Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial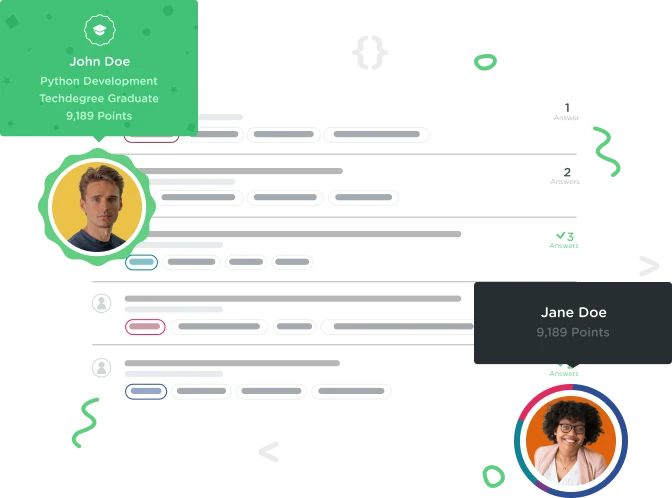

isaac schwartzman
963 Pointsno idea where to go from here
I get the general idea but could someone help me get a better grasp on this
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
import java.util.HashMap;
import java.util.Map;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
public Map<String,Integer> getCategoryCounts (){
Map<String,Integer> categoryCount = new HashMap<String,Integer>();
for(Blog blog : mPost){
for(String Category : mPost.getCategory()){
Integer cCount = }
}
}
}
}
Mike Papamichail
4,883 PointsMike Papamichail
4,883 PointsWell, i'll post the answer to the problem below and then explain what happens step by step so that you can understand how this whole thing works and do it on your own:
Explanation:
So to begin with, we declare a Map that will contain keys of type String and their values will be of type Integer(Declaration using the interface on the left, and implementation on the right). Next, we create the method's structure and then we begin thinking about the code that will go into it. We know that we want to add every unique category of all :
BlogPost post
objects to the Map and also add their count value. So, the getPosts() method returns a List of BlogPost objects as seen in your code above.Then, in order for us to loop through each of those BlogPost objects inside that List we use a for loop and then: For each BlogPost post object, we use an Integer named count.Now what this line does:
Integer count = results.get(post.getCategory());
is that it calls the get() method on our map and searches for a key with the same name as the category that is returned when we call the getCategory() method on each BlogPost post object.So, if the category exists inside our map already, it will return a number, otherwise it will return null.Hence we use an if statement to check if that key(category) exists or not.If it doesn't we assign a value to our Integer count variable(in this case 0) so that we can pass it inside our map.
Next up, using our logic: We just found a category that doesn't yet exist inside our map,so since the count was 0 we should actually increase it by one(count++:).After that, calling the put() method on our map, we add this new category(key) to our map and pass in it's value which is the count variable.
results.put(post.getCategory(),count);
Finally, after the loop ends we need to return the map that we just created to the caller by using the :
return results;
statement.
I hope this helps out a bit and if it helped you solve your problem let me know and make sure to mark it as a Best Answer so that others can find the solution too!
PS: Craig Dennis , is the logic here correct? Am i missing something? I'm not quite sure about what would happen if we passed in a count that was null. Would that be a problem ? Thanks in advance.