Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial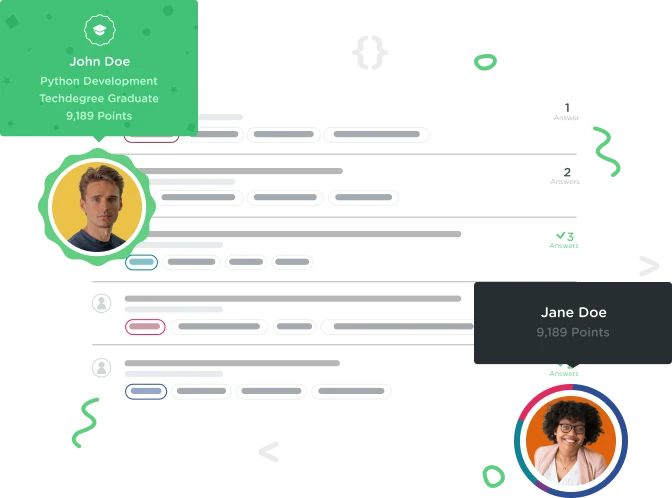

James Barshaw
9,284 Pointsno remove button still error fixed but have new errors
Uncaught ReferenceError: text is not defined at app.js:27 (anonymous) @ app.js:27 app.js:25 Uncaught ReferenceError: li is not defined at HTMLFormElement.form.addEventListener (app.js:25)
here is my code const form = document.getElementById('registrar'); const input = form.querySelector('input'); const ul = document.getElementById('invitedList');
function createLI(text) { const li = document.createElement('li') li.textContent = text; const label = document.createElement('label') label.textContent = 'confirmed'; const checkbox = document.createElelment ('input'); checkbox.type = 'checkbox'; label.appendChild(checkbox); li.appendChild(label); const button = document.createElement('button') button.textContent = 'remove'; li.appendChild(button); return li; }
form.addEventListener('submit', (e) =>{ e.preventDefault(); const text = input.value; input.value = '';
ul.appendChild(li); }); const li = createLI(text); ul.addEventListener ('change', (e) => { const checkbox = event.target; const checked = checkbox.checked; const listItem = checkbox.parentNode.parentNode;
if (checked) { listItem.className = 'responded'; } else { listItem.className = ''; } });
ul.addEventListener('click', (e) => { if (e.target.tagName === 'Button') { const li= e.target.parentNode; const ul= li.parentNode; } });
1 Answer
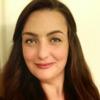
Jennifer Nordell
Treehouse TeacherHi there, James Barshaw ! It seems like you missed a line when following along. Inside your form.addEventListener
, you should have another line which calls the createLI
function and assigns the returned list item to a variable named li
, but because that line is missing, li
is undefined.
You wrote:
form.addEventListener('submit', (e) => {
e.preventDefault();
const text = input.value;
input.value = '';
ul.appendChild(li);
});
But that should be:
form.addEventListener('submit', (e) => {
e.preventDefault();
const text = input.value;
input.value = '';
const li = createLI(text); // it is this line that defines the local li variable
ul.appendChild(li);
});
You can see this at 6:16 of the video linked here.
Hope this helps!
benyoungblood
10,062 Pointsbenyoungblood
10,062 PointsI have formatted your code a little better to make it easier for us to help you. I've also added several semi-colons to the ends of your variable declarations.