Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial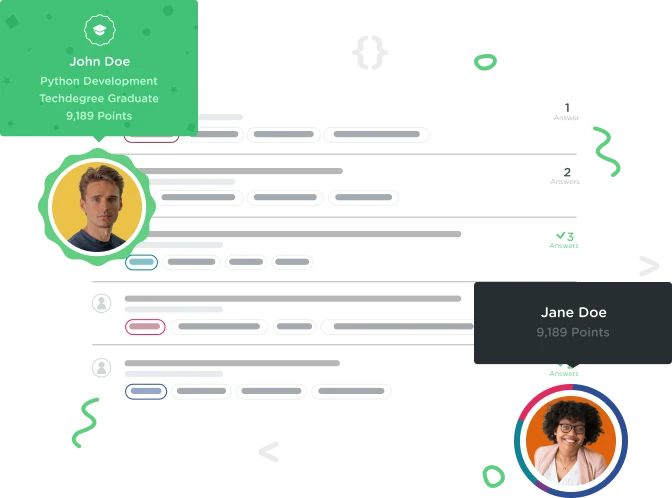

Allen Deckert
9,783 PointsNo Errors but Not Passing Challenge Build Simple iPhone App Swift 3
Not throwing any errors but I can't seem to find out what was wrong. Looked for previous answers to this question and assumed that the challenge is still bugged because of the single quotation mark. but putting \ didn't fix it.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I\'m a machine!")
}
}
// Enter your code below
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up": self.location.y += 1
case "Down": self.location.y -= 1
case "Left": self.location.x += 1
case "Right": self.location.x -= 1
default: break
}
}
}
2 Answers

Thomas Dobson
7,511 PointsHi Allen,
Xcode did not produce the error, but the Treehouse compiler did:
swift_lint.swift:32:19: error: argument names for method 'move(direction:)' do not match those of overridden method 'move' override func move(direction: String) {
looking closer at your code I see two mistakes (See comments):
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) { //You removed the internal name omitance "_" before direction
print("Do nothing! I\'m a machine!")
}
}
// Enter your code below
class Robot: Machine {
override func move(direction: String) { //You removed the internal name omitance "_" before direction
switch direction {
case "Up": self.location.y += 1
case "Down": self.location.y -= 1
case "Left": self.location.x += 1 // Left is -=
case "Right": self.location.x -= 1 // Right is +=
default: break
}
}
}
The final code being:
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! I\'m a machine!")
}
}
// Enter your code below
class Robot: Machine {
override func move(_ direction: String) {
switch direction {
case "Up": self.location.y += 1
case "Down": self.location.y -= 1
case "Left": self.location.x -= 1
case "Right": self.location.x += 1
default: break
}
}
}
Your code compiled fine in Xcode... just not quite what the parameters of the exercise was expecting.

Allen Deckert
9,783 PointsThank you!