred to as the function's "scope". The item argument only exists inside this function's private world, or scope, and will be how that function deals with whatever argument is passed to it when it is used. But when the function is defined, we don't define the value of the argument, we just define the steps we're going to take to handle whatever argument is passed in when we actually run the function.
# here we'll set instructions beforehand for this function to print it's argument
def some_function(argument_placeholder):
print(argument_placeholder)
# Outside of our function definition, we'll define a variable to print
some_argument = 'This is the value printed at runtime'
# result will print 'This is the value printed at runtime'
some_function(some_argument)
Notice that when we pass 'some_argument' into some_function, that function renames some_argument to argument_placeholder while it handles it inside the function.
now let's add one line to the code we have above:
# This will throw an error
some_function(argument_placeholder)
This throws an error if you run it, because argument_placeholder doesn't exist outside of the function, it only exists inside the function for the function alone to use. This is called the function's 'scope', and the area outside of the function is called the 'global scope'. Also, if I were to define a function like this:
external_variable = 10
def some_function(argument_placeholder):
internal_variable = 5
print(internal_variable + external_variable + argument_placeholder)
# This will return 35 because the function can access the external variable
# defined in the global scope
some_function(20)
# This will print 10 because the variable was defined in the global scope
print(external_variable)
# Both of these operations will error, because they are defined inside the function's scope,
# and only exist when the function is ran
print(argument_placeholder)
print(internal_variable)
So the function has access to
- the global scope outside it
- the arguments passed into it at runtime, which it renames
- and any variables defined inside of the function
but outside of the function, the function's definition variables cannot be accessed in the global scope, creating a sort of privacy. This is going to come up a lot in programming, and it's pretty important, so take some time with it. It's connected to a lot, including the idea of a 'closure', and I'm sure Kenneth will get to explaining a lot of this soon, maybe even later in this lesson. For now though, just remember that the variables you use when defining a function only exist inside of that function's world, or scope, and that variables defined outside of a function exist everywhere, in the global scope, and can be accessed inside of functions as well, so be careful naming global variables, because they can overwrite variables inside of a function. I hope this helps, and Happy coding!
Nicolas

Mike Kryski
2,625 PointsIt works because item is a function argument, and new_item is a variable. In order to use a function in python, you need to first define what it is. We do this like so:
def add_to_list (item):
shopping_list.append(item)
print("Added! List has {} items.".format(len(shopping_list)))
# the def keyword means we are DEFINING a function
# add_to_list is the function NAME, which we will use to CALL the function later
# item is the function ARGUMENT, which is basically a place holder for any variable we pass to the function
# The argument name could have been anything we wanted
def add_to_list (foo):
shopping_list.append(foo)
print("Added! List has {} items.".format(len(shopping_list)))
# This is also a valid function definition
Now, when we want to use the function we just defined, we need to call it and pass a variable:
add_to_list(new_item)
# add_to_list is the function we want to use, and we are passing it the variable new_item
# Jumping back to our function definition, the new_item variable replaces the item argument
Hope this helps...
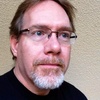
Chris Freeman
Treehouse Moderator 68,423 PointsExtending Mikes answer, when defining a function such as def add_to_list(local_argument)
, a local argument is used to contain the object passed in during the function call add_to_list(some_object)
. Since the function has no idea what object will be passed, this local argument becomes the local reference to that object. When the function call occurs, the local_argument
points to the some_object
.
The id
of an object is its unique location in memory. If the id
s of two objects are equal, the two objects are the same entity.
PS C:\Users\User> python
Python 3.5.0 (v3.5.0:374f501f4567, Sep 13 2015, 02:27:37) [MSC v.1900 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> # Define some function that prints argument and argument id
>>> def some_function(some_argument):
... print("some_argument: {}; id:{}".format(some_argument, id(some_argument)))
...
>>> # Define a list and check its id
>>> lst = [1, 2, 3]
>>> id(lst)
220368429320
>>> # define a string and check its id
>>> dog = "Fluffy"
>>> id(dog)
220368445936
>>> # run the function and see the local argument id of 'lst'
>>> some_function(lst)
some_argument: [1, 2, 3]; id:220368429320
>>> # run the function and see the local argument id of 'dog'
>>> some_function(dog)
some_argument: Fluffy; id:220368445936
Since the id
s match, the local some_argument
and the exterior variable are actually the same object.
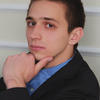
Iskander Ismagilov
13,298 PointsMike, Chris thank you for detailed answers. I got that the local_argument is used as a place holder for objects (such as variables and other elements) and it points to them.
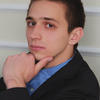
Iskander Ismagilov
13,298 PointsThank you very much, NIcolas! Your answer is very detailed and clear. It helped me and added some comprehension of how function works and uses variables as arguments! Most important for me now is some_argument has to be defined outside of a function for using it at runtime! I will return to your answer later again for getting more comprehension and understanding of arguments, placeholders and functions. Thank's!