Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial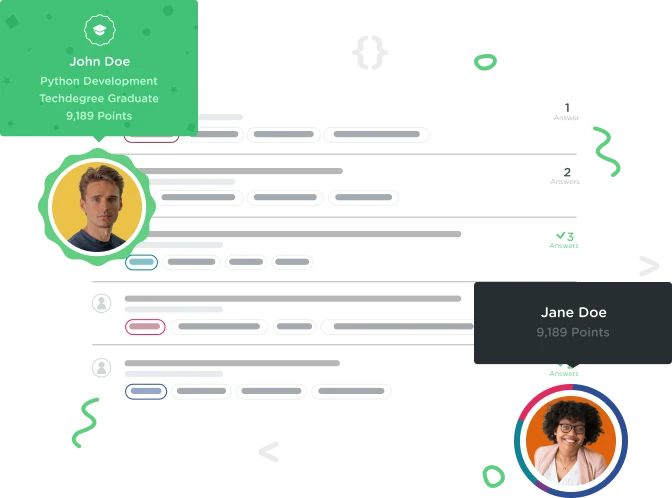
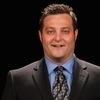
Jeffrey Davidson
26,147 PointsNodejs Basics - Profile (Moved Permanately)
I'm trying to figure out with the my code why I would be getting the following error when I run node in the console.
There was an error getting the profile for jeffreydavidson. (Moved Permanently)
My profile json response works just fine.
https://teamtreehouse.com/jeffreydavidson.json
//Problem: We need a simple way to look at a user's badge count and Javascript points
//Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
var http = require("http");
var username = "jeffreydavidson";
//Print out message
function printMessage(username, badgeCount, points) {
var message = username + " has " + badgeCount + " total badge(s) and " + points + " points in Javascript";
console.log(message);
}
//Print out error messages
function printError(error) {
console.error(error.message);
}
//Connect to API URL (https://teamtreehouse.com/username.json)
var request = http.get("http://teamtreehouse.com/" + username + ".json", function(response) {
var body = "";
//Read the data
response.on('data', function(chunk) {
body += chunk;
});
response.on('end', function() {
if(response.statusCode == 200){
try {
var profile = JSON.parse(body);
printMessage(username, profile.badges.length, profile.points.Javascript);
} catch(error) {
//Parse Error
printError(error);
}
} else {
//Status Code Error
printError({message: "There was an error getting the profile for " + username +". (" + http.STATUS_CODES[response.statusCode] + ")"});
}
});
//Parse the data
//Print the data
});
//Connection Error
request.on('error', printError);
1 Answer
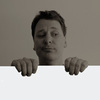
Sean T. Unwin
28,690 Points//Replace
var http = require("http");
// with
var https = require("https");
-- Add an 's' to 'http'. Then update all references to the http
variable to https
. Such as in the var request
line.
As to the reason why, please see the Teacher's Notes for the lesson - Making a GET Request with http
Jeffrey Davidson
26,147 PointsJeffrey Davidson
26,147 PointsI guess I'm not understanding because the code is the same as the video so it should work.
Sean T. Unwin
28,690 PointsSean T. Unwin
28,690 PointsIt is because teamtreehouse.com switched to SSL so all URIs go through HTTPS now instead of HTTP. You can see this in the address bar of your browser on this page.
The video was shot before this change was made to teamtreehouse.com, which is why the issue is updated in the Teacher's Notes. Read the Teacher's Notes in the link given above for more information.
ProTip: Before starting each lesson always check the Teacher's Notes if there are any.