Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial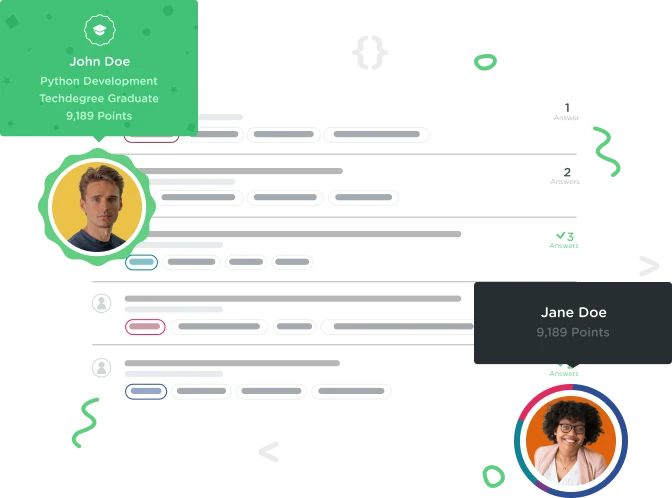

paulscanlon
Courses Plus Student 26,735 PointsNode Js Extra Credit
Hi guys.
Just wondering what you think of my Weather app for the Node Js basics course. It retrieves an address from Google geolocation API, converts it to latitude and longitude, then retrieves the current weather for that location.
I know it's a lot to ask to go over my work but this is my first difficult project and any comments/criticisms are more than welcome.
Thanks in advance
Happy coding
Paul
paulscanlon
Courses Plus Student 26,735 Pointspaulscanlon
Courses Plus Student 26,735 Points