Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial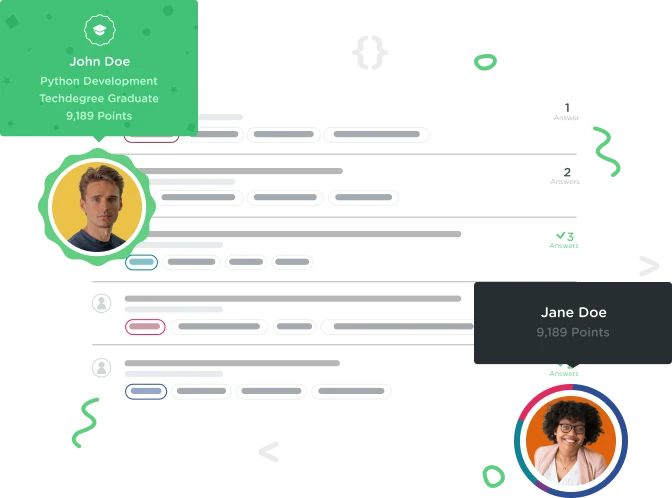
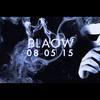
Jill Marcum
16,088 PointsNo split method in video
Hi – just FYI, in Methods 3, Jim mentions that the join method is similar to the split method, but split is never covered in any of the javascript videos.
2 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsThe split method in its basic form is not really that complicated. It splits a string into an array based on the separator parameter passed to the method.
What does that mean? And why would you use it?
Here's a simple example. Let's say you're working on a text editing app and you want to display the word count on the client side, dynamically, as people are typing.
The most basic way of displaying word count would be splitting the text people have written, and using a space (" "
) as the separator.
This is what would happen:
var text = "This is some text.";
var textArr = text.split(" "); // textArr now contains the array: ["This", "is", "some", "text."]
To display the word count, all you'd need to do is check the length property of the newly created array.
The split method will go through a string, looking for the separator that you've passed. When it encounters it, it will grab all the characters up to that separator, create another string from those character and push it into an array. Then it will keep looking for the next occurrence of the separator and repeat those steps. When it reaches the end of the string, it will add the leftover characters as the last element of the array and then it's going to return that array.
If it doesn't find the separator anywhere in the string, it will just return an array with a single element inside — the complete string the method was called on (the same things happens if you don't pass the separator parameter to the method):
var text = "Some text";
var textArr = text.split("!!!"); // ["Some text"];
If the separator is an empty string (""
), the string will be split into separate characters:
var text = "Some text";
var textArr = text.split(""); // ["S", "o", "m", "e", " ", "t", "e", "x", "t"]
The separator itself will never appear in the returned array. It's only used to pinpoint the location where splitting occurs, but after that it's removed from the text that's being split.
This is the most basic functionality. Additionally, the split method accepts a second optional parameter — limit. The method will still go through all of the string and create an array, but the array itself will be truncated so that its number of elements doesn't exceed the limit.
The separator parameter can also be a regular expression, so things can get a bit complicated here. I'm not going to get into that as regular expressions are an area of their own, but it's important to note that it's possible.
Are there any other reasons why you'd want to use split?
Sure. Maybe your web app allows the users to tag their posts. If you had an input field for those tags, you could split the list with comma as the separator, trim the whitespace of the elements and get an array of the tags relevant to the post.
Or maybe you have a database column that contains numerous entries in one field, formatted with an agreed-upon delimiter (e.g. entries in an interests column for user profiles could look like this: "dancing|writing|playing guitar|swimming"
). Again, you could use the split method with the vertical bar character (pipe |
) to get those into an array and do something with them. (Please note that this isn't a good database design decision, but sometimes things like that happen, or you don't have any say in the matter.)
I know this isn't a video about the split method, but hopefully it provides an intro to what you can do with it and how you can use it. It's also a good idea to check the MDN documentation on split.
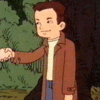
Kiran Matthews
138 PointsWould love it if y'all could post a video covering Split - it's a real head scratcher!