Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial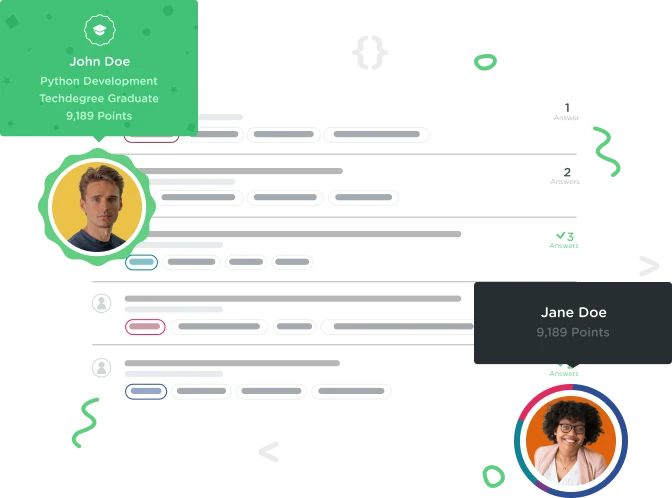

Jery Althaf
5,818 PointsNot able to get through this challenge.
import android.app.Activity; import android.util.Log; import com.parse.ParseUser; import com.parse.ParseException; import com.parse.SignUpCallback;
public class SignUpActivity extends Activity {
String username="jeryalthaf";
String password="jeryalthaf";
public static final String TAG = SignUpActivity.class.getSimpleName();
protected SignUpCallback mSignUpCallback = new SignUpCallback() {
ParseUser newUser = new ParseUser();
newUser.setUsername(username);
newUser.setPassword(password);
@Override
public void done(ParseException e) {
}
};
// onCreate() and other code has been omitted
protected void signUp(String username, String password) {
}
}
Shows error eachtime
import android.app.Activity;
import android.util.Log;
import com.parse.ParseUser;
import com.parse.ParseException;
import com.parse.SignUpCallback;
public class SignUpActivity extends Activity {
String username="jeryalthaf";
String password="jeryalthaf";
public static final String TAG = SignUpActivity.class.getSimpleName();
protected SignUpCallback mSignUpCallback = new SignUpCallback() {
ParseUser newUser = new ParseUser();
newUser.setUsername(username);
newUser.setPassword(password);
@Override
public void done(ParseException e) {
}
};
// onCreate() and other code has been omitted
protected void signUp(String username, String password) {
}
}
Tyler Vanderley
20,491 PointsTyler Vanderley
20,491 PointsHaven't been active in a long time, and this looks like an old question, but for anyone stumbling on this in the future, I hope this helps.
You were close in what you said below, which is as follows:
But you just put this snippet of code in the wrong place:
Where it should be is in the signUp() method, which would look something like this:
Now, for a brief explanation as clearly as I can, and I apologize if I'm unclear (long day at work, yada, yada, yada). What you originally did is you created a new ParseUser object and set his or her username and password in the SignUpCallback object that we created called mSignUpCallback.
The problem may not seem immediately obvious until we get to the next part of the challenge, which prompts you to use the signUpInBackground() method of the ParseUser object that you created (newUser). Following the direction you were headed originally would yield something that looks like this NOTE PLEASE DON'T USE THE BELOW CODE AS IT'S INCORRECT AND USED ONLY FOR ILLUSTRATION:
Notice that we're starting to pass the object that we're creating as a parameter, which should look a little funny, but more importantly, it's not adding any functionality to the method that we're creating below, signUp(). This means, when we go to call the signUp() method later, it wouldn't do anything for us; however, if we move that code you wrote down into the signUp() method, we have a perfectly good method to call later. So, when we say:
signUp("ObviousName", "ObviousPassword");
We will have created a new ParseUser with the username ObviousName and the password ObviousPassword.
I hope this helps, and I'm sorry for the long answer, I just think that the why contributes as much to the conversation as the what.