Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial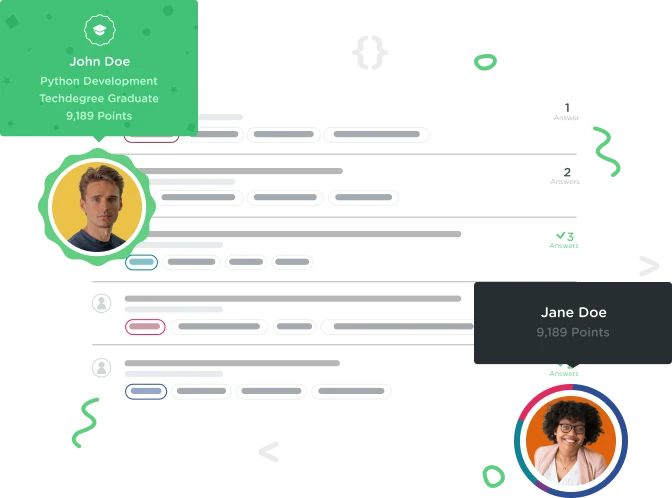

Massimo Scola
Courses Plus Student 632 PointsnormalizeDiscountCode => NullPointerException - why?
What is wrong here?
private String normalizeDiscountCode(String aDiscountCode) { String newString = aDiscountCode.toUpperCase(); return newString; }
I am returning the upper case version of the String aDiscountCode. Why is there an error here?
Isn't this what I am asked to do? Transform a string to upper case?
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
private String normalizeDiscountCode(String aDiscountCode) {
String newString = aDiscountCode.toUpperCase();
return newString;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(this.discountCode);
}
}
2 Answers
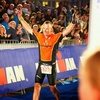
Steve Hunter
57,712 PointsHi Massimo,
The issue here seems to be the call to normalizeDiscountCode
. The compiler is complaining about the parameter you are sending out of applyDiscountCode
. It doesn't like the fact that the class member variable is being passed, this.discountCode
. If you just pass discountCode
it work fine; in terms of the exception, anyway. I'm not sure but this may be because this.discountCode
is private
; perhaps that means it can't be directly passed - without loading the code into Intelli-J to get more detailed error messages, I can't tell.
Back to the challenge, though. In normalizeDiscountCode
for the first part of this challenge, you just want to return the received string in upper case form. We don't need to create a new string variable, but you can if you want to. Fewer lines of code would look like:
private String normalizeDiscountCode(String aDiscountCode) {
return aDiscountCode.toUpperCase();
}
Inside applyDiscountCode
, assign the returned value from normalizeDiscountCode
into this.discountCode
. The parameter you send to normalizeDiscountCode
is the same that this method receives. Don't send the member variable directly.
public void applyDiscountCode(String discountCode) { // <- send that one
this.discountCode = normalizeDiscountCode(discountCode); // <- no 'this' here
}
I hope that makes sense.
Steve.

Massimo Scola
Courses Plus Student 632 PointsThere was a problem with the compiler as even something like this didn't work:
public String normalizeString(String aString)
{
return aString.toUpperCase();
}
Which works in other IDEs. After I restarted everything worked again properly.
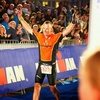
Steve Hunter
57,712 PointsThe challenge compiler is very precise as to what it is expecting to see - entering general code in there is unlikely to work. The Preview button will show you any syntax errors but the challenge will fail if the task isn't passed.
Some of the challenges won't be 'fully loaded' so they'll only understand certain aspects of Java relating to the task in hand.
I hope you got it sorted and can move on. The next part of the challenge is frequently asked about; think your logic through about the allowed characters.
Good luck!
Steve.
P.S. Shout if you need any help on any courses.