Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial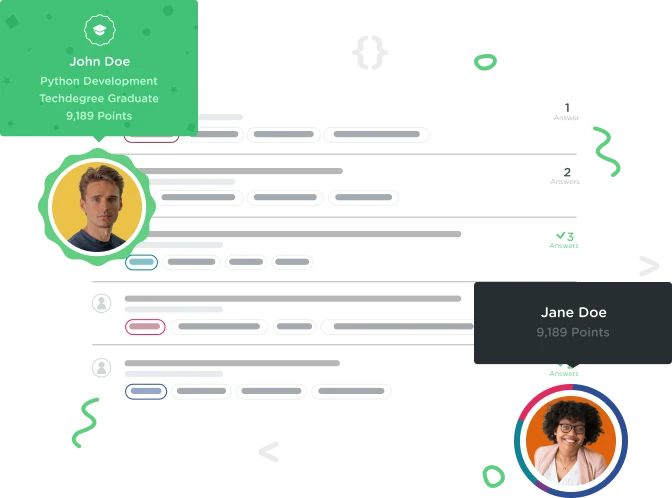

David Huck
3,676 PointsNot 100% sure what I am missing. It says "Looks like task 2 no longer passes" but when I go back, task 2 passes.
I go back to task 2 and without changing anything it passes just fine. Same with task 3. Any help would be appreciated.
public class Forum {
private String mTopic;
// TODO: add a constructor that accepts a topic and sets the private field topic
public Forum(String topic){
mTopic = topic;
}
public String getTopic() {
return mTopic;
}
// Uncomment this when you are prompted to do so
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
mTopic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
}
public class User {
// TODO: add private fields for firstName and lastName
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
// TODO: set and add the private fields
mFirstName = firstName;
mLastName = lastName;
}
// TODO: add getters for firstName and lastName
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
}
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
// TODO: add a constructor that accepts the author, title and description
public ForumPost(User author, String title, String description) {
mAuthor = author;
mTitle = title;
mDescription = description;
}
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getDescription() {
return mDescription;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
// Uncomment this when prompted
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
User author = new User(args[0], args[1]);
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost(author, "This is your forum post title", "This is your forum post description");
forum.addPost(post);
}
}
2 Answers

Dino Šporer
5,430 Points1) public Forum (String topic) { this.topic = topic; }
2)private String firstName; private String lastName;
this.firstName = firstName; this.lastName = lastName;
public String getFirstName() { return firstName; }
public String getLastName() { return lastName; }
3) public ForumPost (User author, String title, String description ) { this.author = author; this.title = title; this.description = description; }
4) Forum forum = new Forum("Java"); User author = new User(args[0], args[1]); ForumPost post = new ForumPost(author, "new title", "no description"); forum.addPost(post);
Just check it out one more, I think the problem is you user mFirstName instead of firstName, but in the task , firstName is what they really want

David Huck
3,676 PointsThank you. Went back and looked at the variables. Changed them all to "this.var = var" and that seemed to do the trick.