Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial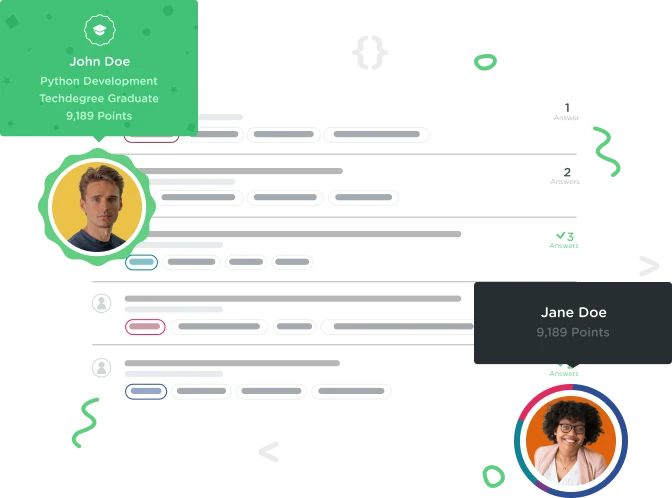
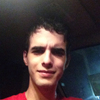
Leonardo Cavalcanti
5,308 PointsNo TEXT data shown using sqlite3
I made this code based off the one taught in the videos.
from peewee import *
import datetime
import sys
db = SqliteDatabase('diary.db')
class Diary(Model):
entries = TextField()
date = DateTimeField(default = datetime.datetime.now)
class Meta:
database = db
def initialize():
"""initializes the database"""
db.connect()
db.create_tables([Diary], safe = True)
#end of initialize
def menu_loop():
"""show menu"""
choice = 0
while choice != 2:
print("Enter '2' to quit")
print('1) to add an entry')
choice = input()
if choice == 1:
add_entry()
#end of menu_loop
def add_entry():
"""add an entry"""
print("Enter your entry, press ctrl+d when done")
data = raw_input().strip()
if data:
while(True):
option = raw_input('\nSave entry?[Y/n] ').lower()
if option == 'y':
Diary.create(content=data)
print ("Saved sucessfully!")
break
if option == 'n':
print ("exited")
break
#end of add_entry
if __name__ == '__main__':
initialize()
menu_loop()
Using Sqlite3 through the terminal I get :
sqlite>
Basically everything seems to be working perfectly, except when I try to look into the TEXT entries, it won't show anything as if the text date isn't being stored.
1 Answer

Seth Kroger
56,413 PointsWhen you create the new diary entry in add_entry you're using the wrong name for the member field. Diary.create(content=data)
should be Diary.create(entries=data)