Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial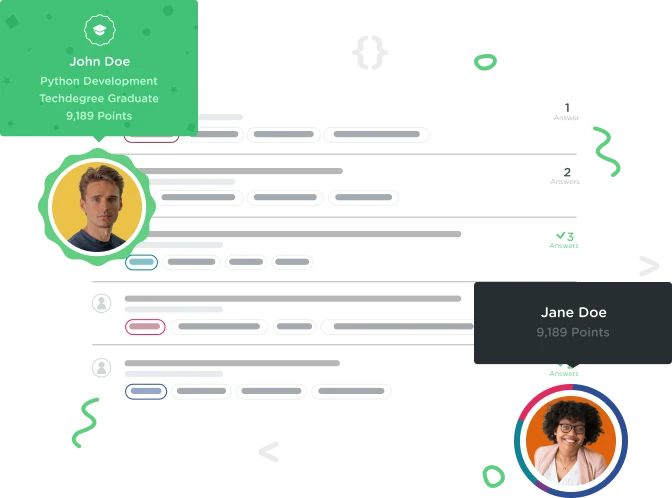

Peter Gess
16,553 PointsNot able to get the right result comparing two sets
I am not sure where my code is wrong...please assist!
Great work!
OK, let's create something a bit more refined. Create a new function named covers_all that takes a single set as an argument. Return the names of all of the courses, in a list, where all of the topics in the supplied set are covered.
For example, covers_all({"conditions", "input"}) would return ["Python Basics", "Ruby Basics"]. Java Basics and PHP Basics would be excluded because they don't include both of those topics.
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers_all(args):
result = []
for key, value in COURSES.items():
if args & COURSES.values():
result.append(value)
return result
3 Answers

Cheo R
37,150 PointsYou're close.
if args & COURSES.values():
COURSES.values() is not a set (type(COURSES.valuess()) gives dict_values). But you can do:
if args & value
Because type(value) is a set.
Then all you need to swap out is what you append to your result (you want the course name).

Cheo R
37,150 PointsMy mistake, looks like
if args & value
Is true if at least one of the args is present in value. So all you'd have to do is to check if both are present.

Peter Gess
16,553 PointsYes I have that...so why isn't it working?

Cheo R
37,150 PointsWhat does your current code look like now?

Peter Gess
16,553 PointsThanks for you continued help Cheo. I found another implementation that used difference and it passed...sometimes these code challenges can be really finicky.
Peter Gess
16,553 PointsPeter Gess
16,553 PointsThanks Cheo.
I modified my code but still not passing...Shouldn't I be appending the key?