Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial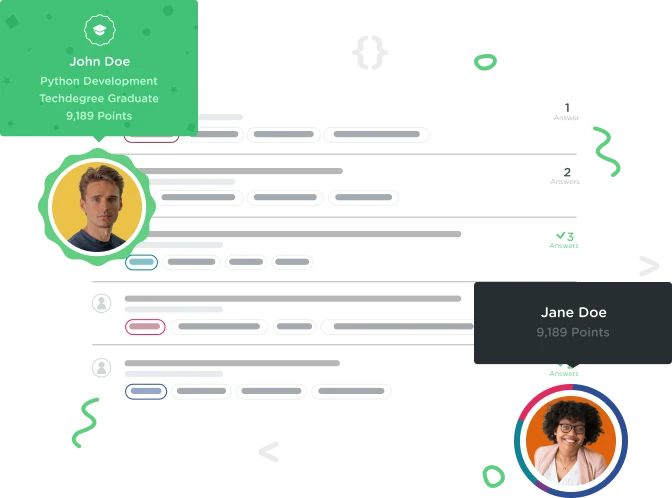
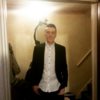
James Barrett
13,253 PointsNo idea what is happening and how things are being called in the promptForGuess method!
Really struggling to get to grips with the promptForGuess method:
public boolean promptForGuess() {
Console console = System.console();
boolean isHit = false;
boolean isValidGuess = false;
while (!isValidGuess) {
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
try {
isHit = mGame.applyGuess(guess);
isValidGuess = true;
} catch (IllegalArgumentException iae) {
console.printf("%s. Please try again.\n", iae.getMessage());
}
}
return isHit;
}
I feel like I have just been bombarded with information on what things are being called from other methods.
Thanks, James.
4 Answers
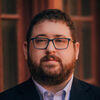
Jonathan Petersen
45,721 PointsSo im going to start from the top of the method and work my way down.
-
Method signature
- Public visibility – everyone can see it / access this method
- Returns data with the type Boolean (true/false)
- promptForGuess is the method name
Get an instance of the console
Define two Boolean type variables isHit and isValidGuess
-
Open a while loop. This loop will continue until the condition is evaluated to false.
- The character '!' means not
- This line reads like , while not a valid guess. So this loop will run until the value of isValidGuess is equal to true.
-
Prompt the user for a string, by displaying the message “Enter a letter: ”
- The string value received from the user is stored in a variable named guessAsString
Get the first character of the string and store it in a char variable named guess
Open a try catch block, this will let you catch any exception aka. Abnormal program flow.
-
mGame is a class variable but it will point to an instance of a class, the Game class.
- The applyGuess instance in the game class, can return either true, false, or throw an IllegalArgumentException
- If the IllegalArgumentException is thrown then it will be caught by the catch block, which prints Please try again preceded by the exception message, and the loop continues
If an exception is not thrown then isValidGuess is set to true, and the loop will terminate because the condition !isValidGuess will evaluate to false.
The method promptForGuess returns the Boolean value that was stored in isHit.
All variables defined in this method will be out of scope and marked for garbage collection after the line 'return isHit;' runs.
I hope this helps. If you have anything you would like me to drill down into further, let me know. Thanks.
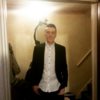
James Barrett
13,253 PointsThanks for the answer, Jonathon.
I'm still struggling to fully get my head around it :( So what you are saying is that !isValidGuess is still esentially saying: 'while false'? If so why is the '!' not inverting it?
Sorry if I am souding silly I just really want to grasp this, it feels like that ! operator has always inverted a boolean value
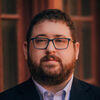
Jonathan Petersen
45,721 PointsYou are correct is does invert it. That part I think you understand. What I think you are not getting is that it is not being assigned to the variable. To assign a value to a variable in java you need to use a single = .
Example
String myString = "Hello";
int myInt = 5;
boolean myBool = true;
This is assignment. It means take the value on the right and place it into the variable on the left. Notice it is only using a single equals. This is how you set a variable.
while(!isValidGuess){
}
There is not single = in that code so the value stored in isValidGuess is never changed.
isValidGuess = true;
Here I am using a single = so the value of isValidGuess has been set to true.
When the JVM evaluates an expression, it does not change the value of the variable, it just wanted to know the end result.
Think about this
1) x = 2
2) y = 3
3) x + y = z
So z = 5.
What is the value of x? What is the value of y?
x is still equal to 2 ..Right.
y is still equal to 3 .. Right.
So even though it evaluated to 5 the variables did not change. The value of x does not change anywhere. The value of y does not change.
This is the same thing. Java is only looking at what the expression evaluates to. It is not changing any variable.
I hope this helps.
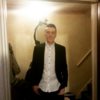
James Barrett
13,253 PointsOk I have just grasped it... Wow that took a long time!
Thanks for the answers :)
James Barrett
13,253 PointsJames Barrett
13,253 PointsI have only just revisited this and I must say this is a fantastic answer! What I do not understand is the isValidGuess boolean.
isValidGuess is declared as false but the in the while condition it inverts it by using '!'... So surely it already sets it to true? This immediately confuses for the rest of the code in the block.
Thanks! James.
Jonathan Petersen
45,721 PointsJonathan Petersen
45,721 PointsThe variable isValidGuess does not get set to true, in the expression for the while loop. The expression is just evaluated.
Consider the following code
The isValidGuess variable is set to true in the try/catch block. Just after isHit = mGame.applyGuess(guess); .
So if apply guess does NOT throw an IllegalArgumentException (Or any other exception) then the assignment line isValidGuess = true; runs. When the while loop evaluates !isValidGuess it evaluates to "Not True" or false, so the program breaks out of the loop.
Here is the code with comments that may help.