Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial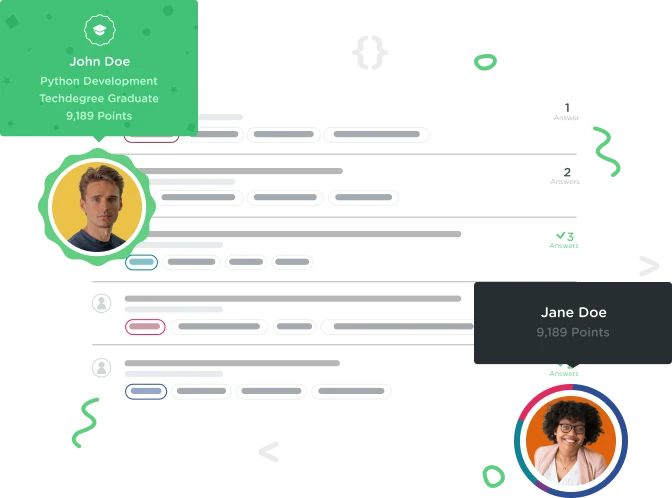

Sierra Lorenzini
838 PointsNo idea what to do
I have absolutely no idea what to do on this one. I've tried so many things and they don't work
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
2 Answers
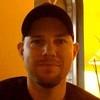
Jeremy Hill
29,567 PointsIn the drive method the challenge wants you to throw a new exception when user attempts to drive more laps than the battery will allow. So you need to add an if statement that will check to see if the number of laps exceeds the current battery level.
The code you need to add in the if statement body is:
throw new IllegalArgumentException("Not enough battery remains!");
After that just add an else statement that will subtract the number of laps from the battery.

Raquel Smith
10,683 PointsHi Sierra,
The first thing you need to do is check whether the number of laps is greater than the bars of battery left. Since you can only drive one lap per battery bar, if you try to drive more laps than you have battery then your car would die.
You can do this check in your if statement:
if (mBarsCount < laps)
or you can do the check first and assign the true/false to a variable:
boolean cantDrive = mBarsCount < laps;
if (cantDrive) {
//throw exception
}
Both of the above will return true if the number of laps exceeds your battery. Because they return true, the if statement will run, which means the exception will be thrown. If the number of laps doesn't exceed your battery (i.e. the if statement returns false), the if statement will not run and no exception will be thrown.
You would leave the mBarsCount -= laps;
statement at the bottom either way. If an exception is thrown, any code below it will not be run. If it's not thrown (i.e. the if statement does not run because the number of laps was NOT more than the battery available), then you need to decrement your battery amount by the number of laps.