Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial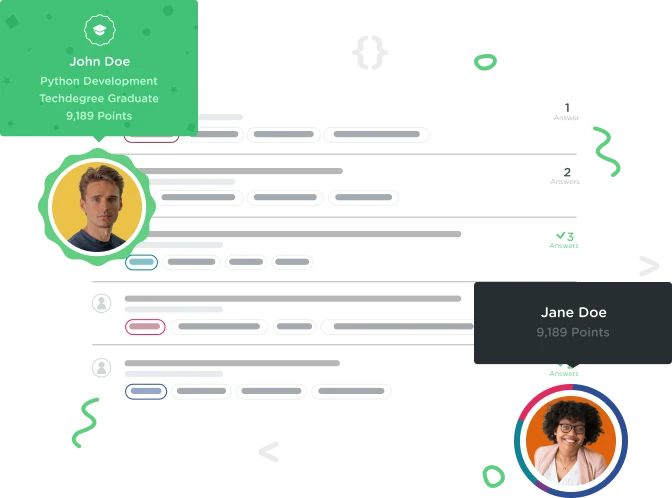
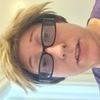
Katriel Paige
9,260 PointsNot finding correct index?
I think I have the method right even accounting for relocating the if statements, but I may have messed up - it's still not returning the correct/expected index. Did I miss a key word somewhere?
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def find_index(name)
index = 0
found = false
todo_items.index do |todo_item|
if todo_item.name == name
found = true
break
else index += 1
end
if found
return index
else
return nil
end
end
end
end
1 Answer

Ken jones
5,394 PointsThere are a few little problems with this class.
As it stands this function will never actually return the index because the logic is broken, it won't even make it through one iteration of your loop.
There are two paths that will get travelled here, lets say you pass the name of the first todo in your array as an argument.
todo_item.name == name
This will return true. Great! Thats what we want, However, the next two lines is where things go wrong..
found = true
break
If you break at this point ruby will exit the innermost loop. Thats your todo_items loop. This stops the function going any further so it won't make it to the part where you check if found == true !
The second path is if you pass an argument that isn't the name of the first item in the array. Then you will make it to this section...
if found
return index
else
return nil
end
In ruby the return statement will exit the function entirely. As you will only ever reach this part with a todo_item.name that isn't equal to name its going to exit the function and return nil.
So basically your loop will only make it through one iteration then it exits. Thats why you aren't getting the index returned.
the following should fix it up, I've refactored it slightly because refactoring is fun!
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def find_index(name)
todo_items.each_with_index do |todo_item, index|
if todo_item.name == name
return index
end
end
end
end
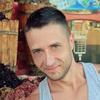
Vladislav Trotsenko
9,291 PointsNice, but your code is too big. No need to use .each_with_index method in this case ;)
def find_index(name)
@todo_items.find_index { |item| item.name == name }
end
David Long
8,264 PointsDavid Long
8,264 PointsHi Katriel,
Is there more to your code? I think the issue lies with the add_items method. It does not seem to know what "TodoItem" is.