Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial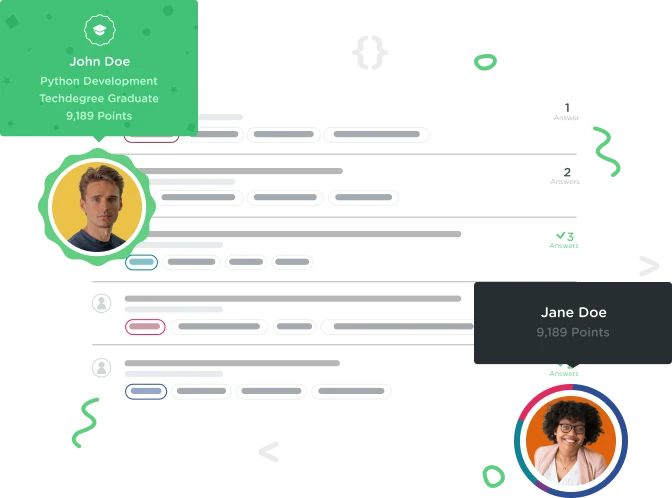
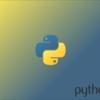
Kevin Faust
15,353 Pointsnode.js try-catch block placement question
it makes more sense for me to use the first block of code. if we dont have a 200 status code, then dont run anything and just end the code. the instructor used the second block of code. which code would be better?
var request = https.get('https://teamtreehouse.com/' + username + '.json', function(response) {
if (response.statusCode === 200) {
var body = "";
response.on('data', function(chunk) {
body += chunk;
});
response.on('end', function() {
try {
var profile = JSON.parse(body);
printMessage(profile.name, profile.badges.length, profile.points.JavaScript);
} catch (e) {
printError(e);
}
});
} else {
//error
}
});
var request = https.get('https://teamtreehouse.com/' + username + '.json', function(response) {
var body = "";
response.on('data', function(chunk) {
body += chunk;
});
response.on('end', function() {
if (response.statusCode === 200) {
try {
var profile = JSON.parse(body);
printMessage(profile.name, profile.badges.length, profile.points.JavaScript);
} catch (e) {
printError(e);
}
} else {
//error
}
});
});
1 Answer
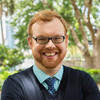
Andrew Chalkley
Treehouse Guest TeacherHey Kevin Faust!
Great question. I did the exact same thing when I was developing this project. In the version of Node.js I was working on at the time it was timing out. The end
event wasn't handle. Node.js' event loop would hang until it was handled. You'd have to trigger the end
event manually in an undocumented method call to trigger the end.
The other way was to implement the end
. Since this course was released Node has been through several versions and I've upgraded to the latest long term support of Node.js in Workspaces, so your code should work now. It looks like they've fixed that issue so it doesn't timeout and hang and end
event handler can be avoided by the conditional code in the first example.
This would have been a massive technical tangent to explain but now it's seems to be resolved in the latest Node.js versions.
So yeah, use the first code if you want, just be super careful when using it in older version of Node.js.
Hope that helps!
Andrew
Kevin Faust
15,353 PointsKevin Faust
15,353 Pointshi andrew thanks. so basically in older versions of node, it needed that 'end' handler/thing otherwise the program would hang. and thats why you took the approach of the second code block?
is one code block faster than the other? if theyre both the same speed then i would use the second code block just for the extra support. but then again i only like to use new things/versions
Andrew Chalkley
Treehouse Guest TeacherAndrew Chalkley
Treehouse Guest TeacherThat's exactly right.
Since it doesn't hang now I'd assume the first way would be slightly faster, but I don't know what it does to the connection, is it still hanging around because you haven't streamed the data or does the V8 engine or Node.js APIs do some code analysis and close the connection knowing it'll never be executed? What ever the answer is it looks like it's been optimized now since it doesn't hang and successfully falls through. I'd go with the first approach.