Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial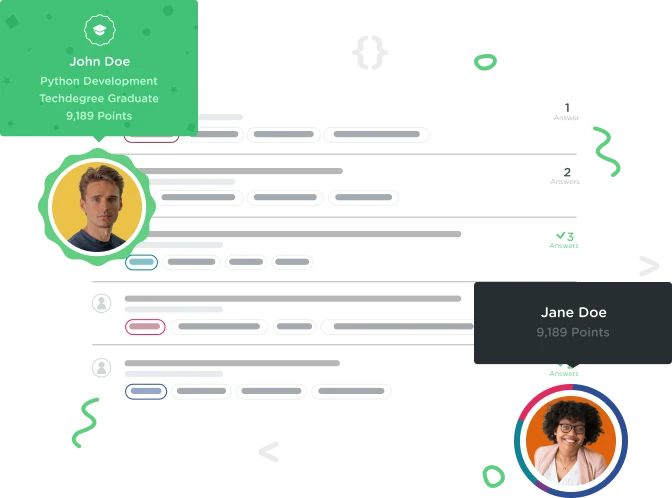

Kari Soto
1,836 PointsNo overload for method 'DistanceTo' takes '1' arguments
I spent forever trying to figure out what is wrong.
Point.cs:
using System;
namespace TreehouseDefense { class Point { public readonly int X; public readonly int Y;
public Point(int x, int y) {
X = x;
Y = y;
}
public int DistanceTo(int x, int y)
{
int xDiff = X - x;
int yDiff = Y - y;
int xDiffSquared = xDiff * xDiff;
int yDiffSquared = yDiff * yDiff;
return (int)Math.Sqrt(xDiffSquared + yDiffSquared);
}
} }
MapLocation.cs:
using System; namespace TreehouseDefense { class MapLocation : Point { public MapLocation(int x, int y, Map map) : base (x, y) { if (!map.OnMap(this)) { throw new OutOfBoundsException(x + "," + y + " is outside the boundaries of the map."); } }
public bool InRangeOf(MapLocation location, int range)
{
return DistanceTo(location) <= range;
}
} class OnPath : Point { public OnPath(int x, int y) : base(x, y) { if(y == 2) { throw new OutOfBoundsException("You can not place a tower on the path. (" + x + "," + y + ")"); }
}
} }
Tower.cs:
// To check if you can place a tower, use this command: // OnPath onPath = new OnPath(x, y);
namespace TreehouseDefense { class Tower { private const int _range = 1; private const int _power = 1;
private readonly MapLocation _location;
public Tower (MapLocation location)
{
_location = location;
}
public void FireOnInaders(Invader[] invaders)
{
foreach(Invader invader in invaders)
{
if(invader.IsActive && _location.InRangeOf(invader.Location, _range))
{
invader.DecreaseHealth(_power);
break;
}
}
}
} }
6 Answers

Vance Rivera
18,322 PointsSo from I can tell is that you need to calculate two values provided as the parameters then return the result as an integer from the DistanceTo function. My question is what does the MapLocation Class look like. If the MapLocation class has two integer properties that you can use to calculate the DistanceTo then i would just pass Maplocation["property name here"] for x and the MapLocation["other property name here"] to the initial DistanceTo function. For example:
//Example MapLocation Class
public class MapLocation{
public int MyCordinates {get; set;}
public int TargetCordinates {get; set;}
public string Name {get; set;}
}
//Now to call the DistanceTo function from your code
public bool InRangeOf(MapLocation location, int range)
{
return DistanceTo(location.MyCordinates, location.TargetCordinates) <= range;
}
Hope this is helpful cheers!

Chris Atchley
18,468 PointsIt was skipped in the videos or I missed it as well, but an overload function that only takes one argument was added to the Point.cs file. After your DistanceTo function you will need to add the overload function like this:
public int DistanceTo(int x, int y)
{
int xDiff = X-x;
int yDiff = Y-y;
int xDiffSquared = xDiff * xDiff;
int yDiffSquared = yDiff * yDiff;
return (int)Math.Sqrt(xDiffSquared + yDiffSquared);
}
public int DistanceTo(Point point)
{
return DistanceTo(point.X, point.Y);
}
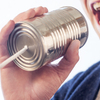
Brian Workman
10,519 PointsNot sure how I missed this, really confused on how this wasn't there. Adding the overload fixed it.
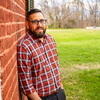
Ryan Watson
5,471 PointsNeed to add an overloaded method to the Point class that takes in a MapLocation object as a single parameter. In the MapLocation class, DistanceTo(), a method of the Point class, is called and passed a location object.
public bool InRangeOf(MapLocation location, int range)
{
return DistanceTo(location) <= range;
}
So in the Point class you need a method that can take "location".
public int DistanceTo(MapLocation location)
{
int xDiff = X-location.X;
int yDiff = Y -location.Y;
double xDiffSquared = Math.Pow(xDiff, 2);
double yDiffSquared = Math.Pow(yDiff, 2);
return (int)Math.Sqrt(xDiffSquared + yDiffSquared);
}
This results in two identically named methods that perform the same function, but take different parameters. This is an example of overloading methods.
public int DistanceTo(int x, int y)
{
int xDiff = X-x;
int yDiff = Y -y;
double xDiffSquared = Math.Pow(xDiff, 2);
double yDiffSquared = Math.Pow(yDiff, 2);
return (int)Math.Sqrt(xDiffSquared + yDiffSquared);
}
public int DistanceTo(MapLocation location)
{
int xDiff = X-location.X;
int yDiff = Y -location.Y;
double xDiffSquared = Math.Pow(xDiff, 2);
double yDiffSquared = Math.Pow(yDiff, 2);
return (int)Math.Sqrt(xDiffSquared + yDiffSquared);
}

Vance Rivera
18,322 PointsThe problem you are having is that you are trying to call the method DistanceTo that take two arguments and you are only passing it one argument. Therefore you would need to create an overload function of DistanceTo that only takes in one parameter. C# is very strict about signatures unlike javascript so when you call a function the arguments you pass it must be the exact type and number of arguments expected. In this case you the compiler is looking for a DistanceTo function that only takes in one argument.
You can create an overload function by creating the same function with a different signature and the compilier will resolve the call based on the name and arguments being passed to the function. so in your case you can have:
public int DistanceTo(int x, int y)
{
int xDiff = X - x;
int yDiff = Y - y;
int xDiffSquared = xDiff * xDiff;
int yDiffSquared = yDiff * yDiff;
return (int)Math.Sqrt(xDiffSquared + yDiffSquared);
}
//overload function that only takes in one argument
public int DistanceTo(MapLocation location)
{
//your code here
}
Hope this helps. Cheers!

Kari Soto
1,836 PointsI figured out what the error means, but I have no idea what I need to replace it with. The video shows what I have, excluding everything in the OnPath class.

Tobi Sam
1,067 PointsChris Atchley is very correct! I missed that too and I've been searching for where the error was. He covered it in the "overloading methods" video. A new DistanceTo overloading method, needs to be added to the Point class.
Thanks Chris.

MD NOMAN HOSSAIN
10,833 PointsThanks, Ryon Watson