Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial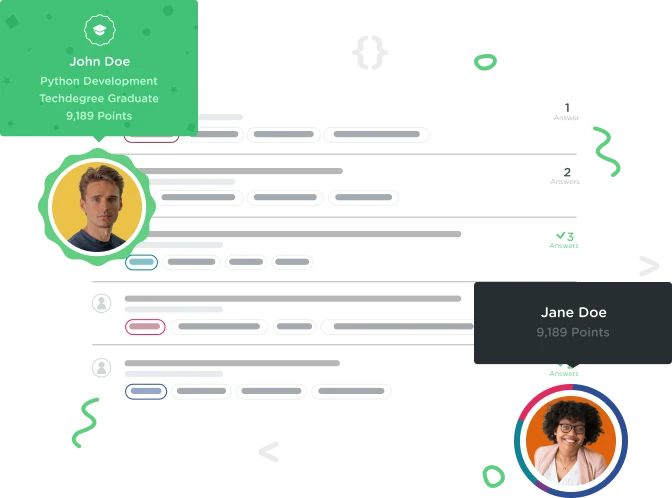

Jonathan Ambriz
Courses Plus Student 3,902 PointsNot able to view game. Clicking button does not run startGame(); Chrome console error provided.
Here is the error I get in Chrome: Uncaught TypeError: this.spaces is not iterable (line 29)
Here is my Board.js file (the one referenced for line 29):
class Board { constructor() { this.rows = 6; this.columns = 7; this.spaces = this.createSpaces(); }
/**
* Generates 2D array of spaces
* @return {Array} An array of space objects
*/
createSpaces(){
const spaces = [];
for (let x=0; x< this.columns; x++){
const column = [];
for (let y = 0; y< this.rows; y++) {
const space = new Space(x,y);
column.push(space);
}
spaces.push(column)
}
}
/**
* Render Method for drawing HTML Board using drawSVGSpace()
*/
drawHTMLBoard(){
for (let column of this.spaces){
for (let space of column){
space.drawSVGSpace();
}
}
}
}
1 Answer
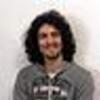
Gergely Bocz
14,244 PointsHi Jonathan!
I think the problem comes from missing return spaces from you code! Because of that this.spaces in the constructor does call the createSpaces() function, but the object doesn't get assigned the values of the const spaces[] array inside createSpaces()!
Try replacing your createSpaces() function with this:
createSpaces(){
const spaces = [];
for (let x=0; x< this.columns; x++){
const column = [];
for (let y = 0; y< this.rows; y++) {
const space = new Space(x,y);
column.push(space);
}
spaces.push(column);
}
return spaces;
}
I just added the return spaces line before the last bracket.
I hope it works now!
Jonathan Ambriz
Courses Plus Student 3,902 PointsJonathan Ambriz
Courses Plus Student 3,902 PointsThis is the full console error: Board.js:29 Uncaught TypeError: this.spaces is not iterable at Board.drawHTMLBoard (Board.js:29) at Game.startGame (Game.js:33) at HTMLButtonElement.<anonymous> (app.js:6)
It seems to be due to the use of spaces in the constructor of a Board object. I checked the working code along side this one and don't see the difference. Here's the code for the Board constructor:
class Board { constructor() { this.rows = 6; this.columns = 7; this.spaces = this.createSpaces(); }