Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial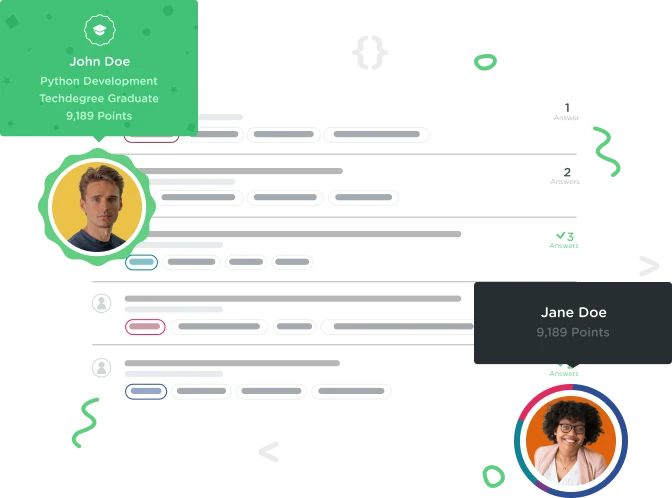

Arun Shivaramakrishna
4,771 Pointsnot able to get the mistake in the code
def most_classes(dict): dict = {'PQR':['ruby foundation', 'ruby on rails', 'Tech foundations'], 'ABC':['python basics' , 'python collection']} max_count = 0 for key in dict: if value in key: count[value] +=1 print(max(count[value]), dict[key])
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(dict):
dict = {'PQR':['ruby foundation', 'ruby on rails', 'Tech foundations'], 'ABC':['python basics' , 'python collection']}
max_count = 0
for key in dict:
if value in key:
count[value] +=1
print(max(count[value]), dict[key])
3 Answers
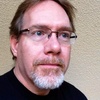
Chris Freeman
Treehouse Moderator 68,423 PointsThere a few things to clear up in your code.
-
dict
does not need to be defined. It's value is set by the arguments whenmost_classes()
is referenced by the grader code. Comment outdict = ....
line - The
count
dictionary needs to be initialized. Addcount = {}
to init as empty dictionary. - When checking if
value
is present in thecount
dict, you need to reference the value asdict[key]
and to check against keyscount.keys()
. That is:if dict[key] in count.keys()
- You need to compare the current
count[value]
against max_count to see if a new maximum has been reached. - As the hints suggest "Better hold onto the teacher name somewhere", perhaps in a new variable max_teacher
- While a print shows the values, you need to use a
return
statement to pass values back to the grader. Replaceprint()
withreturn
. And return the teacher's name ("max_teacher") and the count value ("count[value]"). Notereturn
does not need parens. - Best to use 4-space indent instead of 2-spaces.
def most_classes(dict):
dict = {'PQR':['ruby foundation', 'ruby on rails', 'Tech foundations'], 'ABC':['python basics' , 'python collection']}
max_count = 0
for key in dict:
if value in key:
count[value] +=1
print(max(count[value]), dict[key])
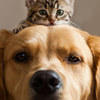
Devin Scheu
66,191 PointsThis is the code I got for this challenge:
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(teacher_dict):
max_count = 0
busy_teacher = ''
for teacher1,teacher2 in teacher_dict.items():
if len(teacher2) > max_count:
max_count = len(teacher2)
busy_teacher = teacher1
return busy_teacher
def num_teachers(dct):
return len(dct)
def stats(arg3):
teacherList = []
for key in arg3:
tempList = []
tempList.append(key)
tempList.append(len(arg3[key]))
teacherList.append(tempList)
return teacherList
def courses(teachers):
total_courses = []
end_result = []
for key in teachers.items():
total_courses.append(teachers.values())
for courses_of_one_teacher in teachers.values():
for course in courses_of_one_teacher:
end_result.append(course)
return end_result

Arun Shivaramakrishna
4,771 PointsThanks Chris!