Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial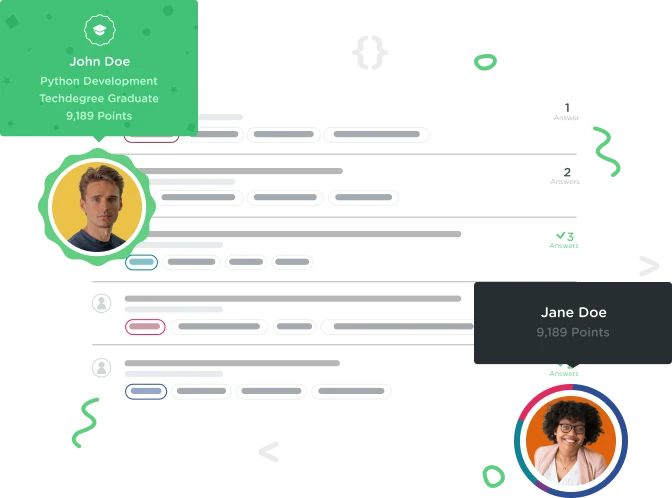
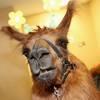
A X
12,842 PointsNot Clear How a Function Can Overwrite a Global Variable
Javascript
<html>
<head>
<title>name - let and Const</title>
</head>
<body>
<h1>Name</h1>
<script>
var name = "Andrew";
function createFullName(fName, lName) {
name = fName + " " + lName;
console.log(name);
}
console.log(name);
createFullName("Joel", "Kraft");
</script>
</body>
</html>
We find as a problem w/ using the var keyword exclusively instead of const in this video that a global variable can get overwritten by a function, but I'm not clear how that is or why that is as I thought that functions were localized. Can someone explain how a function can overwrite a global variable? Isn't the code example more of a case of bad naming practices than "needing" to use a const?
3 Answers
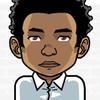
webdesignertroy
Front End Web Development Techdegree Graduate 14,718 PointsThis is a question of scope. And, it's not that functions are localized. The correct statement is: Variables named within a function are localized. (See http://www.w3schools.com/js/js_scope.asp. )
Browsers that read JavaScript read it like human beings: from left to right and from top to bottom in that order. Instead of thinking about variables and words like “override,” think of a variable like a newly created sticker after we define it (or give it a value). As we read the script from left to right and top to bottom, imagine placing the sticker on our favorite notepad. The first sticker has the name of Andrew.
When we call (or invoke) the function with command createFullName("Joel", "Kraft")
, this is when we enter the function to find yet another sticker with the name “Joel Kraft” (name = fName + " " + lName;
). In this instance, we place the sticker “Joel Kraft” over initial sticker “Andrew”. We can now only see Joel Kraft on the notebook. We continue to read through the function from left to right and top bottom until we complete all of the function’s instructions.
Here is your example script in real-time: http://codepen.io/webdesignertroy/pen/ZBvzwm
In this CodePen, comment out line 5 in the JS to witness the order of operations the browser is utilizing. When you comment out line 5, you get the name Andrew. This shows that Andrew was written to the DOM first before it was overwritten by the function and its local variable, called afterwards.

Andrzej Hanusek
6,388 PointsBut if you use the "var" keyword in function it give you the same result like with the "const" and in global scope the variable name has value = "Andrew".
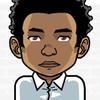
webdesignertroy
Front End Web Development Techdegree Graduate 14,718 Points@Andrzej Hanusek - "Redefining" variables and "Re-Declaring and Defining" variables are two different approaches that are not quite related to each other. When we use the var keyword and utilize the same variable name, we "re-declare and define" a variable. In your example above, we simply "re-define" a variable, or add a new value, to an already existing "name" variable. The two approaches are subject to slightly different rules and implications in terms of scope.
That being said. "Re-declaring and defining" a variable is same as creating a new variable; It's a new variable with the same name. Furthermore, the old variable name is overwritten, or written out of existence. So, if I understand your statement correctly, your claimed result is incorrect:
But if you use the "var" keyword in function it give you the same result like with the "const" and in global scope the variable name has value = "Andrew".
This can be proven by simply rewriting the editable CodePen I linked to this question.
const name = "Andrew";
function createFullName(fName, lName) {
var name = fName + " " + lName;
document.getElementById("name").innerHTML = name;
}
document.getElementById("name").innerHTML = name;
createFullName("Joel", "Kraft");
The output here is "Joel Kraft" and not "Andrew".
Please review your statement.

Christopher Bobek
Courses Plus Student 12,329 PointsHey, nekilof. Check this out . . .
/* Open terminal, launch Node, and run the code below */
var name = "Joe Strummer";
var tripOut = function () {
var name = "Mick Jones";
console.log(name);
console.log(this.name);
};
Using the keyword var
within the function creates a new variable with the same name as the global variable that was defined above the function. If you omit the keyword 'var' in the function definition, you'll change the global variable.
Call tripOut()
with and without the var
keyword to see what happens.