Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial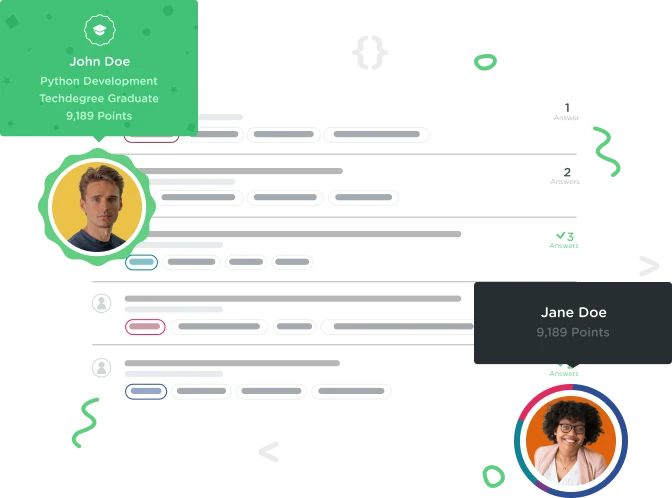

sujal khatiwada
Courses Plus Student 389 PointsNot displaying Breakfast recipes
this is my code for cookbook.php
<?php
include"classes/recipes.php";
include"classes/render.php";
include"classes/recipecollection.php";
include"inc/recipes.php";
$cookbook=new RecipeCollection("Treehouse Recipes");
$cookbook->addRecipe($lemon_chicken);
$cookbook->addRecipe($dried_mushroom_ragout);
$cookbook->addRecipe($rabbit_catalan);
$cookbook->addRecipe($grilled_salmon_with_fennel);
$cookbook->addRecipe($pistachio_duck);
$cookbook->addRecipe($chili_pork);
$cookbook->addRecipe($crab_cakes);
$cookbook->addRecipe($beef_medallions);
$cookbook->addRecipe($silver_dollar_cakes);
$cookbook->addRecipe($french_toast);
$cookbook->addRecipe($corn_beef_hash);
$cookbook->addRecipe($granola);
$cookbook->addRecipe($spicy_omelette);
$cookbook->addRecipe($scones);
$breakfast=new RecipeCollection("Favorite Breakfasts");
foreach($cookbook ->filterByTag("breakfast") as $recipe){
$breakfast->addRecipe($recipe);
}
//var_dump($cookbook);
echo Render::listRecipes($breakfast->getRecipeTitles());
//echo Render::displayRecipe($belgian_waffles);
?>
and code for recipecollection.php is
<?php
class RecipeCollection{
private $title;
private $recipes =array();
public function __construct($title=null){
$this->setTitle($title);
}
public function setTitle($title)
{
if(empty($title)){
$this->title=null;
}else{
$this->title=ucwords($title);
}
}
public function getTitle()
{
return $this->title;
}
public function addRecipe($recipe){
$this->recipes[]= $recipe;
}
public function getRecipes()
{
return $this->recipes;
}
public function getRecipeTitles(){
$titles=array();
foreach($this->recipes as $recipe){
$titles[]=$recipe->getTitle();
}
return $titles;
}
public function filterByTag($tag){
$taggedRecipes=array();
foreach($this->recipes as $recipe){
if(in_array(strtolower($tag), $recipe->getTags())){
$taggedRecipes[]=$recipe;
}
return $taggedRecipes;
}
}
}
?>
but I cant see the output
Moderator edited: Added markdown so the code renders properly in the forums.
1 Answer

Uriahs Victor
676 PointsYou have the return $taggedRecipes;
inside the scope of the foreach loop in your recipecollection.php try moving it one parenthesis down and test again
A function can only return once, and your return wasn't even in the if statement(which would still be wrong), so it would just return on the first foreach loop and stop execution.