Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial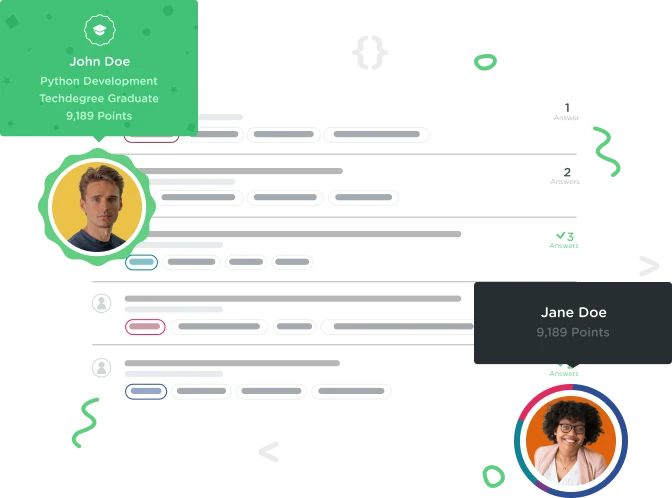
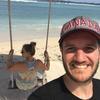
Tobias Graefe
11,934 PointsNodeJs modules and return values
I wrote a function that reads out all train stops in the city I live in from an API. I used the nodeJS https object. That works great. Now I want to transfer the data to my app.js file. I used "module.exports.getStopData = getStopData" and require in app.js. That works out great, but I don't get, how I can return data from my request.on("end") function.
stops.js
const https = require('https');
function getStopData(stopName){
var request = https.get('https://data.webservice-kvb.koeln/service/opendata/haltestellen/json', response => {
if(response.statusCode === 200){
var body = "";
response.on("data", data => {
body += data.toString();
});
response.on("end", ()=>{
let kvbData = JSON.parse(body).features;
let stops = [];
for(let stop in kvbData){
if(kvbData[stop].properties.Name === stopName){
stops.push(kvbData[stop]);
}
}
return stops;
});
}
});
}
module.exports.getStopData = getStopData;
app.js
const stop = require('./stop');
const name = 'Heumarkt'
stop.getStopData(name);
Thanks everyone.
1 Answer
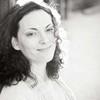
Alexandra Silcox
24,486 PointsYou can do a couple things to return the data
1) Return stops in a callback function
stops.js
function getStopData(stopName, someCallBack){
var request = https.get('https://data.webservice-kvb.koeln/service/opendata/haltestellen/json', response => {
if(response.statusCode === 200){
var body = "";
response.on("data", data => {
body += data.toString();
});
response.on("end", ()=>{
let kvbData = JSON.parse(body).features;
let stops = [];
for(let stop in kvbData){
if(kvbData[stop].properties.Name === stopName){
stops.push(kvbData[stop]);
}
}
someCallBack(stops);
});
}
});
}
app.js
const stop = require('./stops');
const name = 'Heumarkt'
stop.getStopData(name, () => console.log(res));
2. Return stops with a promise-based wrapper
stops.js
function getStopData(stopName){
return new Promise(resolve => {
var request = https.get('https://data.webservice-kvb.koeln/service/opendata/haltestellen/json', response => {
if(response.statusCode === 200){
var body = "";
response.on("data", data => {
body += data.toString();
});
response.on("end", ()=>{
let kvbData = JSON.parse(body).features;
let stops = [];
for(let stop in kvbData){
if(kvbData[stop].properties.Name === stopName){
stops.push(kvbData[stop]);
}
}
resolve(stops);
});
}
});
});
}
app.js
const stop = require('./stops');
const name = 'Heumarkt'
stop.getStopData(name).then(res => {
console.log(res)
);