Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial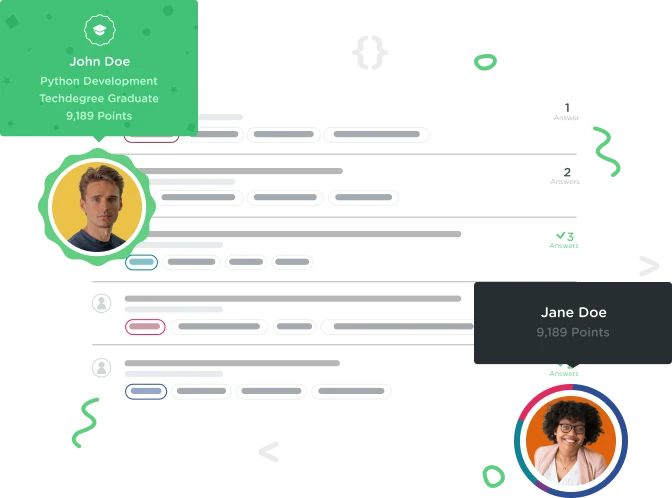

Ron Besser
1,416 PointsNo errors in preview, but code won't pass the challenge. Everything should work I think.
class Robot: Machine {
override func move(direction: String) { switch direction { case "Up" : location.y += 1 case "Down" : location.y -= 1 case "Right" : location.x += 1 case "Left" : location.x -= 1 default : break } } }
This is my code, not sure why its not passing the challenge, but I would love to move on.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I\'m a machine!")
}
}
// Enter your code below
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up" : location.y += 1
case "Down" : location.y -= 1
case "Right" : location.x += 1
case "Left" : location.x -= 1
default : break
}
}
}
2 Answers

Dhanish Gajjar
20,185 PointsIn the code that you have pasted, the external name for the argument for the move method is NOT omitted, it does not exist in the Machine class. The challenge has it, when you start. (look for the underscore before the name "direction")
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! I\'m a machine!")
}
}
So check for that in the challenge, that it is not missing there. After that your code should work fine, because overriding the move method in the Robot class, will include the external name for argument as well.
class Robot: Machine {
override func move(_ direction: String) {
switch direction {
case "Up": location.y += 1
case "Down": location.y -= 1
case "Left": location.x -= 1
case "Right": location.x += 1
default: break
}
}
}
Hope it helps.

Ron Besser
1,416 PointsAwesome. Not sure how that got deleted in the original. Out of curiosity, why would we need to omit the name. I think that is confusing me a bit.
Thanks again!
Ron

Dhanish Gajjar
20,185 PointsRon Besser Using the same example as above, If the external name is not omitted, when you call the function move on an instance of a Robot
let robot = Robot()
robot.move(direction: "Up")
It would read like move ( direction : up )
whereas If it is omitted using an _
let robot = Robot()
robot.move("Up")
This would read like move up. It is not mandatory to always omit the external name. It is what shows up as a label when you call the function. By omitting it, direction becomes the internal name, which you can use within the function, but it won't show up elsewhere.
Hope it's not too confusing, and I have made it clear.