Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial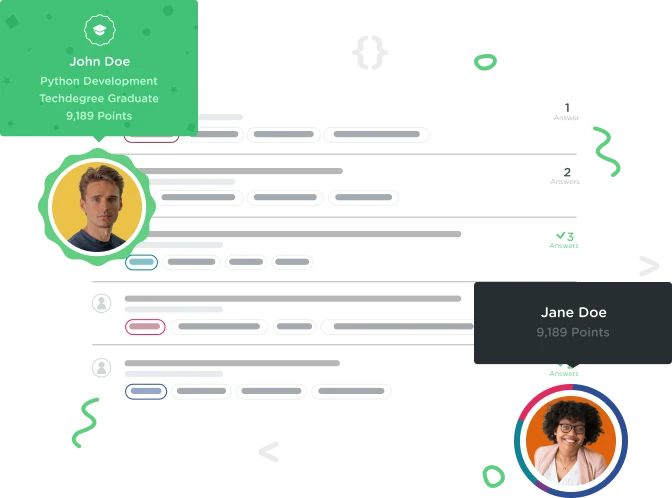
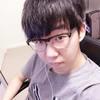
Mingzhe Zheng
27,273 PointsNo idea how to get the first letter in String
Next we need to define a function that we can apply as an operation on a String literal. Create a function named firstLetter that takes a string and returns a string. In the body, return the first character of the string passed in.
Hint: To get individual letters in a string, use the characters property, which returns an array of Character type.
// Enter your code below
extension String {
func modify(sth: String->String)->String{
return sth(self)
}
}
func firstLetter(sth: String)->String{
return "sth".characters[0]
}
3 Answers
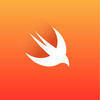
Steven Deutsch
21,046 PointsHey Mingzhe Zheng,
You can just use the .first property to retrieve the first value from the characters array. Your function has a return type of String so make sure you convert the first character in the array back to a String before returning the value.
// Enter your code below
extension String {
func modify(sth: String->String)->String{
return sth(self)
}
}
func firstLetter(sth: String)->String{
return String(sth.characters.first!)
}
Good Luck

Patrick Wiseman
12,215 PointsI had the same error and got it to work by force unwrapping the string returned in firstLetter:
func firstLetter(sth: String)->String{
return String(sth.characters.first!)
}
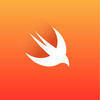
Steven Deutsch
21,046 PointsYes because the .first property returns an optional value. The return type of the function is a non-optional type. If you don't force unwrap then you will return an optional value that mismatches the return type for the function.
Good catch

Jacky Chung
10,913 PointsThis is how I implement it. Passan said we should minimize the use of force unwrapping.
func firstLetter(a: String) -> String {
return String(a[a.startIndex])
}
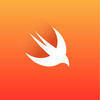
Steven Deutsch
21,046 PointsGood work! I like it, but this will still give us an error if we pass in an empty String. Subscripting an array isn't safe if the index we pass in is out of range. Unlike dictionaries, the value returned is not always an optional.
For example:
let numbers = [1, 2, 3, 4, 5, 6]
print(numbers[6]) // runtime error index out of range
let moreNumbers: [Int] = []
print(moreNumbers[0]) // can't subscript from empty array
What I wanted to do for this challenge is return an optional String. That would be the safest way to handle this situation, but the challenge doesn't accept an optional return type.
func firstLetter(a: String) -> String? {
guard let character = a.characters.first else { return nil }
return String(character)
}
Mingzhe Zheng
27,273 PointsMingzhe Zheng
27,273 PointsIt's not working Error: Make sure the firstLetter function returns the first letter of any string passed in
Steven Deutsch
21,046 PointsSteven Deutsch
21,046 PointsMake sure you are calling the characters.first property chain on the function parameter sth. In the example above you are calling it on the string literal "sth".