Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial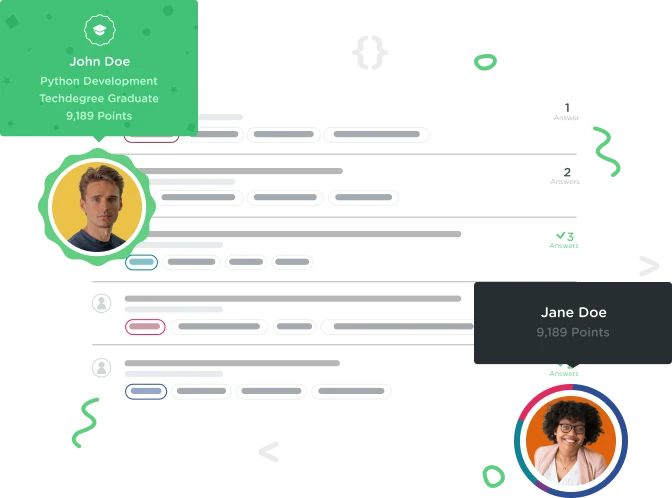

Michael Bragg
811 PointsNon-Compilation
My code compiles in Visual Studio, but I get bummer here
using System;
namespace Temerature { class Program { static void Main() { string input = Console.ReadLine(); int temperature = int.Parse(input);
if (temperature < 21)
{
System.Console.WriteLine("Too cold!");
}
else if (temperature >= 21 && temperature < 22)
{
System.Console.WriteLine("Just right.");
}
else
{
System.Console.WriteLine("Too hot!");
}
}
}
}
using System;
namespace Temerature
{
class Program
{
static void Main()
{
string input = Console.ReadLine();
int temperature = int.Parse(input);
if (temperature < 21)
{
System.Console.WriteLine("Too cold!");
}
else if (temperature >= 21 && temperature < 22)
{
System.Console.WriteLine("Just right.");
}
else
{
System.Console.WriteLine("Too hot!");
}
}
}
}
14 Answers
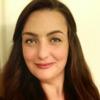
Jennifer Nordell
Treehouse TeacherHi there, Michael Bragg! First, and foremost, these challenges generally require that you not do anything they haven't asked for. In your code, you've included all the code that was necessary to compile your program. This includes the directive to use System
, a namespace, and even a main
function. That is already set up for you in the challenge. All it wants you to do is enter the small code snippet it's asking for. The code it's looking for is between the line with string input
and the end of the else
statement.
Once you put that code into the challenge, you will still get a Bummer! message. There's a small logic flaw in else if
statement. It should print out "Just right." if the temperature is 22.
Hope this helps!

Michael Bragg
811 Pointsstring input = Console.ReadLine();
int temperature = int.Parse(input);
if("temperature < 21)
{
System.Console.WriteLine("Too cold!");
}
else if("temperature == 22);
{
System.Console.WriteLine("Just right!");
}
else
{
System.Consolte.WriteLine("Too hot!");
}
Still get the bummer error
Moderator edited: Markdown added so that code renders properly in the forums.

Michael Bragg
811 Pointsstring input = Console.ReadLine();
int temperature = int.Parse(input);
if("temperature < 21")
{
System.Console.WriteLine("Too cold!");
}
else if("temperature == 22);
{
System.Console.WriteLine("Just right!");
}
else
{
System.Console.WriteLine("Too hot!");
}
Moderator edited: Markdown added so that code renders properly in the forums.
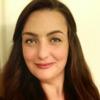
Jennifer Nordell
Treehouse TeacherHi again! Now you have some syntax errors. Because you've included your temperature < 21
in quotation marks, it is now a string instead of an expression. You will need to remove the quotation marks there. You also have a stray quotation mark in your else if
statement. The code should print out "Just right" if the temperature is 21 or 22.

Michael Bragg
811 Pointsstring input = Console.ReadLine();
int temperature = int.Parse(input);
if("temperature < 21")
{
System.Console.WriteLine("Too cold!");
}
else if("temperature == 22");
{
System.Console.WriteLine("Just right!");
}
else
{
System.Console.WriteLine("Too hot!");
}
Moderator edited: Markdown added so that code renders properly in the forums.

Michael Bragg
811 Pointsstring input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature < 21)
{
System.Console.WriteLine("Too cold!");
}
else if(temperature == 22);
{
System.Console.WriteLine("Just right!");
}
else
{
System.Console.WriteLine("Too hot!");
}
Not a string any longer and still bummer
Moderator edited: Markdown added so that code renders properly in the forums.

Michael Bragg
811 Pointsstring input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature < 21)
{
System.Console.WriteLine("Too cold!");
}
else if(temperature == 22);
{
System.Console.WriteLine("Just right!");
}
else
{
System.Console.WriteLine("Too hot!");
}
Moderator edited: Markdown added so that code renders properly in the forums.
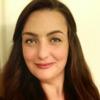
Jennifer Nordell
Treehouse TeacherSpamming this thread will not make anyone more inclined to give you an explicit answer. Again, the code should print out "Just right" if the temperature is 21. It should also print out "Just right" if the temperature is 22. Either one of those temperatures fall in the "Just right" range.

Michael Bragg
811 Pointsstring input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature < 21)
{
System.Console.WriteLine("Too cold!");
}
else if(temperature == 22);
{
System.Console.WriteLine("Just right.");
}
else
{
System.Console.WriteLine("Too hot!");
}
with correct punctuation even Bummer
Moderator edited: Markdown added so that code renders properly in the forums.
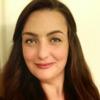
Jennifer Nordell
Treehouse TeacherPlease read what I have written repeatedly. Your code currently prints out "Just right." when the temperature is 22. It should also print out "Just right." when the temperature is 21. What does your code currently print out when the temperature is 21? It prints out "Too hot!".

Michael Bragg
811 PointsHow am I spamming posting my code Jennifer?
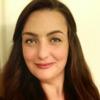
Jennifer Nordell
Treehouse TeacherPersonally, I feel like posting within a few seconds of each other while completely disregarding what was written back to you several posts up could be considered spamming.

Michael Bragg
811 PointsMaybe I am behind in the posts, but my code now reads as you suggested
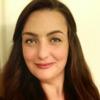
Jennifer Nordell
Treehouse TeacherThe last piece of code that you posted contains this: else if(temperature == 22);
.
But again, the code should print out "Just right." if the temperature is 22 or 21. Either one. But currently, your code only prints out "Just right." when it's 22.

Michael Bragg
811 PointsJennifer, I am making changes to my code and posting updates.

Michael Bragg
811 PointsI have corrected what you suggested:
string input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature < 21)
{
System.Console.WriteLine("Too cold!");
}
else if(temperature >= 21 && <= 22);
{
System.Console.WriteLine("Just right.");
}
else
{
System.Console.WriteLine("Too hot!");
}
Moderator edited: Markdown added so that code renders properly in the forums.

Michael Bragg
811 Points...html
string input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature < 21)
{
System.Console.WriteLine("Too cold!");
}
else if(temperature >= 21 && temperature <= 22);
{
System.Console.WriteLine("Just right.");
}
else
{
System.Console.WriteLine("Too hot!");
}
Moderator edited: Markdown added so that code renders properly in the forums. ...
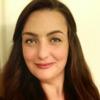
Jennifer Nordell
Treehouse TeacherOk now it's correct except for one semicolon. You've placed an extra semicolon at the end of the expression in the else if
. Removing that will cause it to pass.

Michael Bragg
811 Points...html <p> string input = Console.ReadLine(); int temperature = int.Parse(input);
if(temperature < 21) { System.Console.WriteLine("Too cold!"); } else if(temperature >= 21 && temperature <= 22); { System.Console.WriteLine("Just right."); } else { System.Console.WriteLine("Too hot!"); } </p> ...
This is my current code and still Bummer
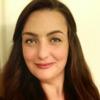
Jennifer Nordell
Treehouse TeacherAgain, you have an extra semicolon at the end of the expression in the else if
that is causing a syntax error. Removing that will cause the challenge to pass.

Michael Bragg
811 PointsThank you, Thank you, Thank you.