Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial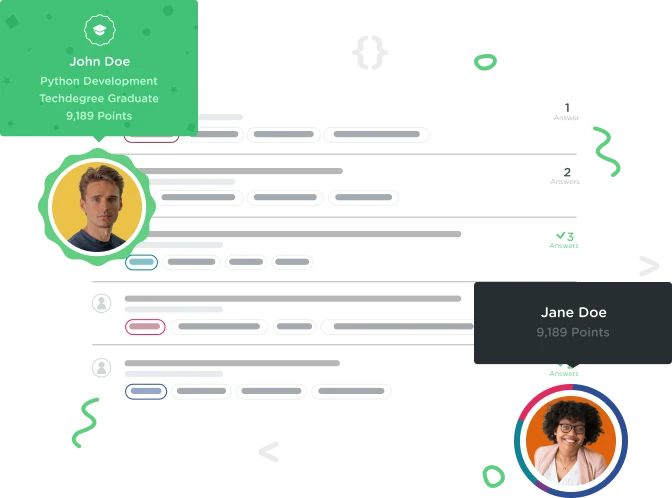

mm4
8,600 PointsNoMethodError: undefined method 'each' for #<BankAccount:0x007ff7db82fd40>
I have tried debugging for an hour to no avail.
class BankAccount
def self.create_for(firstname, lastname)
@accounts ||= []
@accounts = BankAccount.new(firstname, lastname)
end
def self.find_for(first_name, last_name)
@accounts.find{|account| account.full_name == "#{first_name} #{last_name}"}
end
def initialize(firstname, lastname)
@balance = 0
@firstname = firstname
@lastname = lastname
end
def full_name
"#{@firstname} #{@lastname}"
end
def deposit(amount)
@balance += amount
end
def withdraw(amount)
@balance -= amount
end
end
And this is my error:
irb(main):045:0> bankaccount = BankAccount.find_for("J","R")
NoMethodError: undefined method `each' for #<BankAccount:0x007ff7db82fd40>
from /Users/Macbook/hello.rb:13:in `find'
from /Users/Macbook/hello.rb:13:in `find_for'
from (irb):45
from /Users/Macbook/.rbenv/versions/2.1.5/bin/irb:11:in `<main>'
1 Answer
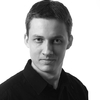
Maciej Czuchnowski
36,441 PointsPlease rewatch the video, especially 2:28 and make sure your code is correct, especially the self.create_for method with << operator.
mm4
8,600 Pointsmm4
8,600 PointsActually that's what I've been playing around with. When I originally had that, it said:
irb(main):016:0> BankAccount.create_for("D","S")
NoMethodError: undefined method `<<' for #<BankAccount:0x007fb181868398>
I thought << was supposed to be already defined in Ruby...
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 Points<< is defined in Ruby, it allows inserting elements into arrays and hashes.
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsI tried your code, it works fine with << operator.
mm4
8,600 Pointsmm4
8,600 PointsOkay, thanks! That's really odd. Not sure why my terminal is saying such things but I'll look into it...
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsI used your code in the terminal by running `
ruby bank.rb
(I saved it with that name locally). I did not run it in IRB. But in IRB you would have to load it first, like Jason did, then run BankAccount.create_for("J","R") and then you can use bankaccount = BankAccount.find_for("J","R") command.mm4
8,600 Pointsmm4
8,600 PointsHi Maciej,
I think I just had to quit the terminal and restart it because once I did that, I got it to work. Maybe I had typed something weird and forgotten about it, and that's why it wasn't working properly, but it's good now! Thanks for the help!