Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial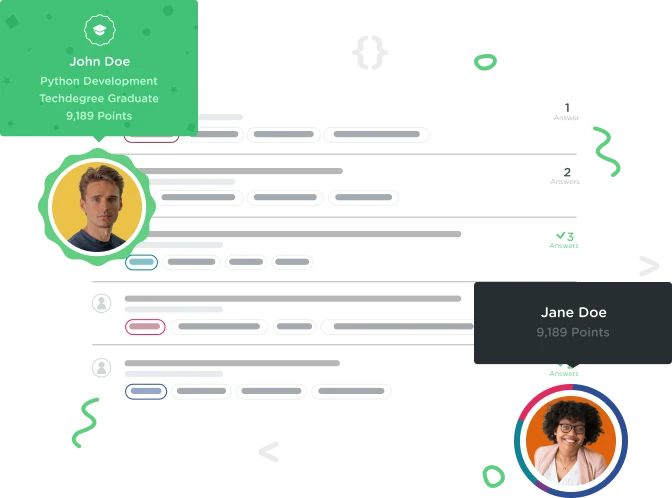

Saad Hamid
3,150 PointsNot Able to complete the challenge Exercise
I am having trouble in completing task 2 of Apply Discount code exercise
I have written method for "normalized" method, which throws an error that task 1 is not passing
I am not able to figure out what's wrong with the code
Can anyone please help?
private String normalizeDiscountCode (String discountCode)
{
discountCode = discountCode.toUpperCase();
for(char charArr : discountCode.toCharArray()){
if((!Character.isLetter(charArr)) && (!discountCode.contains("$")))
{
throw new IllegalArgumentException("Invalid Discount Code");
}
}
return discountCode;
}
public void applyDiscountCode(String discountCode) {
discountCode = normalizeDiscountCode(discountCode);
this.discountCode = discountCode;
}
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
private String normalizeDiscountCode (String discountCode)
{
discountCode = discountCode.toUpperCase();
for(char charArr : discountCode.toCharArray()){
if((!Character.isLetter(charArr)) && (!discountCode.contains("$")))
{
throw new IllegalArgumentException("Invalid Discount Code");
}
}
return discountCode;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
discountCode = normalizeDiscountCode(discountCode);
this.discountCode = discountCode;
}
}
3 Answers
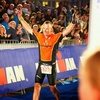
Steve Hunter
57,712 PointsHi Saad,
You're pretty close with that. However, you want to test each letter within the for loop. Having a blanket contains
for the whole string removes that specificity that you need.
Your first test is correct, the second should simply check if the character does not equal '$'
.
My code looks like:
private String normalizeDiscountCode(String code){
for(char letter : code.toCharArray()){
if(!Character.isLetter(letter) && letter != '$'){
throw new IllegalArgumentException("Invalid discount code");
}
}
return code.toUpperCase();
}
I hope that helps.
Steve.

Houssem Mhimdi
664 Points@Saad Hamid You have to use matches() instead to verify that you voucher contains only characters and ignore the other special characters but allow $.... so instead use this:
if( !Character.isLetter(charArr) && !discountCode.matches("[a-zA-Z$]+") )

Saad Hamid
3,150 PointsThank you Houssem Mhimdi and Steve Hunter for your help. I am able to get through my problem with your solution.
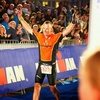
Steve Hunter
57,712 PointsNo problem! Glad you got it fixed. Shout if you run into any more problems!
Steve
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsThe test fires the code "
$@ving$
" at your code, for example - there are other tests behind the scenes.Let's work your logical tests:
if((!Character.isLetter(charArr)) && (!discountCode.contains("$")))
So, let's use the
@
symbol which is what the test is expecting to fail. The first test isisLetter
which will returnfalse
which is then negated totrue
.The second test
contains("$")
will returntrue
, negated tofalse
. The&&
has atrue
and afalse
so it won't throw the exception.Testing for:
if(!Character.isLetter(letter) && letter != '$')
First test
@
isn't a letter; negated totrue
. The second test is alsotrue
as '@' is not equal to '$'. Twotrue
evaluations means the exception gets thrown.Steve.
Houssem Mhimdi
664 PointsHoussem Mhimdi
664 PointsDid you tested this ??
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsYeah - it ran fine for me. Did it not work for you?
Houssem Mhimdi
664 PointsHoussem Mhimdi
664 PointsYes i just tested it right now, it works im still gravitated more to the matches() method instead ...
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsI might agree if this were a real-world problem but it isn't. I'm aware of the course content and what's been taught so far. The challenges are designed to reiterate the content of the previous videos which helps reinforce that content and help with learning.
Regular expressions haven't been taught yet at this point of the Java track, so I'm keeping 'on message' supporting Saad by adhering to course content rather than introducing something that's totally new inside a forum post. There's lots of ways of solving many of the challenges - many are much more elegant code-wise but that's not what the challenges are about. By reinforcing the video content, we help Treehouse students learn by repetition and reinforcement.
Houssem Mhimdi
664 PointsHoussem Mhimdi
664 Pointstotally agree on this.