Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial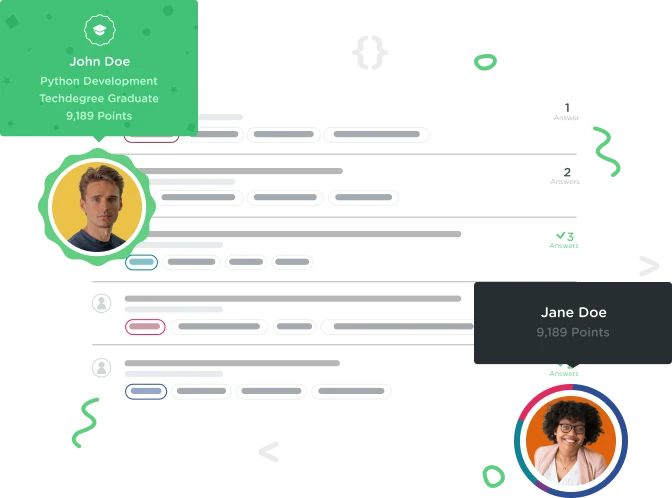

Mahfuzur Rahman
3,204 Pointsnot executing the code inside of loop
also having some weird output display while running the line break
for ($i=1; $i<=100; ++$i) { echo $i."<br /> \n";
while((list ($key, $val)=each($facts)) && (isset($i)===$facts[key])){
echo "$key=> $val \n"; }
}
<?php
$facts = array(
57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.',
2 => ' is the approximate hours a day Giraffes sleeps',
18 => ' is the average hours a Python sleeps per day',
10 => ' per cent of the world is left-handed.',
11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.',
98 => '% of the atoms in your body are replaced every year',
69 => ' is the largest number of recorded children born to one woman',
);
//add your loop below this line
for ($i=1; $i<=100; ++$i) {
echo $i."<br /> \n";;
while((list ($key, $val)=each($facts)) && (isset($i)===$facts[key])){
echo "$key=> $val \n";
}
}
5 Answers
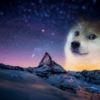
Connor Walker
17,985 PointsI'm not entirely sure what is going on inside your code but I think you are over complicating your it. Only one loop (the for loop) is needed for this challenge.
for ($i = 1; $i <= 100; $i++) {
echo $i;
if (isset($facts[$i])) {
echo $facts[$i] . "<br />\n";
}
}
You don't need to concatenate anything to $i while echoing it and use the isset function to check the $facts array with the index of $i (shown above)

Tobias Karlsson
2,751 PointsI haven't gone through the PHP courses but it seems odd that you should loop 100 times and check if there is a key set for each iteration in the loop. Can't you use count()
, next()
, each()
etc. to solve it. Something like this:
<?php
for ($i = 0; $i < count($facts); $i++) {
$item = next($facts);
echo key($facts) . $item . "\n";
}
or
<?php
for ($i = 0; $i < count($facts); $i++) {
[$key, $fact] = each($facts);
echo $key . $fact . "\n";
}
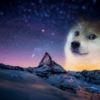
Connor Walker
17,985 PointsIn this example with the $facts array you wouldn't be able to use for ($i = 0; $i < count($facts); $i++) {
because the facts array only has 7 elements so only the giraffe fact would be displayed (because the indexes have been set seperatly).
One alternative to this could be the for each loop
foreach ($facts as $fact){
echo $fact . "<br>";
}
This would print out each item in the array without the use of a counter, however the index number wouldn't be displayed

Tobias Karlsson
2,751 PointsI do not understand what you mean by this?
(because the indexes have been set seperatly).
The array is like this, isn't it?
<?php
$facts = array(
57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.',
2 => ' is the approximate hours a day Giraffes sleeps',
18 => ' is the average hours a Python sleeps per day',
10 => ' per cent of the world is left-handed.',
11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.',
98 => '% of the atoms in your body are replaced every year',
69 => ' is the largest number of recorded children born to one woman',
);
and this code
<?php
$facts = array(
57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.',
2 => ' is the approximate hours a day Giraffes sleeps',
18 => ' is the average hours a Python sleeps per day',
10 => ' per cent of the world is left-handed.',
11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.',
98 => '% of the atoms in your body are replaced every year',
69 => ' is the largest number of recorded children born to one woman',
);
//add your loop below this line
for ($i = 0; $i < count($facts); $i++) {
[$key, $fact] = each($facts);
echo $key . $fact . "\n";
}
Will print out this:
57 on Heinz ketchup bottles represents the number of varieties of pickles the company once had.
2 is the approximate hours a day Giraffes sleeps
18 is the average hours a Python sleeps per day
10 per cent of the world is left-handed.
11 Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.
98% of the atoms in your body are replaced every year
69 is the largest number of recorded children born to one woman
But as I said I haven't done the course so I might be missing something here.
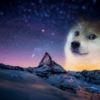
Connor Walker
17,985 PointsThat code wouldn't work for me when I have tried it, although this code you showed did work fine when i tried it in Workspaces (although a foreach loop would probably just be simpler).
for ($i = 0; $i < count($facts); $i++) {
$item = next($facts);
echo key($facts) . $item . "<br>";
}
(because the indexes have been set separately).
57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.',
The "57 =>" part of this line is saying to place that string in index 57 of the $facts array instead of index 0 like it would be without this small bit code. Because of this, even though the array contains 7 items the highest index that is being used is not 6, it is 98. This is why you can't use the array length as the counter limit.

Tobias Karlsson
2,751 PointsActually I do not think you fully understand what is happening here. The index in my examples is irrelevant. The index can contain strings or what ever.
The count() function determines how many elements are in the array.
The each() and the next() functions moves the internal counter of the array in each iteration of the for-loop.
If this example doesn't work for you, it is probably because of your PHP version. This is called Symmetric array destructuring and is a fairly new feature.
<?php
for ($i = 0; $i < count($facts); $i++) {
[$key, $fact] = each($facts);
echo $key . $fact . "\n";
}
Then you can switch it to a list() each():
<?php
for ($i = 0; $i < count($facts); $i++) {
list($key, $fact) = each($facts);
echo $key . $fact . "\n";
}
edit:
although a foreach loop would probably just be simpler
Of course it would, but the task in the course was to use the for-loop.

Tobias Karlsson
2,751 PointsOk, now I have read the question and it should loop 100 times and print the numbers.
Use the function isset to test if the incremented value equals one of the keys in the $facts array. If there is a key that matches, display the value AFTER the number. NOTE: all numbers between 1 and 100 should still be displayed
Sorry :)
Mahfuzur Rahman
3,204 PointsMahfuzur Rahman
3,204 Pointshmm, I was surely over complicating the stuff. And I surely underestimated the power of isset() function. Thanks a lot. :) But how is the 'echo $facts[$i]' getting the values from array. In my understanding it's supposed to get only the keys.