Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial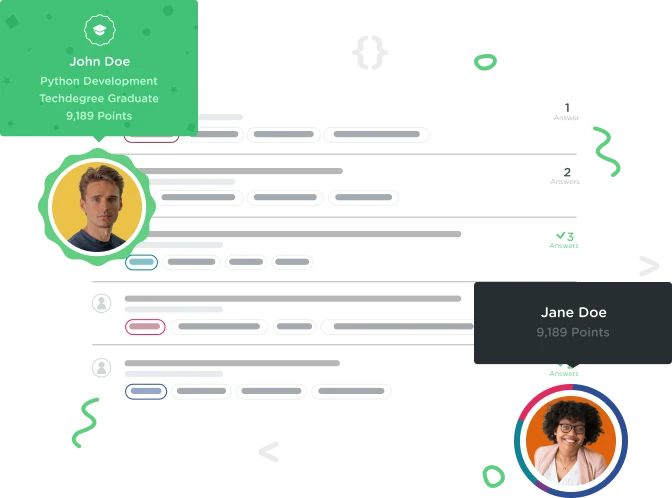
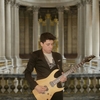
Emerson Gonzalez
Front End Web Development Techdegree Student 22,500 PointsNo Items to display
When I run my App it only shows: No items to display! I'd greatly appreciate any help you can provide!
package com.emersonleaf.blogreader;
import android.app.ListActivity;
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.os.AsyncTask;
import android.os.Bundle;
import android.text.Html;
import android.util.Log;
import android.view.Menu;
import android.widget.ArrayAdapter;
import android.widget.Toast;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
public class MainListActivity extends ListActivity {
protected String[] mBlogPostTitles;
public static final int NUMBER_OF_POSTS = 20;
public static final String TAG = MainListActivity.class.getSimpleName();
protected JSONObject mBlogData;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
if (isNetworkAvailable()) {
GetBlogPostTask getBlogPostTask = new GetBlogPostTask();
getBlogPostTask.execute();
} else {
Toast.makeText(this, "Network is unavailable!", Toast.LENGTH_LONG).show();
}
// Resources resources = getResources();
//mBlogPostTiles = resources.getStringArray(R.array.android_names);
//ArrayAdapter <String> adapter = new ArrayAdapter<String>(this,android.R.layout.simple_list_item_1, mBlogPostTiles);
//setListAdapter(adapter);
//Toast.makeText(this, getString(R.string.no_items),Toast.LENGTH_LONG).show();
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager)
getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main_list, menu);
return true;
}
public void updateList() {
if (mBlogData == null) {
//to do: handle error
} else {
try {
JSONArray jsonPosts = mBlogData.getJSONArray("posts");
mBlogPostTitles = new String[jsonPosts.length()];
for (int i = 0; i < jsonPosts.length(); i++){
JSONObject post = jsonPosts.getJSONObject(i);
String title = post.getString("title");
title = Html.fromHtml(title).toString();
mBlogPostTitles[i] = title;
}
ArrayAdapter <String> adapter = new ArrayAdapter<String>(this,
android.R.layout.simple_list_item_1, mBlogPostTitles);
setListAdapter(adapter);
} catch (JSONException e) {
Log.e(TAG, "Exception caught!", e);
}
}
}
private class GetBlogPostTask extends AsyncTask<Object, Void, JSONObject> {
@Override
protected JSONObject doInBackground(Object[] objects) {
int responseCode = -1;
JSONObject jsonResponse = null;
//URL blogFeedUrl = new URL("http://blog.teamtreehouse.com/api/get_recent_summary/?count =" + NUMBER_OF_POSTS);
try {
URL blogFeedUrl = new URL("http://blog.teamtreehouse.com/api/get_recent_summary/?count=" + NUMBER_OF_POSTS);
HttpURLConnection connection = (HttpURLConnection) blogFeedUrl.openConnection();
connection.connect();
//Creating are response code so that we can get data from the internet
responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
InputStream inputStream = connection.getInputStream();
Reader reader = new InputStreamReader(inputStream);
int nextCharacter; // read() returns an int, we cast it to char later
String responseData = "";
while (true) { // Infinite loop, can only be stopped by a "break" statement
nextCharacter = reader.read(); // read() without parameters returns one character
if (nextCharacter == -1) // A return value of -1 means that we reached the end
break;
responseData += (char) nextCharacter; // The += operator appends the character to the end of the string
jsonResponse = new JSONObject(responseData);
}
} else {
Log.i(TAG, "Unsuccessful HTTP response Code: " + responseCode);
}
} catch (MalformedURLException e) {
Log.e(TAG, "Exception caught: ", e);
} catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
} catch (Exception e) {
Log.e(TAG, "Exception caught: ", e);
}
return jsonResponse;
}
@Override
protected void onPostExecute(JSONObject result) {
mBlogData = result;
updateList();
}
}
}
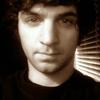
Ryan Farber
5,329 PointsFrom the look of it, you haven't finished the whole BlogReader. It should sort itself out when you finish the application
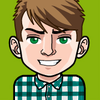
Harry James
14,780 PointsHey Emerson - Are you getting any errors in LogCat? If so, can you provide them?
Sultan Aljaradi
2,592 PointsSultan Aljaradi
2,592 Pointssame problem wit me
i use android studio
help me