Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial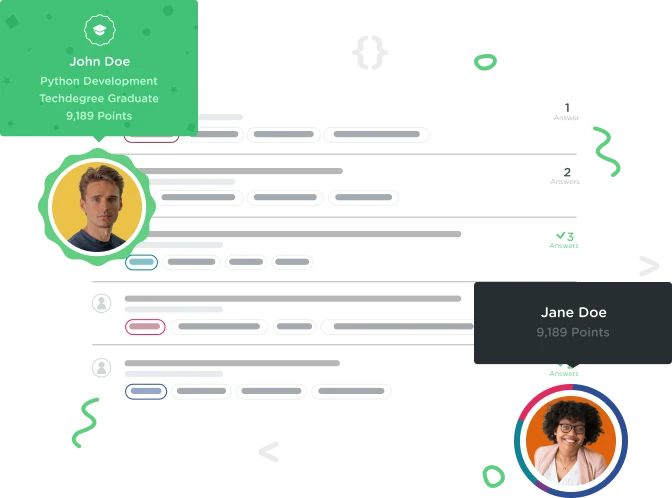
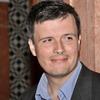
Brian Patterson
19,588 Pointsnode not returning anything.
I am trying to sharpen my node.js skill by using a different api. The api I am using is the following: http://ergast.com/api/f1/2008/results.json
Here is my code.
const https = require('https');
const http = require('http');
const season = 2008;
function printMessage(Results, season) {
const message = `There are ${Results} rounds at the ${season}`;
console.log(message);
}
function get(season) {
const request = http.get(`http://ergast.com/api/f1/${season}/results.json`, response => {
let body = "";
response.on('data', chunk => {
body += chunk;
});
response.on('end', () => {
const f1Season = JSON.parse(body);
console.dir(f1Season);
})
});
}
Now the file is called seasonF1.js and when I put that into the console nothing is returned.. Can anyone help me as to why this has happened?
1 Answer

Alexander La Bianca
15,959 PointsIt looks like you are not running the get function.
just do get(season) and then it works for me.
Also, a small tip on improving this and not hardcoding the 2008 season into your code. You can accept it as a command line argument like so:
const https = require('https');
const http = require('http');
function printMessage(Results, season) {
const message = `There are ${Results} rounds at the ${season}`;
console.log(message);
}
function get(season) {
const request = http.get(`http://ergast.com/api/f1/${season}/results.json`, response => {
let body = "";
response.on('data', chunk => {
body += chunk;
});
response.on('end', () => {
const f1Season = JSON.parse(body);
console.dir(f1Season);
})
});
}
//accept season as a command line argument
const season = process.argv[2];
get(season)
//when you run the program you can do in the command line
// node <nameOfFile> 2008
// node <nameOfFile> 2009
// and so on
Brian Patterson
19,588 PointsBrian Patterson
19,588 PointsThanks will try this when I get home.