Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial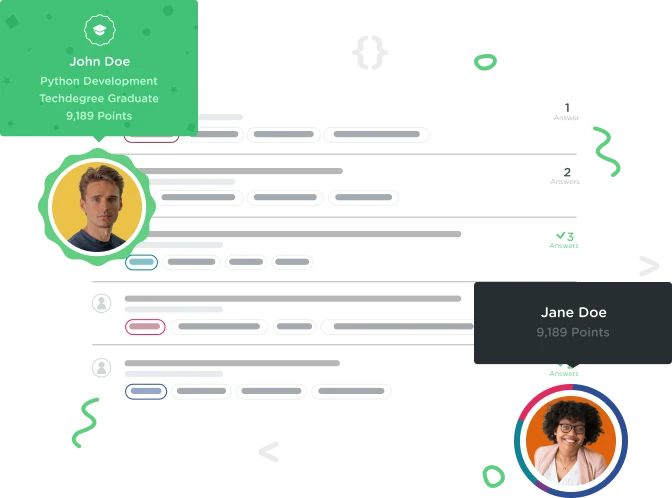

Jeff Styles
2,784 PointsNo output / project file updates out of order
I carefully followed along with this video but am not getting any output when attempting to list the recipes. I'm not getting any syntax errors or missing include files, just no output at all. I'm using a local work environment instead of workspaces so I figured I would just download the project files from the next video (filtering) and compare the code to mine to figure out the issue. However the files for the next video don't seem to be in order. In recipecollection.php the constructor is missing as well as the set and get functions for title. And inc/recipes.php is inexplicably changed to inc/allrecipes.php. In render.php the list recipe function was moved to the bottom of the page and no longer used the asort function. When the proj file code doesn't completely match what the instructor is using it becomes difficult to be sure i'm doing it correctly and leaves room for doubts.
Please see my code below and advise on where I'm going wrong here. Many Thanks!
<?php
include "/Users/Jeff/Sites/classes/recipes.php";
include "/Users/Jeff/Sites/classes/render.php";
include "/Users/Jeff/Sites/inc/recipecollection.php";
include "/Users/Jeff/Sites/inc/recipes.php";
$cookbook = new recipeCollection('Recipe Collection 1');
$cookbook->addRecipe($lemon_chicken);
$cookbook->addRecipe($granola_muffins);
$cookbook->addRecipe($belgian_waffles);
$cookbook->addRecipe($pepper_casserole);
$cookbook->addRecipe($lasagna);
$cookbook->addRecipe($dried_mushroom_ragout);
$cookbook->addRecipe($rabbit_catalan);
$cookbook->addRecipe($grilled_salmon_with_fennel);
$cookbook->addRecipe($pistachio_duck);
$cookbook->addRecipe($chili_pork);
$cookbook->addRecipe($crab_cakes);
$cookbook->addRecipe($beef_medallions);
$cookbook->addRecipe($silver_dollar_cakes);
$cookbook->addRecipe($french_toast);
$cookbook->addRecipe($corn_beef_hash);
$cookbook->addRecipe($granola);
$cookbook->addRecipe($spicy_omelette);
$cookbook->addRecipe($scones);
echo Render::listRecipes($cookbook->getRecipeTitles());
//echo Render::displayRecipe($belgian_waffles);
class RecipeCollection {
private $title;
private $recipes = array();
public function __construct($title = null) {
$this->setTitle($title);
}
public function setTitle($title) {
if(empty($title)){
$this->title = null;
} else {
$this->title = ucwords($title);
}
}
public function getTitle() {
return $this->title;
}
public function addRecipe($recipe) {
$this->recipe=$recipe;
}
public function getRecipes() {
return $this->recipes;
}
public function getRecipeTitles() {
$titles = array();
foreach ($this->recipes as $recipe) {
$titles[] = $recipe->getTitle();
}
return $titles;
} }
class Recipe
{
private $title;
public $ingredients = array();
public $instructions = array();
public $yield;
public $tag = array();
public $source = "Alena Holligan";
private $measurement = array (
"tsp",
"tbsp",
"cup",
"cups",
"oz",
"lb",
"fl oz",
"pint",
"quart",
"gallon"
);
public function __construct($title = null){
$this->setTitle($title);
}
public function __toString(){
$output = " you are calling a " . __CLASS__ . " object with the title \"";
$output .= $this->getTitle() . "\"";
$output .= "</br>"." It is stored in " . basename(__FILE__) . " at " . __DIR__ . ".";
$output .= "</br>"."This display is from the line " . __LINE__ ." in method " . __METHOD__;
$output .= "</br>"."The following method are available for object of this class:"."</br>";
$output .= implode("</br>", get_class_methods( __CLASS__));
return $output;
}
public function setTitle($title)
{
if(empty($title)){
$title = null;
} else {
$this->title = ucwords($title);
} }
public function getTitle() {
return $this->title;
}
public function setYield($yield) {
$this->yield = $yield;
}
public function getYield() {
return $this->yield;
}
public function setSource($source) {
$this->source = $source;
}
public function getSource() {
return $this->source;
}
public function addIngredient($item, $amount = null, $measure = null){
if($amount != null && !is_float($amount) && !is_int($amount)){
exit("the amount must be a float or int: ".gettype($amount)." $amount given");
}
if($measure != null && !in_array(strtolower($measure), $this->measurement)){
var_dump($measure);
exit("please enter a valid measurement: ". implode(", " ,$this->measurement). " are acceptable.");
}
$this->ingredients[] = array(
"item" => ucwords($item),
"amount" => $amount,
"measure" => strtolower($measure)
);
}
public function getIngredients() {
return $this->ingredients;
}
public function addInstruction($string) {
$this->instructions[] = $string;
}
public function getInstructions() {
return $this->instructions;
}
public function addTag($tag) {
$this->tags[] = strtolower($tag);
}
public function getTags() {
return $this->tags;
} }
class Render {
public function __toString() {
$output .= "</br>"."The following methods are available for ". __CLASS__ ." objects:"."</br>";
$output .= implode("</br>", get_class_methods( __CLASS__));
return $output;
}
public static function listIngredients($ingredients) {
$output = "";
foreach($ingredients as $ing) {
$output .= $ing["amount"] . " " . $ing["measure"] . " " . $ing["item"];
$output .= "</br>";
}
return $output;
}
public static function displayRecipe($recipe) {
$output = "";
$output .= $recipe->getTitle() . " by " . $recipe->getSource();
$output .= "</br>";
$output .= implode(", ", $recipe->getTags());
$output .= "</br>";
$output .= self::listIngredients($recipe->getIngredients());
$output .= "</br>";
$output .= implode("</br>", $recipe->getInstructions());
return $output;
}
public static function listRecipes($titles) {
asort($titles);
return implode("</br>", $titles);
} } ?>
Please advise! Thanks!
Jeff Styles
2,784 PointsJeff Styles
2,784 PointsI've solved the main issue I had - in recipecollection.php I simply used $this->recipe = $recipe; But it should be $this->recipes[] = $recipe;
BUT the other issue remains - when needing to reference the project files its confusing if they aren't updated to match the code that the instructor completed in the previous video.