Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial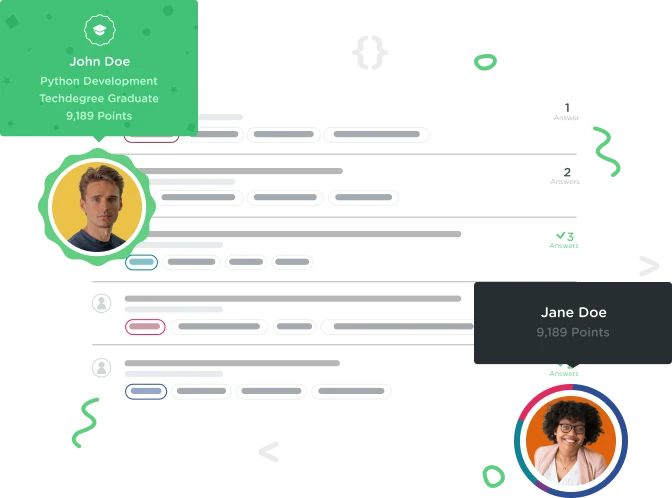
janeporter
Courses Plus Student 23,471 PointsNo route matches [GET] "/todo_lists/1/todo_items/9/complete" error
I'm getting a routing error as follows:
Routing Error
No route matches [GET] "/todo_lists/1/todo_items/9/complete"
Rails.root: C:/Users/jporter/Documents/ruby_projects_tth/odot
Application Trace | Framework Trace | Full Trace
Routes
Routes match in priority from top to bottom
Helper HTTP Verb Path Controller#Action
Path / Url
Path Match
complete_todo_list_todo_item_path PATCH /todo_lists/:todo_list_id/todo_items/:id/complete(.:format) todo_items#complete
todo_list_todo_items_path GET /todo_lists/:todo_list_id/todo_items(.:format) todo_items#index
POST /todo_lists/:todo_list_id/todo_items(.:format) todo_items#create
new_todo_list_todo_item_path GET /todo_lists/:todo_list_id/todo_items/new(.:format) todo_items#new
edit_todo_list_todo_item_path GET /todo_lists/:todo_list_id/todo_items/:id/edit(.:format) todo_items#edit
todo_list_todo_item_path GET /todo_lists/:todo_list_id/todo_items/:id(.:format) todo_items#show
PATCH /todo_lists/:todo_list_id/todo_items/:id(.:format) todo_items#update
PUT /todo_lists/:todo_list_id/todo_items/:id(.:format) todo_items#update
DELETE /todo_lists/:todo_list_id/todo_items/:id(.:format) todo_items#destroy
todo_lists_path GET /todo_lists(.:format) todo_lists#index
POST /todo_lists(.:format) todo_lists#create
new_todo_list_path GET /todo_lists/new(.:format) todo_lists#new
edit_todo_list_path GET /todo_lists/:id/edit(.:format) todo_lists#edit
todo_list_path GET /todo_lists/:id(.:format) todo_lists#show
PATCH /todo_lists/:id(.:format) todo_lists#update
PUT /todo_lists/:id(.:format) todo_lists#update
DELETE /todo_lists/:id(.:format) todo_lists#destroy
root_path GET / todo_lists#index
Request
Parameters:
None
Toggle session dump
Toggle env dump
Response
Headers:
None
x
>>
here is my route.rb file:
Rails.application.routes.draw do
resources :todo_lists do
resources :todo_items do
member do
patch :complete
end
end
end
root 'todo_lists#index'
# The priority is based upon order of creation: first created -> highest priority.
# See how all your routes lay out with "rake routes".
# You can have the root of your site routed with "root"
# root 'welcome#index'
# Example of regular route:
# get 'products/:id' => 'catalog#view'
# Example of named route that can be invoked with purchase_url(id: product.id)
# get 'products/:id/purchase' => 'catalog#purchase', as: :purchase
# Example resource route (maps HTTP verbs to controller actions automatically):
# resources :products
# Example resource route with options:
# resources :products do
# member do
# get 'short'
# post 'toggle'
# end
#
# collection do
# get 'sold'
# end
# end
# Example resource route with sub-resources:
# resources :products do
# resources :comments, :sales
# resource :seller
# end
# Example resource route with more complex sub-resources:
# resources :products do
# resources :comments
# resources :sales do
# get 'recent', on: :collection
# end
# end
# Example resource route with concerns:
# concern :toggleable do
# post 'toggle'
# end
# resources :posts, concerns: :toggleable
# resources :photos, concerns: :toggleable
# Example resource route within a namespace:
# namespace :admin do
# # Directs /admin/products/* to Admin::ProductsController
# # (app/controllers/admin/products_controller.rb)
# resources :products
# end
end
here is my complete_spec.rb file:
require 'spec_helper'
describe "Completing todo items" do
let!(:todo_list) { TodoList.create(title: "Grocery List", description: "Groceries") }
let!(:todo_item) { todo_list.todo_items.create(content: "Milk") }
it "is successful whem marking a single item complete" do
expect(todo_item.completed_at).to be_nil
visit_todo_list todo_list
within dom_id_for(todo_item) do
click_link "Mark Complete"
end
todo_item.reload
expect(todo_item.completed_at).to_not be_nil
end
end
here is my index.html.erb file:
<h1><%= @todo_list.title %></h1>
<table class="todo_items">
<% @todo_list.todo_items.each do |todo_item| %>
<tr id="<%= dom_id(todo_item) %>">
<td><%= todo_item.content %></td>
<td>
<%= link_to "Mark Complete", complete_todo_list_todo_item_path(todo_item), method: :patch %>
<%= link_to "Edit", edit_todo_list_todo_item_path(todo_item) %>
<%= button_to "Destroy", todo_list_todo_item_path(todo_item), method: :delete, data: { confirm: "Are you sure?" } %>
</td>
</tr>
<% end %>
</table>
<p>
<%= link_to "New Todo Item", new_todo_list_todo_item_path %>
</p>
and here is the complete controller in my todo_items_controller.rb file:
def complete
@todo_item = @todo_list.todo_items.find(params[:id])
@todo_item.update_attribute(:completed_at, Time.now)
redirect_to todo_list_todo_items_path, notice: "Todo item marked as complete."
end
Any idea what could be happening? FYI: i'm on windows 10 pro, using Rails 4.2.5, on a Dell xps 9550 laptop.