Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial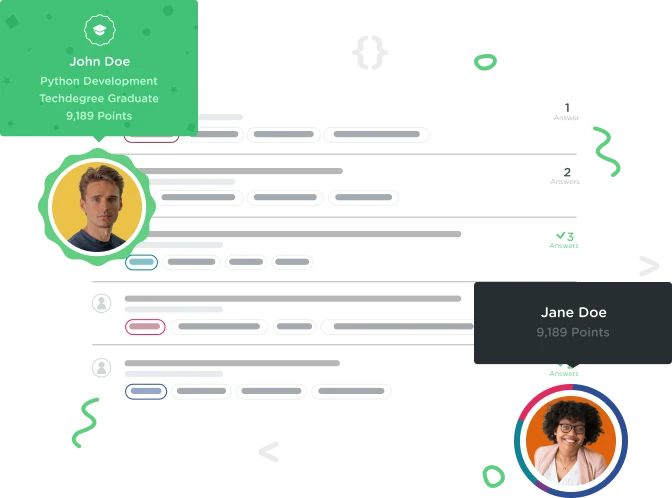

Amit Ghosh
9,314 PointsNot all code paths returns a Value
I am trying with the variations, somehow I get this error. Any thoughts.
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
class RepeatDetector: SequenceDetector
{
public override bool Scan(int[] sequence1)
{
for( int i=0 ; i < sequence1.Length-1 ; i++)
{
if (sequence1[i] == sequence1[i+1])
{
return true;
break;
}
else
{
return false;
}
}
}
}
}
2 Answers

andren
28,558 PointsThere is more than 1 issue with your code, but since you say you are trying to figure stuff out yourself I'll only point out the cause of the current error message you get, you can ask me follow up question if you need further assistance.
The cause of that error is the fact that in C# no matter what happens your function needs to return something when called. Your function only returns values within the for loop, if the for loop never runs (an empty int array is passed for example) then your function will never return anything.
This can be fixed by adding a return statement after the for loop.

Amit Ghosh
9,314 PointsHi Andren,
I made it work with below:
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence1)
{
if (sequence1.Length <2)
{
return false;
}
for ( int i=1 ; i< sequence1.Length ; i++)
{
if (sequence1[i] == sequence1[i-1])
{
return true;
}
}
return false;
}
}
}
However what I don't understand if the length check is necessary to perform.

andren
28,558 PointsWith the way your code is written the first length check is indeed unnecessary. If the array length is 1 or 0 then the for loop will never run since it's starting condition would be false from the start, which means that the function will return false in those cases even without the first if statement.