Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial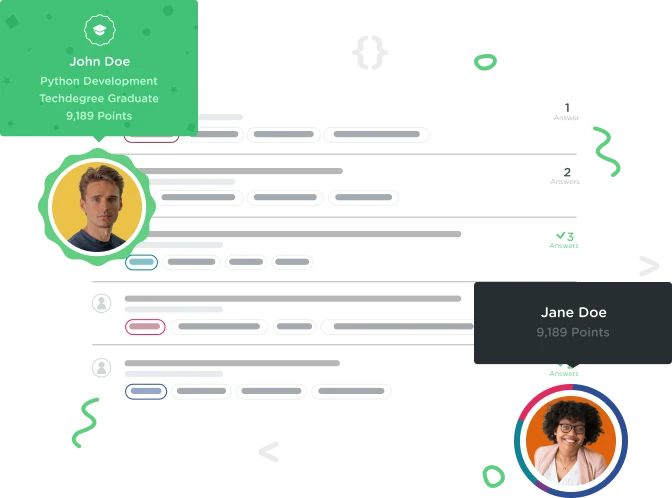

Dearce Goodman
2,331 PointsNo sure where to go
I feel like I'm close, just not sure what I'm missing.
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char letter){
int counter = 0;
for( char enteredLetter : tiles.toCharArray()){
if(tiles.indexOf(letter) != -1){
counter++;
}
}
return counter;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
1 Answer

daniel104729
7,176 PointsFirst, please ask a question explaining the issue and try to explain what you have tried. I am assuming that you are trying to implement getCountOfLetter
. There is an issue with your if
statement. You should be comparing the value of letter
and enteredLetter
, right now I am guessing it either returns 0 or the length of titles
. Hope this helps