Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial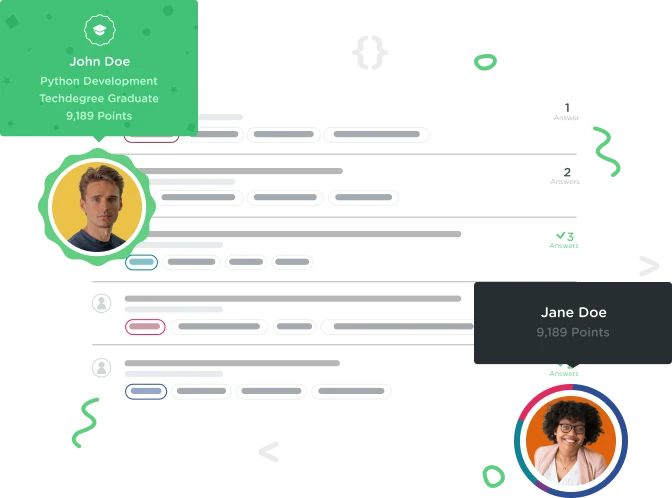

daviddavis3
4,904 PointsNo weather data at this step
My Stormy app seems to be running OK, and my emulator has "Hello world!". However, I am not getting any weather data after starting the app. What could be the matter with this?
Do I have to be logged in to my forecast account?
8 Answers

daviddavis3
4,904 PointsI believe clearing the cache and restarting the emulator seemed to help the best. I am still uncertain what the EGL errors were.

Douglas Rodriguez
23,577 PointsI had the same issue for the weather data, it would not show up. I fixed it by placing a log call on the onFailure method to see if it was failing there.
public void onFailure(Request request, IOException e) {
Log.d(TAG, e.getMessage());
}
I ran the app and checked the log to see if it threw an error and it did: "Unable to resolve host "api.forecast.io": No address associated with hostname" The hostname was correct so I imagined it was a problem with the connection so I check the Genymotion emulator to see if it had connection and it didn't. I connected it to the WiFi it had available and it worked afterwards. Also my data did not display like it was in the video, mine was all in one line continuously.
Also I believe the other error you mentioned is common, it always comes out in my logs: "Failed to set EGL_SWAP_BEHAVIOR on surface 0xa2431ce0, error=EGL_SUCCESS"

Wesley Seago
10,424 PointsPlease post your code.
You do not have to be logged in to your API account, but you do need to confirm that your account is setup.

daviddavis3
4,904 Pointspackage com.example.postadavis.stormy;
import android.app.DownloadManager;
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.widget.Toast;
import com.squareup.okhttp.Call;
import com.squareup.okhttp.Callback;
import com.squareup.okhttp.OkHttpClient;
import com.squareup.okhttp.Request;
import com.squareup.okhttp.Response;
import java.io.IOException;
public class MainActivity extends ActionBarActivity {
public static final String TAG = MainActivity.class.getSimpleName();
private boolean mNetworkAvailable;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apikey = "404c6a2f41acf9214599bf7c6c757656";
double laditude = 37.8267;
double longitude = -122.423;
String forecastUrl = "https://api.forecast.io/forecast/" + apikey +
"/" + laditude + "," + longitude;
if (isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
This is the top part of my code.
My logcat log doesn't show weather data when I run the app. So far, I can follow along the Weather App sequence halfway with no error in my emulator. But I am getting to the part (Working with JSON) that does something with the weather data feed. Since I have no feed, "I'm up a creek" with no stream.

daviddavis3
4,904 PointsStupid mistake #1: I misspelled latitude.
After corrections, I still get an error in logcat: "W/OpenGLRenderer﹕ Failed to set EGL_SWAP_BEHAVIOR on surface 0xa47192a0, error=EGL_SUCCESS".

daviddavis3
4,904 Points<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.postadavis.stormy" >
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
The above code is my AndroidManifest.xml file. It differs from Ben's code in the package and the Activity names. Maybe something happened that the IDE didn't create the manifest file correctly. I could go back and manually edit the file to try to get it to run. I am getting some weather data, but it also is accompanied with a lot of red error text in the logcat.
01-24 01:35:39.171 1751-1771/com.example.postadavis.stormy W/EGL_emulation﹕ eglSurfaceAttrib not implemented
01-24 01:35:39.171 1751-1771/com.example.postadavis.stormy W/OpenGLRenderer﹕ Failed to set EGL_SWAP_BEHAVIOR on surface 0xa4519200, error=EGL_SUCCESS
I tried running it with both the greymotion and the default emulators.
With the default emulator, I get the logcat below, starting it up. I do not get the weather data. I think I did get weather data with the greymotion emulator, but with lots of error data.
01-23 20:39:21.810 919-919/com.example.postadavis.stormy D/dalvikvm﹕ Not late-enabling CheckJNI (already on)
01-23 20:39:22.670 919-919/com.example.postadavis.stormy I/dalvikvm﹕ Could not find method android.view.ViewGroup.onNestedScrollAccepted, referenced from method android.support.v7.internal.widget.ActionBarOverlayLayout.onNestedScrollAccepted
01-23 20:39:22.670 919-919/com.example.postadavis.stormy W/dalvikvm﹕ VFY: unable to resolve virtual method 11348: Landroid/view/ViewGroup;.onNestedScrollAccepted (Landroid/view/View;Landroid/view/View;I)V
01-23 20:39:22.670 919-919/com.example.postadavis.stormy D/dalvikvm﹕ VFY: replacing opcode 0x6f at 0x0000
01-23 20:39:22.670 919-919/com.example.postadavis.stormy I/dalvikvm﹕ Could not find method android.view.ViewGroup.onStopNestedScroll, referenced from method android.support.v7.internal.widget.ActionBarOverlayLayout.onStopNestedScroll
01-23 20:39:22.670 919-919/com.example.postadavis.stormy W/dalvikvm﹕ VFY: unable to resolve virtual method 11354: Landroid/view/ViewGroup;.onStopNestedScroll (Landroid/view/View;)V
01-23 20:39:22.670 919-919/com.example.postadavis.stormy D/dalvikvm﹕ VFY: replacing opcode 0x6f at 0x0000
01-23 20:39:22.730 919-919/com.example.postadavis.stormy I/dalvikvm﹕ Could not find method android.support.v7.internal.widget.ActionBarOverlayLayout.stopNestedScroll, referenced from method android.support.v7.internal.widget.ActionBarOverlayLayout.setHideOnContentScrollEnabled
01-23 20:39:22.730 919-919/com.example.postadavis.stormy W/dalvikvm﹕ VFY: unable to resolve virtual method 9042: Landroid/support/v7/internal/widget/ActionBarOverlayLayout;.stopNestedScroll ()V
01-23 20:39:22.740 919-919/com.example.postadavis.stormy D/dalvikvm﹕ VFY: replacing opcode 0x6e at 0x000e
01-23 20:39:22.780 919-919/com.example.postadavis.stormy I/dalvikvm﹕ Could not find method android.content.res.TypedArray.getChangingConfigurations, referenced from method android.support.v7.internal.widget.TintTypedArray.getChangingConfigurations
01-23 20:39:22.780 919-919/com.example.postadavis.stormy W/dalvikvm﹕ VFY: unable to resolve virtual method 367: Landroid/content/res/TypedArray;.getChangingConfigurations ()I
01-23 20:39:22.780 919-919/com.example.postadavis.stormy D/dalvikvm﹕ VFY: replacing opcode 0x6e at 0x0002
01-23 20:39:22.840 919-919/com.example.postadavis.stormy I/dalvikvm﹕ Could not find method android.content.res.TypedArray.getType, referenced from method android.support.v7.internal.widget.TintTypedArray.getType
01-23 20:39:22.840 919-919/com.example.postadavis.stormy W/dalvikvm﹕ VFY: unable to resolve virtual method 389: Landroid/content/res/TypedArray;.getType (I)I
01-23 20:39:22.840 919-919/com.example.postadavis.stormy D/dalvikvm﹕ VFY: replacing opcode 0x6e at 0x0002
01-23 20:39:23.490 919-919/com.example.postadavis.stormy D/dalvikvm﹕ GC_FOR_ALLOC freed 230K, 13% free 3442K/3956K, paused 28ms, total 31ms
01-23 20:39:23.500 919-919/com.example.postadavis.stormy D/MainActivity﹕ Main UI code is running!
01-23 20:39:24.140 919-932/com.example.postadavis.stormy W/dalvikvm﹕ VFY: unable to find class referenced in signature (Ljava/nio/file/Path;)
01-23 20:39:24.150 919-932/com.example.postadavis.stormy W/dalvikvm﹕ VFY: unable to find class referenced in signature ([Ljava/nio/file/OpenOption;)
01-23 20:39:24.150 919-932/com.example.postadavis.stormy I/dalvikvm﹕ Could not find method java.nio.file.Files.newOutputStream, referenced from method okio.Okio.sink
01-23 20:39:24.150 919-932/com.example.postadavis.stormy W/dalvikvm﹕ VFY: unable to resolve static method 13821: Ljava/nio/file/Files;.newOutputStream (Ljava/nio/file/Path;[Ljava/nio/file/OpenOption;)Ljava/io/OutputStream;
01-23 20:39:24.150 919-932/com.example.postadavis.stormy D/dalvikvm﹕ VFY: replacing opcode 0x71 at 0x000a
01-23 20:39:24.160 919-932/com.example.postadavis.stormy W/dalvikvm﹕ VFY: unable to find class referenced in signature (Ljava/nio/file/Path;)
01-23 20:39:24.160 919-932/com.example.postadavis.stormy W/dalvikvm﹕ VFY: unable to find class referenced in signature ([Ljava/nio/file/OpenOption;)
01-23 20:39:24.160 919-932/com.example.postadavis.stormy I/dalvikvm﹕ Could not find method java.nio.file.Files.newInputStream, referenced from method okio.Okio.source
01-23 20:39:24.160 919-932/com.example.postadavis.stormy W/dalvikvm﹕ VFY: unable to resolve static method 13820: Ljava/nio/file/Files;.newInputStream (Ljava/nio/file/Path;[Ljava/nio/file/OpenOption;)Ljava/io/InputStream;
01-23 20:39:24.160 919-932/com.example.postadavis.stormy D/dalvikvm﹕ VFY: replacing opcode 0x71 at 0x000a
01-23 20:39:24.350 919-919/com.example.postadavis.stormy D/﹕ HostConnection::get() New Host Connection established 0xb73f7f88, tid 919
01-23 20:39:24.480 919-932/com.example.postadavis.stormy D/dalvikvm﹕ GC_FOR_ALLOC freed 334K, 15% free 3580K/4204K, paused 21ms, total 22ms
01-23 20:39:24.570 919-919/com.example.postadavis.stormy W/EGL_emulation﹕ eglSurfaceAttrib not implemented
01-23 20:39:24.580 919-919/com.example.postadavis.stormy D/OpenGLRenderer﹕ Enabling debug mode 0
With the greymotion emulator, I get the logcat error below:
I/art﹕ Late-enabling -Xcheck:jni
D/MainActivity﹕ Main UI code is running!
D/OpenGLRenderer﹕ Render dirty regions requested: true
D/﹕ HostConnection::get() New Host Connection established 0xacf88c60, tid 1520
D/Atlas﹕ Validating map...
D/libEGL﹕ loaded /system/lib/egl/libEGL_emulation.so
D/libEGL﹕ loaded /system/lib/egl/libGLESv1_CM_emulation.so
D/libEGL﹕ loaded /system/lib/egl/libGLESv2_emulation.so
D/﹕ HostConnection::get() New Host Connection established 0xa4516120, tid 1541
I/OpenGLRenderer﹕ Initialized EGL, version 1.4
D/OpenGLRenderer﹕ Enabling debug mode 0
W/EGL_emulation﹕ eglSurfaceAttrib not implemented
W/OpenGLRenderer﹕ Failed to set EGL_SWAP_BEHAVIOR on surface 0xa4519200, error=EGL_SUCCESS

Slava Fleer
6,086 PointsI found too that with all emulators with api 21 the screen stays black with this logcat:
but works fine on api 19.

maivandahmadzai
3,467 PointsIs there any fix for this? the code mor or less looks the same on OkHttp:s website eventho the new version is 2.5
maivandahmadzai
3,467 Pointsmaivandahmadzai
3,467 PointsI still have the same problem, Restarting did not help neither did changing from api 21 to 19 just getting another error but no data from the Forecast.io