Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial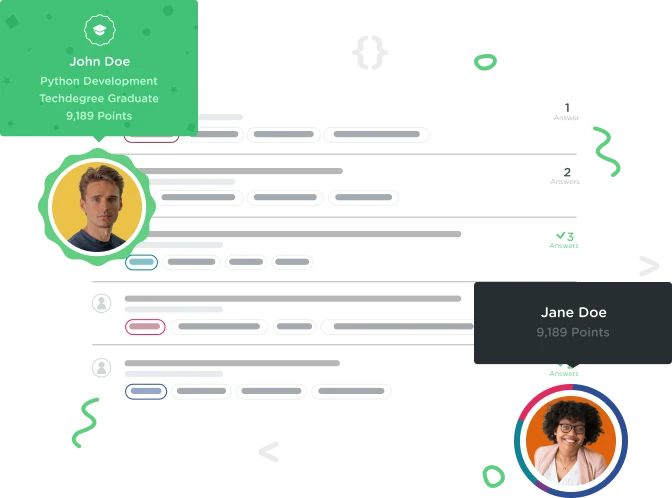

RUCHI MISHRA
2,968 PointsNot able to get expected output, as issue in my nested loops
attached rectified code
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.ArrayList;
import java.util.Set;
import java.util.TreeSet;
import java.util.Map;
import java.util.HashMap;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
public List<String> getAllCategory() {
List<String> category = new ArrayList<>();
for (BlogPost post: mPosts) {
category.add(post.getCategory());
}
return category;
}
public Map<String, Integer> getCategoryCounts()
{
Map<String, Integer> categoryCounts = new HashMap<String, Integer>();
// List<String> category = new ArrayList<String>();
for (BlogPost post: mPosts) {
// category.add(post.getCategory());
for(String categ : getAllCategory() )
{
Integer count = categoryCounts.get(categ);
if(count == null)
{
count = 0;
}
count++;
System.out.printf("%s %d",categ, count);
categoryCounts.put(categ,count);
}
}
return categoryCounts;
}
}
2 Answers

Yanuar Prakoso
15,196 PointsHi Ruchi
You actually only need to add one method that is the getCategoryCounts method. You do not need getAllCategory method and most important you do not need any nested loops. This is what I proposed for you to pass the challenge:
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> categoryCounts = new HashMap<String, Integer>();
//you do not need the public List<String> getAllCategory() method
//you do not need nested for loops.
for (BlogPost post: mPosts) {
if (categoryCounts.get(post.getCategory()) == null){
categoryCounts.put(post.getCategory(), 0);
}
int count = categoryCounts.get(post.getCategory());
count++;
categoryCounts.put(post.getCategory(), count);
}
return categoryCounts;
}
I hope this will help you pass the challenge.
If you still curious with the logic behind the code here some explanation. Little bit of warning that it might get long and boring, so if you already understand it by reading the code you may pass the explanations below:
First of all you only need to map the category and how many times it comes up in the blog. Thus you need FOR loops all over the posts. Then from EACH post you need to GET the category of that specific post to use it to count how many times it comes up in the blog.
However, one thing about map or HashMap is IF you have not listed the specific post category (passed using post.getCategory()) into the Hashmap before it will return the counts of comes up as null not zero (0) which is troublesome and in many cases invokes errors. This also indicate that there are no key on such post that you need to set it first using:
if (categoryCounts.get(post.getCategory()) == null){
categoryCounts.put(post.getCategory(), 0);
}
use PUT to set the KEY in this case the post category to VALUE of zero as it is just added to the map. We will add the come up number value after we finish the IF statement process. As you can see after finishing the IF process which add new mapping of the post category to zero comes up number it add the counts.:
int count = categoryCounts.get(post.getCategory());
count++;
categoryCounts.put(post.getCategory(), count);
IF the post category already mapped inside the getCategoryCounts meaning it is already a key thus it will pass without entering IF ststement it will add the count of come ups. Then we just get the last number of how many it comes up by using GET method from the HashMap class.
In the end it will update the number of come ups of each key post category by using put method pairing the current post category to new count value after being added by one (count++). Then you just return the categoryCounts map. That is all
Sorry for the long explanation. I hope this will also help you a little.

RUCHI MISHRA
2,968 PointsThanks a lot for such detailed explanation, it worked