Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial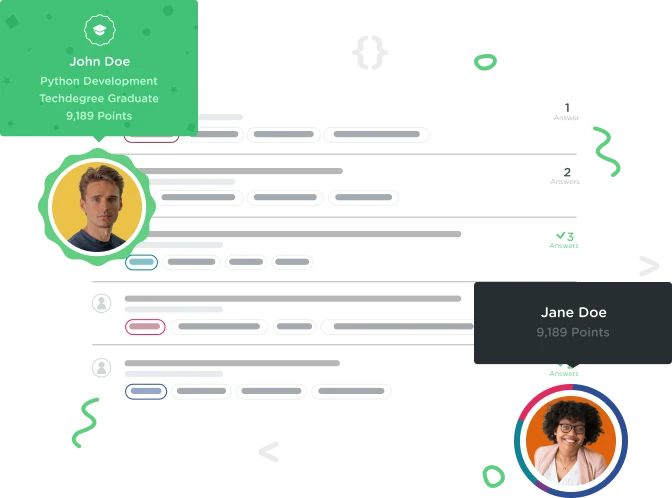
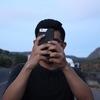
Marc Martinez
12,909 PointsNo fields in Game State (Script)
After I save my code and save my scene, I don't have any fields where I can put stuff like the Game State text, Player, etc. into the Game State (Script) in the Game Manager
Here is my code below
using UnityEngine; using System.Collections; using UnityEngine.UI;
public class GameState : MonoBehaviour {
private bool gameStarted = false;
[SerializeField]
private Text gameStateText;
[SerializeField]
private GameObject player;
[SerializeField]
private BirdMovement birdMovement;
[SerializeField]
private FollowCamera followCamera;
private float restartDelay = 3f;
private float restartTimer;
private PlayerMovement playerMovement;
private PlayerHealth playerHealth;
// Use this for initialization
void Start () {
Cursor.visible = false;
playerMovement = player.GetComponent<PlayerMovement> ();
playerHealth = player.GetComponent<PlayerHealth> ();
// Prevent player from moving at start of the game
playerMovement.enabled = false;
// Prevent bird from moving at start of the game
birdMovement.enabled = false;
// Prevent the follow camera from moving to its game position
followCamera.enabled = false;
}
// Update is called once per frame
void Update () {
// If the game is not started and the player presses the spacebar...
if (gameStarted == false && Input.GetKeyUp(KeyCode.space)) {
//... start the game
StartGame();
}
// If the player is no longer alive...
if (playerHealth.alive == false) {
//... end the game...
EndGame();
//... increment a timer to count up to restarting...
restartTimer = restartTimer + Time.deltaTime;
//... and if it reaches the restart delay...
if (restartTimer >= restartDelay) {
//... then reload the currently loaded level.
Application.LoadLevel (Application.loadedLevel);
}
}
}
private void StartGame () {
// Set the game state
gameStarted = true;
// Removing the start text
gameStateText.color = Color.clear;
// Allow player to move
playerMovement.enabled = true;
// Allow bird to move
birdMovement.enabled = true;
// Allow camera to move
followCamera.enabled = true;
}
private void EndGame () {
// Set the game State
gameStarted = false;
// Show the game over text
gameStateText.color = Color.white;
gameStateText.text = "Game Over";
// Remove the player from the game
player.SetActive (false);
}
}