Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial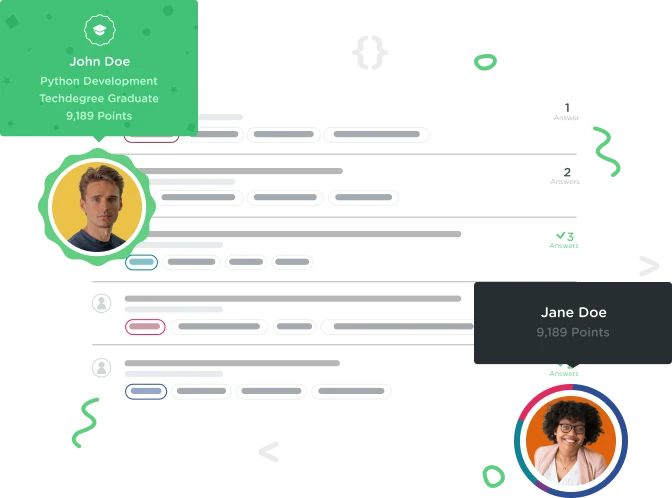

krs dino
Courses Plus Student 778 PointsNo query results for model [Contact]
when i run localhost:8000/todo/create i get this error "No query results for model [Contact]" . help to solving this error
12 Answers
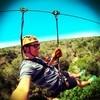
thomascawthorn
22,986 PointsThe routes are matching /todo/{something here}. Therefore /todo/create is calling:
<?php
Route::get('/todos/{id}', "TodoListController@show");
Because it can't find a todo list with an id of 'create', it's saying "No query results for model". If you did this in your todo list show method:
<?php
public function show()
{
return "I am here by accident";
// rest of your code still here
}
you will see you're hitting the wrong method.
Add this to your routes:
<?php
Route::get('/todos/create', "TodoListController@create");
and that should get you to the right place! :-)

krs dino
Courses Plus Student 778 Pointshi Tom Cawthorn,
in the Eloquent ORM section of the video where he just tested if the create function is working...he didn't create a route yet for the create function..all he did was call localhost:8000/todos/create and he got a return from the create function but when i try same i get a No query results for model [Contact] because Contact is the name of my db table....so how do i solve this error
<?php
public function create() { // this code here
return "created new list";
}
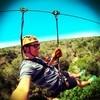
thomascawthorn
22,986 PointsHmm it sounds like you're not getting to your create method. Can you post all of your routes.php file? The error message you're getting back is relating to Eloquent models - which seems strange because in your method, you're not even calling eloquent! So I guess /todo/create is going to the wrong place.

krs dino
Courses Plus Student 778 Pointsthese are my routes
<?php
Route::get('/', "TodoListController@index");
Route::get('/todos', "TodoListController@index");
Route::get('/todos/{id}', "TodoListController@show");
Route::resource('todos', "TodoListController");
Route::get('/db', function(){
return DB::table('contact')->get();
});

krs dino
Courses Plus Student 778 PointsThanks....it was the route with {id} that was causing the problem....thanks

krs dino
Courses Plus Student 778 PointsHi TOM,
now when i use this create function
public function create() { //
$contact = new Contact();
$contact-> first = "new name";
$contact-> last = "dojo";
$contact-> email = "dino_krs@yahoo.com";
$contact-> imageUrl = "dino.jpg";
$contact-> phone = "0707065595";
$contact-> save();
return 'Created new item success';
}
am getting this error
SQLSTATE[42S22]: Column not found: 1054 Unknown column 'updated_at' in 'field list' (SQL: insert into contact
(first
, last
, email
, imageUrl
, phone
, updated_at
, created_at
) values (new name, dojo, dino_krs@yahoo.com, dino.jpg, 0707065595, 2015-01-17 15:20:23, 2015-01-17 15:20:23))
how do i go about this

krs dino
Courses Plus Student 778 Pointsthe problem is larval adds updated_at in my create statement and i don't have a updated_at field in my db ........ larval just keeps add thing unknowingly

krs dino
Courses Plus Student 778 Pointsi just added the updated_at and created_at in my db field and everything is fine...but i think larval should have made a simple switch for one to decide if those fields should be include when creating a new row in the db
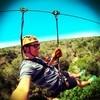
thomascawthorn
22,986 PointsIndeed, laravel will always look for these fields by default. You might try adding:
<?php
$contact->timestamps = false;
I think add this property to the model:
<?php
public $timestamp = false;
might also work.. It's a long time ago since I looked into this so can't quite remember but there is a way to disable the timestamps. Hope this helps.

krs dino
Courses Plus Student 778 Pointsyeah... i figured it out.... thanks for your help

taahrqqhar
14,147 PointsI'm having the same problem No query results for model
```Route::get('/', 'TodoListController@index');
Route::get('todos', 'TodoListController@index');
Route::get('/todos/{id}', 'TodoListController@show');
Route::get('/todos/create', "TodoListController@create");```

krs dino
Courses Plus Student 778 Pointscheck you table if you have "created_at" and "updated_at" fields in you table, if you did not create those fields then add this to you model
<?php
public $timestamp = false;

taahrqqhar
14,147 Pointsyes I have the created_at and updated_at fields on the table but why isnt it working is driving me crazy 2 days stuck on this and i did everything right from the video...

krs dino
Courses Plus Student 778 PointsRoute::get('/', 'TodoListController@index');
Route::get('/todos', 'TodoListController@index');
Route::resource('/todos', 'TodoListController');
Route::get('/todos/{id}', 'TodoListController@show');
Route::get('/todos/create', "TodoListController@create");
Route::get('/db', function(){
return DB::table('name of your table')->get();
});
*NOTE: replace 'name of your table' with the actual name of your table.......replace all this in your routes and lets see if it fixes your issue

taahrqqhar
14,147 PointsI got it.. the problem was that I had Route::resource('/todos', 'TodoListController'); after Route::get('/todos/create', "TodoListController@create");
but once i put resource before /todos/create everything works perfectly
thomascawthorn
22,986 Pointsthomascawthorn
22,986 PointsHmm.. I think we'll need code from your create method. Can you find your todo list controller and paste JUST the method for create. like:
Can you also paste the route from routes.php that directing /todo/create to the todo list controller?